使用Java提供示例代码
时间: 2023-08-18 20:10:45 浏览: 45
以下是使用Java实现可重入锁和公平锁的示例代码:
可重入锁:
```java
import redis.clients.jedis.Jedis;
public class RedisReentrantLock {
private ThreadLocal<Integer> count = new ThreadLocal<>();
private ThreadLocal<Long> owner = new ThreadLocal<>();
private Jedis jedis;
private String lockKey;
public RedisReentrantLock(Jedis jedis, String lockKey) {
this.jedis = jedis;
this.lockKey = lockKey;
}
public boolean acquire() {
long threadId = Thread.currentThread().getId();
Integer lockCount = count.get();
if (lockCount != null && owner.get() == threadId) {
count.set(lockCount + 1);
return true;
}
boolean acquired = "OK".equals(jedis.set(lockKey, Long.toString(threadId), "NX", "EX", 30));
if (acquired) {
owner.set(threadId);
count.set(1);
}
return acquired;
}
public boolean release() {
long threadId = Thread.currentThread().getId();
if (owner.get() != threadId) {
throw new IllegalMonitorStateException("Not holding the lock.");
}
int lockCount = count.get() - 1;
count.set(lockCount);
if (lockCount == 0) {
jedis.del(lockKey);
owner.remove();
}
return true;
}
}
```
公平锁:
```java
import redis.clients.jedis.Jedis;
public class RedisFairLock {
private ThreadLocal<Long> owner = new ThreadLocal<>();
private Jedis jedis;
private String lockKey;
private String countKey;
public RedisFairLock(Jedis jedis, String lockKey) {
this.jedis = jedis;
this.lockKey = lockKey;
this.countKey = lockKey + ":count";
}
public boolean acquire() {
long threadId = Thread.currentThread().getId();
boolean acquired = false;
while (!acquired) {
jedis.rpush(lockKey, Long.toString(threadId));
while (!jedis.lindex(lockKey, 0).equals(Long.toString(threadId))) {
try {
Thread.sleep(1);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
long count = jedis.incr(countKey);
if (count == 1) {
jedis.expire(countKey, 30);
acquired = true;
}
}
owner.set(threadId);
return true;
}
public boolean release() {
long threadId = Thread.currentThread().getId();
if (owner.get() != threadId) {
throw new IllegalMonitorStateException("Not holding the lock.");
}
jedis.lpop(lockKey);
long count = jedis.decr(countKey);
if (count == 0) {
jedis.del(countKey);
}
owner.remove();
return true;
}
}
```
以上代码仅供参考,实际使用时需要根据具体情况进行修改和优化。需要注意的是,使用Redis实现分布式锁时,要确保操作的原子性和可靠性,避免死锁和误解锁等问题。
相关推荐
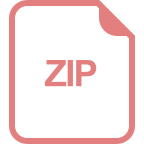















