利用Java语言实现一个DES、AES对文件的加解密;把原始信息存在文件a.txt中,密文存在b.txt中,解密信息存在c.txt中
时间: 2024-05-13 14:17:34 浏览: 127
以下是Java语言实现DES、AES对文件的加解密的示例代码。在代码中,我们使用了Java标准库中的Cipher类来实现DES、AES算法的加解密,FileInputStream和FileOutputStream类来读写文件。
注:由于DES算法已经不安全,本示例代码中仅供学习和参考,不建议在实际应用中使用。
```java
import javax.crypto.*;
import javax.crypto.spec.*;
import java.io.*;
public class FileEncryptor {
// DES加解密密钥
private static final byte[] DES_KEY = {0x11, 0x22, 0x4F, 0x58, (byte)0x88, 0x10, 0x40, 0x38};
// AES加解密密钥
private static final byte[] AES_KEY = {0x2b, 0x7e, 0x15, 0x16, 0x28, (byte)0xae, (byte)0xd2, (byte)0xa6,
(byte)0xab, 0xf7, 0x15, (byte)0x88, 0x09, (byte)0xcf, 0x4f, 0x3c};
// DES加密文件
public static void desEncryptFile(String srcFilePath, String destFilePath) throws Exception {
Cipher cipher = Cipher.getInstance("DES/ECB/PKCS5Padding");
SecretKeySpec keySpec = new SecretKeySpec(DES_KEY, "DES");
cipher.init(Cipher.ENCRYPT_MODE, keySpec);
FileInputStream fis = new FileInputStream(srcFilePath);
FileOutputStream fos = new FileOutputStream(destFilePath);
CipherOutputStream cos = new CipherOutputStream(fos, cipher);
byte[] buffer = new byte[1024];
int len;
while ((len = fis.read(buffer)) != -1) {
cos.write(buffer, 0, len);
}
cos.close();
fos.close();
fis.close();
}
// DES解密文件
public static void desDecryptFile(String srcFilePath, String destFilePath) throws Exception {
Cipher cipher = Cipher.getInstance("DES/ECB/PKCS5Padding");
SecretKeySpec keySpec = new SecretKeySpec(DES_KEY, "DES");
cipher.init(Cipher.DECRYPT_MODE, keySpec);
FileInputStream fis = new FileInputStream(srcFilePath);
CipherInputStream cis = new CipherInputStream(fis, cipher);
FileOutputStream fos = new FileOutputStream(destFilePath);
byte[] buffer = new byte[1024];
int len;
while ((len = cis.read(buffer)) != -1) {
fos.write(buffer, 0, len);
}
fos.close();
cis.close();
fis.close();
}
// AES加密文件
public static void aesEncryptFile(String srcFilePath, String destFilePath) throws Exception {
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
SecretKeySpec keySpec = new SecretKeySpec(AES_KEY, "AES");
IvParameterSpec ivSpec = new IvParameterSpec(AES_KEY, 0, 16);
cipher.init(Cipher.ENCRYPT_MODE, keySpec, ivSpec);
FileInputStream fis = new FileInputStream(srcFilePath);
FileOutputStream fos = new FileOutputStream(destFilePath);
CipherOutputStream cos = new CipherOutputStream(fos, cipher);
byte[] buffer = new byte[1024];
int len;
while ((len = fis.read(buffer)) != -1) {
cos.write(buffer, 0, len);
}
cos.close();
fos.close();
fis.close();
}
// AES解密文件
public static void aesDecryptFile(String srcFilePath, String destFilePath) throws Exception {
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
SecretKeySpec keySpec = new SecretKeySpec(AES_KEY, "AES");
IvParameterSpec ivSpec = new IvParameterSpec(AES_KEY, 0, 16);
cipher.init(Cipher.DECRYPT_MODE, keySpec, ivSpec);
FileInputStream fis = new FileInputStream(srcFilePath);
CipherInputStream cis = new CipherInputStream(fis, cipher);
FileOutputStream fos = new FileOutputStream(destFilePath);
byte[] buffer = new byte[1024];
int len;
while ((len = cis.read(buffer)) != -1) {
fos.write(buffer, 0, len);
}
fos.close();
cis.close();
fis.close();
}
public static void main(String[] args) throws Exception {
// DES加密文件
desEncryptFile("a.txt", "b_des.txt");
// DES解密文件
desDecryptFile("b_des.txt", "c_des.txt");
// AES加密文件
aesEncryptFile("a.txt", "b_aes.txt");
// AES解密文件
aesDecryptFile("b_aes.txt", "c_aes.txt");
}
}
```
在代码中,我们定义了两个密钥:DES_KEY和AES_KEY,分别用于DES和AES算法的加解密。我们使用了Java标准库中的Cipher类来实现加解密操作,其中getInstance方法的参数指定了加密算法、工作模式和填充方式。我们使用CipherOutputStream和CipherInputStream类来实现文件的加解密,这两个类可以将加密和解密操作与文件读写操作结合起来。
在main方法中,我们分别对a.txt文件进行了DES和AES加解密操作,加密后的文件分别保存在b_des.txt和b_aes.txt中,解密后的文件分别保存在c_des.txt和c_aes.txt中。
阅读全文
相关推荐
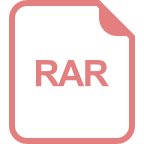
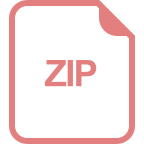
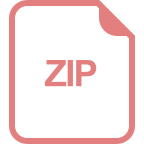
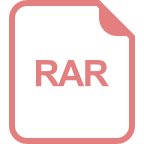
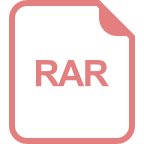
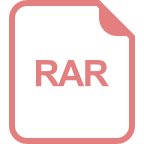
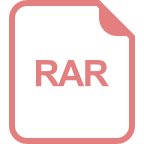
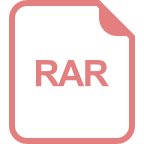
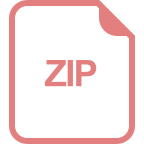
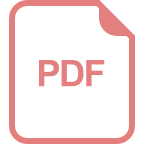
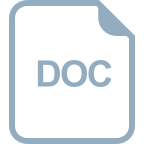
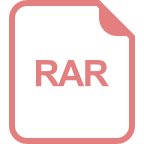
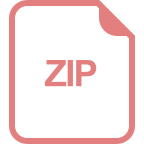
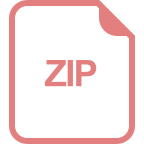
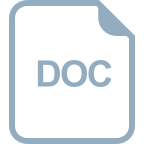
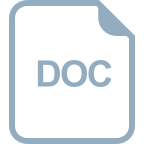
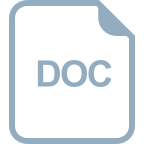
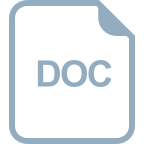