在前端页面中使用JavaScript通过AJAX技术向后端发送查询请求,并接收后端返回的JSON格式的天气信息,使用JavaScript动态更新前端页面展示的天气信息。怎么写代码
时间: 2024-02-11 21:04:37 浏览: 74
以下是一个简单的示例代码,实现了通过JavaScript和AJAX向后端发送查询请求,并将返回的JSON格式的天气信息更新到前端页面上:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>天气查询</title>
</head>
<body>
<h1>输入城市名称查询天气</h1>
<input type="text" id="city">
<button onclick="queryWeather()">查询</button>
<h2>查询结果</h2>
<div id="result"></div>
<script>
function queryWeather() {
var city = document.getElementById('city').value;
var xhr = new XMLHttpRequest();
xhr.open('GET', '/query_weather?city=' + city, true);
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
var weather = JSON.parse(xhr.responseText);
var resultDiv = document.getElementById('result');
resultDiv.innerHTML = '城市:' + weather.city + '<br>温度:' + weather.temperature + '℃<br>湿度:' + weather.humidity + '%<br>风力:' + weather.wind;
}
};
xhr.send();
}
</script>
</body>
</html>
```
在以上代码中,我们使用了XMLHttpRequest对象发起了一个GET请求,查询参数为city,请求的URL为/query_weather。当请求成功返回后,我们将返回的JSON字符串解析为JavaScript对象,然后将天气信息更新到页面上。
在后端程序中,我们需要实现一个接口/query_weather来处理前端发送过来的请求,查询相应的天气信息并将其以JSON格式返回给前端。以下是一个简单的Python代码示例:
```python
from flask import Flask, request, jsonify
app = Flask(__name__)
# 假设天气数据存储在一个字典中,键为城市名称,值为一个字典,包括温度、湿度和风力等信息
weather_data = {
'北京': {'temperature': 23, 'humidity': 50, 'wind': '北风4级'},
'上海': {'temperature': 26, 'humidity': 60, 'wind': '东南风3级'},
'广州': {'temperature': 30, 'humidity': 80, 'wind': '南风2级'}
}
@app.route('/query_weather', methods=['GET'])
def query_weather():
city = request.args.get('city')
weather = weather_data.get(city)
if weather:
return jsonify({'city': city, 'temperature': weather['temperature'], 'humidity': weather['humidity'], 'wind': weather['wind']})
else:
return jsonify({'error': '城市不存在'})
if __name__ == '__main__':
app.run()
```
在以上代码中,我们使用了Flask框架实现了一个/query_weather的接口,通过request.args.get('city')获取前端传来的查询参数city,然后查询相应的天气数据并返回给前端。由于我们使用了jsonify方法,返回的数据会自动转换为JSON格式。
阅读全文
相关推荐



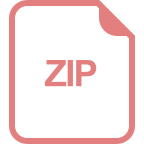












