model = Sequential() #model.add(Embedding(500, embed_dim,input_length = test_X.shape[0])) model.add(LSTM(128, input_shape=(train_X.shape[1], train_X.shape[2]))) model.add(Dropout(0.3)) model.add(Dense(14)) model.add(LSTM(64, input_shape=(train_X.shape[1], train_X.shape[2]))) model.add(Dropout(0.3)) model.add(Dense(1)) model.compile(loss='mae', optimizer='adam')
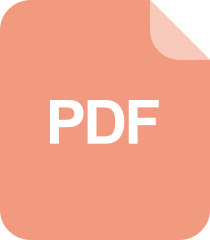
浅谈keras通过model.fit_generator训练模型(节省内存)
### Keras中使用`model.fit_generator`训练模型详解 #### 前言 在机器学习领域,特别是深度学习中,模型训练过程中经常会遇到的一个问题是内存溢出。这主要是因为随着数据集规模的增长以及输入特征维度的增加,所需内存也会急剧上升。例如,在图像识别任务中,如果每个图像大小为224x224像素,颜色通道为3,且数据集包含20000张图像,那么全部加载到内存中将会占用大约11.2GB的空间。对于一般的计算机硬件而言,这是一个非常大的数字。 Keras框架提供了一个解决方案——`model.fit_generator`方法,它允许用户通过分批读取数据的方式来训练模型,从而有效降低内存需求。本文将详细介绍如何使用`model.fit_generator`以及其实现细节。 #### 1. `fit_generator`函数简介 `fit_generator`函数的主要目的是为了能够在训练过程中动态生成数据,而不是一次性将所有数据加载到内存中。这对于处理大型数据集非常有用。该函数的基本结构如下: ```python fit_generator( generator, steps_per_epoch=None, epochs=1, verbose=1, callbacks=None, validation_data=None, validation_steps=None, class_weight=None, max_queue_size=10, workers=1, use_multiprocessing=False, shuffle=True, initial_epoch=0 ) ``` - **generator**:这是整个函数的核心。它可以是一个生成器或`keras.utils.Sequence`对象的实例。生成器需要按照特定的方式返回数据。 - **steps_per_epoch**:表示在一个完整的训练周期(epoch)内,需要调用生成器多少次。通常情况下,它的值设置为数据集中样本数除以批量大小(batch size)。 - **epochs**:模型需要训练的总轮数。 - **verbose**:日志输出模式,可以选择0、1或2,分别代表不输出、输出进度条、每轮输出一行日志。 - **callbacks**:在训练过程中可以调用的一系列回调函数,用于监控训练过程。 - **validation_data**:用于验证的数据生成器,与训练数据生成器类似。 - **validation_steps**:在一个完整的验证周期内需要调用验证数据生成器的次数。 - **class_weight**:一个可选参数,用于指定不同类别的重要性。可以通过一个字典将类别的索引映射到权重值,以便模型更加重视那些样本较少的类别。 - **max_queue_size**:生成器队列的最大容量,默认为10。 - **workers**:用于生成数据的进程数。如果设置为0,则在主进程中执行。 - **use_multiprocessing**:布尔值,决定是否启用基于进程的多线程。 - **shuffle**:布尔值,只有在使用`keras.utils.Sequence`时可用,用于确定是否在每个epoch开始前随机打乱数据顺序。 - **initial_epoch**:指定开始训练的epoch数,通常用于继续先前中断的训练。 #### 2. Generator实现 ##### 2.1 生成器的实现方式 生成器是一种特殊的Python函数,它可以使用`yield`关键字返回一系列结果,而不仅仅是单一的结果。下面是一个简单的生成器示例: ```python import keras from keras.models import Sequential from keras.layers import Dense import numpy as np from sklearn.model_selection import train_test_split from PIL import Image def process_x(path): img = Image.open(path) img = img.resize((96, 96)) img = img.convert('RGB') img = np.array(img) img = np.asarray(img, np.float32) / 255.0 # 进行一些数据增强操作 return img def generate_arrays_from_file(x_y): global count batch_size = 32 # 指定批量大小 while True: x_batch = [] y_batch = [] for i in range(batch_size): # 从x_y中随机选择一个样本 sample = np.random.choice(x_y) x = process_x(sample[0]) y = sample[1] x_batch.append(x) y_batch.append(y) yield (np.array(x_batch), np.array(y_batch)) count += 1 ``` 在这个例子中,`generate_arrays_from_file`是一个典型的生成器,它不断地从数据集中抽取样本,并对图像进行预处理。然后它返回一个批量的图像及其对应的标签。需要注意的是,这里使用了一个无限循环`while True`,这是因为`fit_generator`函数会在每个epoch结束时自动调用生成器来获取新的数据批次。 总结来说,`model.fit_generator`是一种非常有效的训练大型数据集的方法,它能够显著减少内存使用量,并且通过自定义生成器可以轻松实现数据增强等功能。理解并熟练掌握这一技巧对于高效地进行深度学习模型训练至关重要。
相关推荐
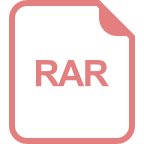
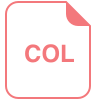
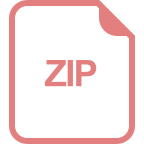
最新推荐
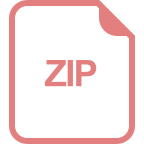
基于freeRTOS和STM32F103x的手机远程控制浴室温度系统设计源码
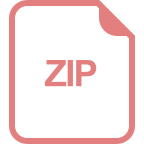
Windows平台下的Fastboot工具使用指南

管理建模和仿真的文件
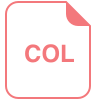
DLMS规约深度剖析:从基础到电力通信标准的全面掌握

修改代码,使其正确运行
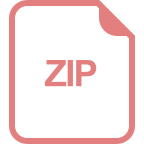
Python机器学习基础入门与项目实践

"互动学习:行动中的多样性与论文攻读经历"
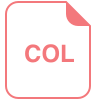
【Shell脚本进阶】:wc命令行数统计的高级用法及解决方案

python编写一个程序,使得根据输入的起点和终点坐标值计算出坐标方位角
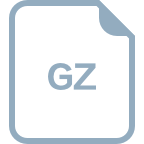