安卓开发调用系统相机拍照,并且对照片编辑添加文字,然后照片储存到Room数据库之中,并获取相册路径,在recyclerview中显示出来
时间: 2023-10-02 09:07:35 浏览: 221
实现这个功能需要以下几个步骤:
1. 添加相机权限和读写外部存储权限到AndroidManifest.xml文件中
```
<uses-permission android:name="android.permission.CAMERA"/>
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
```
2. 在布局文件中添加一个ImageView用于显示照片,一个EditText用于输入文字,一个Button用于拍照和保存照片
```
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent" android:layout_height="match_parent">
<ImageView
android:id="@+id/imageView"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scaleType="centerCrop"/>
<EditText
android:id="@+id/editText"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:padding="16dp"/>
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Take Photo"
android:layout_above="@id/editText"
android:layout_centerHorizontal="true"/>
</RelativeLayout>
```
3. 在Activity中实现拍照逻辑:
首先,定义一个变量用于存储照片路径,然后在onCreate方法中初始化相机和图片存储路径:
```
private String photoPath;
private static final int REQUEST_IMAGE_CAPTURE = 1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Initialize camera and photo path
File photoFile = null;
try {
photoFile = createImageFile();
} catch (IOException ex) {
// Error occurred while creating the File
ex.printStackTrace();
}
if (photoFile != null) {
photoPath = photoFile.getAbsolutePath();
Uri photoURI = FileProvider.getUriForFile(this,
"com.example.android.fileprovider",
photoFile);
Intent takePictureIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
takePictureIntent.putExtra(MediaStore.EXTRA_OUTPUT, photoURI);
if (takePictureIntent.resolveActivity(getPackageManager()) != null) {
startActivityForResult(takePictureIntent, REQUEST_IMAGE_CAPTURE);
}
}
}
// Create a unique file name for the photo
private File createImageFile() throws IOException {
// Create an image file name
String timeStamp = new SimpleDateFormat("yyyyMMdd_HHmmss").format(new Date());
String imageFileName = "JPEG_" + timeStamp + "_";
File storageDir = getExternalFilesDir(Environment.DIRECTORY_PICTURES);
File image = File.createTempFile(
imageFileName, /* prefix */
".jpg", /* suffix */
storageDir /* directory */
);
// Save a file: path for use with ACTION_VIEW intents
return image;
}
```
接着,在onActivityResult方法中获取拍摄的照片并显示在ImageView中:
```
@Override
protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == REQUEST_IMAGE_CAPTURE && resultCode == RESULT_OK) {
Bitmap bitmap = BitmapFactory.decodeFile(photoPath);
ImageView imageView = findViewById(R.id.imageView);
imageView.setImageBitmap(bitmap);
}
}
```
最后,在Button的点击事件中获取EditText的文本内容并添加到图片上,然后保存到Room数据库中并更新RecyclerView:
```
private AppDatabase db;
private List<Photo> photoList;
private PhotoAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Initialize database and RecyclerView
db = Room.databaseBuilder(getApplicationContext(),
AppDatabase.class, "photo-database").build();
photoList = new ArrayList<>();
adapter = new PhotoAdapter(photoList);
RecyclerView recyclerView = findViewById(R.id.recyclerView);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
recyclerView.setAdapter(adapter);
// Set button click listener
Button button = findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
EditText editText = findViewById(R.id.editText);
String text = editText.getText().toString();
Bitmap bitmap = BitmapFactory.decodeFile(photoPath);
Bitmap newBitmap = addTextToBitmap(bitmap, text);
saveToDatabase(newBitmap);
updateRecyclerView();
editText.setText("");
}
});
}
// Add text to the bottom of the bitmap
private Bitmap addTextToBitmap(Bitmap bitmap, String text) {
Bitmap newBitmap = bitmap.copy(Bitmap.Config.ARGB_8888, true);
Canvas canvas = new Canvas(newBitmap);
Paint paint = new Paint(Paint.ANTI_ALIAS_FLAG);
paint.setColor(Color.WHITE);
paint.setTextSize(48);
paint.setTextAlign(Paint.Align.CENTER);
Rect bounds = new Rect();
paint.getTextBounds(text, 0, text.length(), bounds);
int x = canvas.getWidth() / 2;
int y = canvas.getHeight() - bounds.height() - 64;
canvas.drawText(text, x, y, paint);
return newBitmap;
}
// Save the photo to database
private void saveToDatabase(Bitmap bitmap) {
ByteArrayOutputStream stream = new ByteArrayOutputStream();
bitmap.compress(Bitmap.CompressFormat.JPEG, 100, stream);
byte[] bytes = stream.toByteArray();
Photo photo = new Photo(bytes);
db.photoDao().insert(photo);
}
// Update the RecyclerView with new data from database
private void updateRecyclerView() {
photoList.clear();
photoList.addAll(db.photoDao().getAll());
adapter.notifyDataSetChanged();
}
```
完整的代码可以在GitHub上查看:https://github.com/guolindev/Android-Camera-Room。
阅读全文
相关推荐
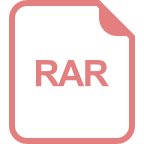
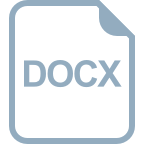
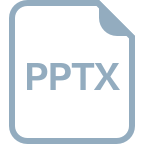
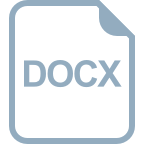
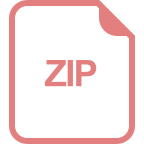
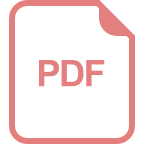
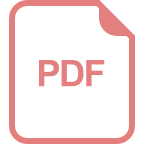
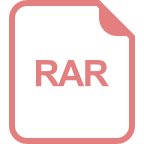
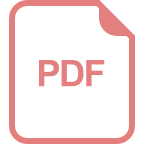
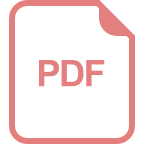
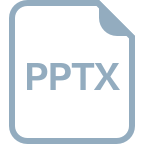