用c++设计长方体求出长方体的表面积和体积并判断两个长方体是否相等
时间: 2024-02-20 12:02:39 浏览: 176
好的,我可以回答这个问题。
首先,我们需要使用C++语言编写一个长方体类。长方体类应该具有长度(length)、宽度(width)和高度(height)这三个成员变量,以及计算表面积和体积的方法。
下面是一个简单的长方体类示例:
```c++
class Cuboid {
public:
// 构造函数
Cuboid(double l, double w, double h) : length(l), width(w), height(h) {}
// 计算表面积
double surface_area() const {
return 2 * (length * width + length * height + width * height);
}
// 计算体积
double volume() const {
return length * width * height;
}
// 判断两个长方体是否相等
bool operator==(const Cuboid& other) const {
return length == other.length && width == other.width && height == other.height;
}
private:
double length;
double width;
double height;
};
```
在这个示例中,我们定义了一个Cuboid类,并在构造函数中初始化了长度、宽度和高度。然后,我们定义了计算表面积和体积的两个方法,并使用运算符重载来判断两个长方体是否相等。
现在,我们可以使用这个类来计算两个长方体的表面积和体积,并判断它们是否相等:
```c++
Cuboid cuboid1(2.0, 3.0, 4.0);
Cuboid cuboid2(3.0, 4.0, 2.0);
// 计算表面积和体积
double surface_area1 = cuboid1.surface_area();
double volume1 = cuboid1.volume();
// 输出结果
std::cout << "Cuboid 1 surface area: " << surface_area1 << std::endl;
std::cout << "Cuboid 1 volume: " << volume1 << std::endl;
// 计算表面积和体积
double surface_area2 = cuboid2.surface_area();
double volume2 = cuboid2.volume();
// 输出结果
std::cout << "Cuboid 2 surface area: " << surface_area2 << std::endl;
std::cout << "Cuboid 2 volume: " << volume2 << std::endl;
// 判断两个长方体是否相等
if (cuboid1 == cuboid2) {
std::cout << "The two cuboids are equal." << std::endl;
} else {
std::cout << "The two cuboids are not equal." << std::endl;
}
```
这段代码将输出两个长方体的表面积和体积,并判断它们是否相等。
阅读全文
相关推荐
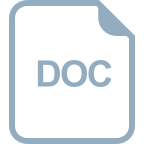
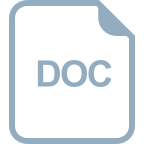
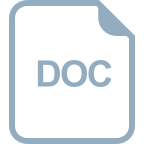





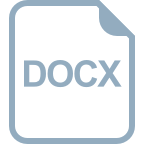
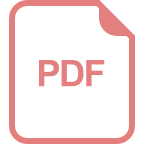
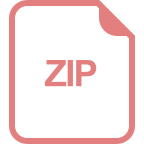
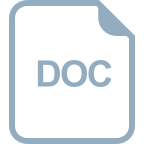
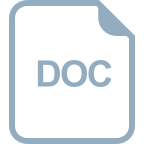
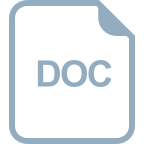
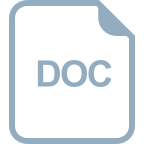