jemeter 将json数据写入到csv文件
时间: 2023-09-30 21:09:08 浏览: 246
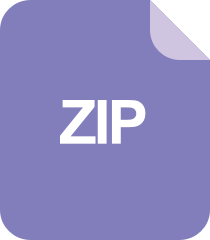
jsonltocsv:将JSON行流式传输到csv文件中
可以使用Python内置的csv和json库来实现将json数据写入到csv文件的操作。具体步骤如下:
1. 导入csv和json库
```python
import csv
import json
```
2. 打开json文件并加载数据
```python
with open('data.json', 'r') as f:
data = json.load(f)
```
3. 打开csv文件并创建写入器
```python
with open('data.csv', 'w', newline='') as f:
writer = csv.writer(f)
```
4. 写入表头
```python
header = ['name', 'age', 'gender']
writer.writerow(header)
```
5. 遍历json数据,并将每个字典中的值写入csv文件
```python
for item in data:
row = [item['name'], item['age'], item['gender']]
writer.writerow(row)
```
完整代码如下:
```python
import csv
import json
with open('data.json', 'r') as f:
data = json.load(f)
with open('data.csv', 'w', newline='') as f:
writer = csv.writer(f)
header = ['name', 'age', 'gender']
writer.writerow(header)
for item in data:
row = [item['name'], item['age'], item['gender']]
writer.writerow(row)
```
注意,这里将json文件中的数据按照一定的格式写入到csv文件中,如果json文件的数据格式与写入csv文件的格式不一致,可能会导致写入失败或数据丢失。因此,在实际应用中需要根据具体情况进行调整。
阅读全文
相关推荐
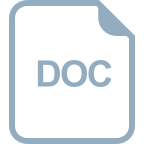
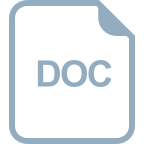

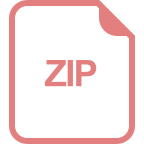
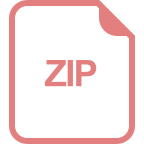
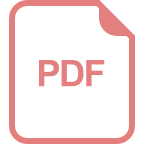
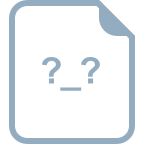
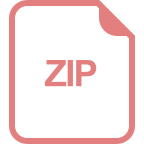

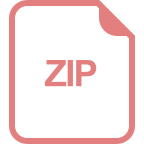
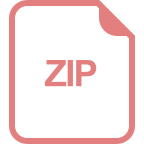
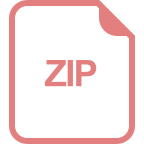
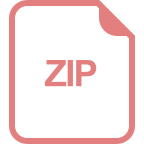
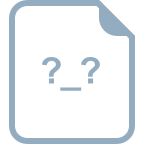
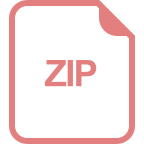
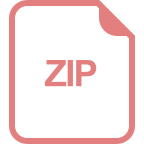
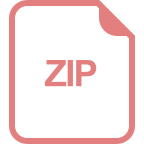
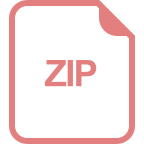