用Objective-c语言纯代码写一个签到的日期界面
时间: 2024-03-03 20:50:48 浏览: 166
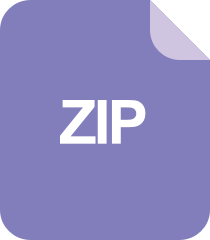
Objective-C语言教程与案例.zip
好的,以下是用Objective-C纯代码实现的签到日期界面。实现的界面包括一个导航栏、一个显示当前日期的标签和一个签到按钮。
ViewController.h文件:
```objective-c
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController
@end
```
ViewController.m文件:
```objective-c
#import "ViewController.h"
@interface ViewController ()
@property (nonatomic, strong) UILabel *dateLabel;
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
// Do any additional setup after loading the view.
// 设置导航栏标题
self.navigationItem.title = @"签到";
// 设置背景颜色
self.view.backgroundColor = [UIColor whiteColor];
// 创建并添加日期标签
self.dateLabel = [[UILabel alloc] initWithFrame:CGRectMake(0, 0, 200, 50)];
self.dateLabel.center = CGPointMake(self.view.frame.size.width/2, self.view.frame.size.height/2 - 50);
self.dateLabel.textAlignment = NSTextAlignmentCenter;
self.dateLabel.textColor = [UIColor blackColor];
[self.view addSubview:self.dateLabel];
// 更新日期标签的文本
[self updateDateLabel];
// 创建并添加签到按钮
UIButton *checkInButton = [UIButton buttonWithType:UIButtonTypeSystem];
checkInButton.frame = CGRectMake(0, 0, 200, 50);
checkInButton.center = CGPointMake(self.view.frame.size.width/2, self.view.frame.size.height/2 + 50);
[checkInButton setTitle:@"签到" forState:UIControlStateNormal];
[checkInButton addTarget:self action:@selector(checkInButtonClicked:) forControlEvents:UIControlEventTouchUpInside];
[self.view addSubview:checkInButton];
}
// 更新日期标签的文本
- (void)updateDateLabel {
NSDateFormatter *formatter = [[NSDateFormatter alloc] init];
formatter.dateFormat = @"yyyy年MM月dd日";
self.dateLabel.text = [formatter stringFromDate:[NSDate date]];
}
// 签到按钮点击事件
- (void)checkInButtonClicked:(UIButton *)sender {
// TODO: 处理签到逻辑
// 更新日期标签的文本
[self updateDateLabel];
}
@end
```
在AppDelegate.m文件中,将ViewController设置为根视图控制器:
```objective-c
#import "AppDelegate.h"
#import "ViewController.h"
@interface AppDelegate ()
@end
@implementation AppDelegate
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
// Override point for customization after application launch.
self.window = [[UIWindow alloc] initWithFrame:[UIScreen mainScreen].bounds];
ViewController *viewController = [[ViewController alloc] init];
UINavigationController *navigationController = [[UINavigationController alloc] initWithRootViewController:viewController];
self.window.rootViewController = navigationController;
[self.window makeKeyAndVisible];
return YES;
}
```
这样就完成了一个简单的签到日期界面的实现。运行程序后,可以看到一个带有日期标签和签到按钮的界面。点击签到按钮后,日期标签上的日期会更新为当前日期。
阅读全文
相关推荐
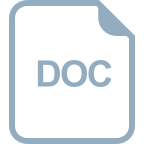
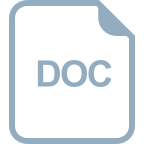

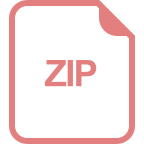
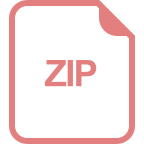
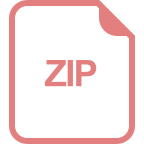
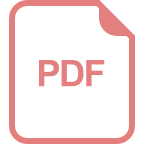
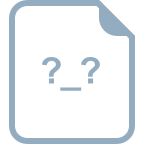
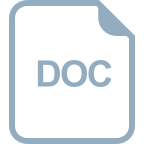
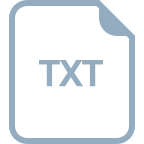
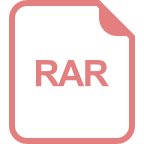
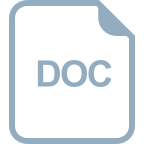
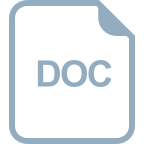
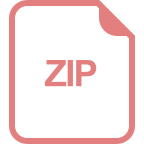
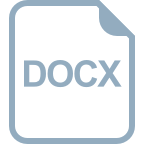
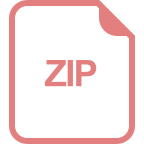
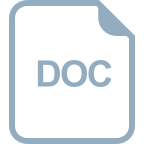