Java语言写一个读取本地excel表中的两个列作为map的key和value并且作为已经写好接口的参数调用接口
时间: 2024-05-01 18:17:07 浏览: 150
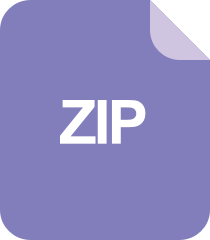
java对Excel进行读取、写入
以下是一个可能的Java代码示例,其中Apache POI库用于读取Excel文件,通过HttpClient库调用API接口:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.mime.MultipartEntityBuilder;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelToApiCaller {
public static void main(String[] args) {
try {
// 读取Excel文件
FileInputStream file = new FileInputStream(new File("path/to/excel/file.xlsx"));
Workbook workbook = new XSSFWorkbook(file);
Sheet sheet = workbook.getSheetAt(0); // 假设第一个sheet是我们需要的
// 将两列数据存入Map
Map<String, String> dataMap = new HashMap<String, String>();
for (Row row : sheet) {
Cell keyCell = row.getCell(0); // 假设第一列是key
Cell valueCell = row.getCell(1); // 假设第二列是value
if (keyCell != null && valueCell != null) {
String key = keyCell.getStringCellValue();
String value = valueCell.getStringCellValue();
dataMap.put(key, value);
}
}
// 调用API接口
HttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost("http://api.example.com/path/to/api");
MultipartEntityBuilder builder = MultipartEntityBuilder.create();
for (Map.Entry<String, String> entry : dataMap.entrySet()) {
builder.addTextBody("key", entry.getKey());
builder.addTextBody("value", entry.getValue());
}
HttpEntity multipart = builder.build();
httpPost.setEntity(multipart);
HttpResponse response = httpClient.execute(httpPost);
HttpEntity responseEntity = response.getEntity();
String responseString = EntityUtils.toString(responseEntity, "UTF-8");
System.out.println(responseString);
// 关闭资源
file.close();
workbook.close();
httpClient.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
请注意,此代码示例仅供参考,具体实现可能需要根据实际情况进行调整和优化。
阅读全文
相关推荐

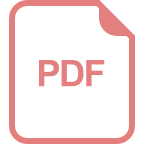
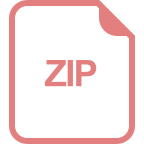
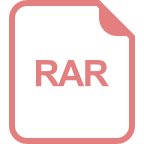
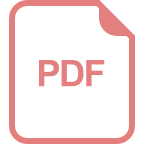
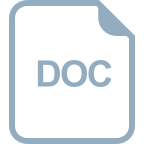
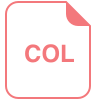
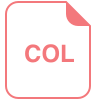
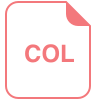






