如何使用pytorch将channel attention机制加入mlp中
时间: 2023-09-18 09:06:06 浏览: 359
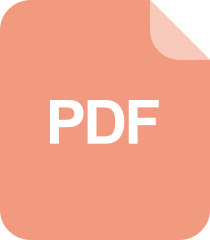
Pytorch 实现注意力机制
### 回答1:
使用pytorch实现将channel attention机制加入MLP中可以通过构建一个自定义的层并将其融入MLP结构中来实现。首先,需要构建一个自定义的channel attention层,并计算每个输入特征图的channel attention score,然后将channel attention score乘以输入特征图,最后将输出特征图拼接起来,作为MLP的输入。
### 回答2:
要将Channel Attention机制加入到MLP中,可以按照以下步骤进行实现:
1. 导入所需的库和模块,包括PyTorch、torch.nn等。
2. 定义一个MLP模型,可以使用torch.nn.Sequential()来堆叠多个全连接层。可以考虑使用ReLU作为激活函数。
3. 在每个全连接层之间添加Channel Attention机制。可以通过定义一个自定义的ChannelAttention模块来实现。在Channel Attention模块中,首先使用全局平均池化(global average pooling)将特征图维度减少为1x1,然后通过一个全连接层来计算每个通道的重要性权重。最后,通过一个Sigmoid函数来将权重限制在0到1之间,作为每个通道的注意力权重。
4. 在MLP模型的正向传播函数中,将Channel Attention模块插入到全连接层之间。在特征图传递到全连接层之前,将其输入到Channel Attention模块中进行通道注意力权重的计算,然后乘以原始特征图,以应用通道注意力机制。
5. 可以使用损失函数和优化器对模型进行训练。
一个示例的代码实现如下所示:
```python
import torch
import torch.nn as nn
class ChannelAttention(nn.Module):
def __init__(self, in_channels, reduction_ratio=16):
super(ChannelAttention, self).__init__()
self.avg_pool = nn.AdaptiveAvgPool2d(1)
self.fc = nn.Sequential(
nn.Linear(in_channels, in_channels // reduction_ratio),
nn.ReLU(inplace=True),
nn.Linear(in_channels // reduction_ratio, in_channels),
nn.Sigmoid()
)
def forward(self, x):
b, c, _, _ = x.size()
y = self.avg_pool(x).view(b, c) # 全局平均池化
y = self.fc(y).view(b, c, 1, 1) # 通道注意力权重计算
return x * y
class MLP(nn.Module):
def __init__(self, in_dim, hidden_dim, out_dim):
super(MLP, self).__init__()
self.model = nn.Sequential(
nn.Linear(in_dim, hidden_dim),
nn.ReLU(inplace=True),
ChannelAttention(hidden_dim), # 在全连接层之间添加Channel Attention层
nn.Linear(hidden_dim, out_dim)
)
def forward(self, x):
return self.model(x)
# 创建模型实例
model = MLP(in_dim=100, hidden_dim=64, out_dim=10)
# 指定损失函数和优化器
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.SGD(model.parameters(), lr=0.01)
# 使用模型进行训练和推理
...
```
在这个示例中,我们首先定义了一个ChannelAttention模块,然后将其应用到MLP模型的中间层。在MLP模型的正向传播过程中,每个全连接层之间都插入了Channel Attention层,以实现通道注意力机制的加入。然后,可以使用指定的损失函数和优化器对模型进行训练。
### 回答3:
要将通道注意力机制(channel attention)加入多层感知机(MLP)中,可以使用PyTorch的torch.nn模块来实现。
首先,需要导入所需的模块:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
```
然后,可以定义一个MLP类,并在其中添加通道注意力。MLP类可以继承自PyTorch中的nn.Module类,并在其构造函数中定义神经网络的各个层:
```python
class MLP(nn.Module):
def __init__(self, input_dim, hidden_dim, output_dim):
super(MLP, self).__init__()
self.fc1 = nn.Linear(input_dim, hidden_dim)
self.fc2 = nn.Linear(hidden_dim, output_dim)
self.channel_att = ChannelAttention(hidden_dim)
def forward(self, x):
x = self.fc1(x)
x = self.channel_att(x)
x = F.relu(x)
x = self.fc2(x)
return x
```
在MLP类中,我们添加了一个ChannelAttention类的实例,该类用于实现通道注意力机制。在MLP类的正向传播方法forward中,将输入x先通过全连接层fc1传递,然后通过通道注意力channel_att层,再经过ReLU激活函数以及最后的全连接层fc2。
接下来,需要定义通道注意力类ChannelAttention:
```python
class ChannelAttention(nn.Module):
def __init__(self, input_dim, reduction_ratio=16):
super(ChannelAttention, self).__init__()
self.avg_pool = nn.AdaptiveAvgPool1d(1)
self.fc = nn.Sequential(
nn.Linear(input_dim, input_dim // reduction_ratio),
nn.ReLU(inplace=True),
nn.Linear(input_dim // reduction_ratio, input_dim)
)
def forward(self, x):
b, c, _ = x.size()
attention = self.avg_pool(x).squeeze(-1)
attention = self.fc(attention).unsqueeze(-1).expand_as(x)
x = x * attention
return x
```
在ChannelAttention类中,我们使用了自适应平均池化层(AdaptiveAvgPool1d)来获得输入x的通道维度的平均值。然后,通过全连接层将维度减小,并经过ReLU激活函数。最后,通过另一个全连接层将维度恢复到原始输入的通道维度,并将该注意力系数应用到输入张量x上,以产生加权的输出。
通过上述步骤,我们已经成功将通道注意力机制加入MLP中。可以使用上述定义的MLP类进行训练和测试,例如:
```python
input_dim = 128
hidden_dim = 256
output_dim = 10
model = MLP(input_dim, hidden_dim, output_dim)
criterion = nn.CrossEntropyLoss()
optimizer = torch.optim.SGD(model.parameters(), lr=0.01)
# 在训练步骤中使用model进行正向传播、计算损失、反向传播和参数更新
# 在测试步骤中使用model进行正向传播并获取预测结果
```
阅读全文
相关推荐
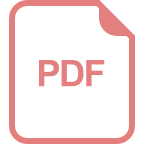
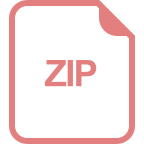
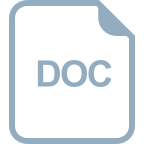
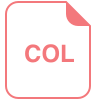
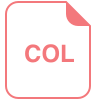
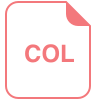
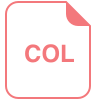


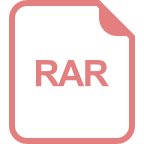
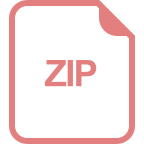
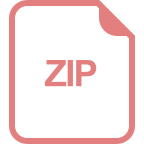
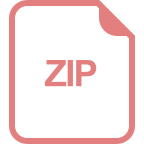
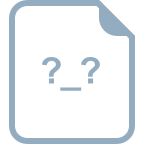