使一个文件夹里的图片以对角线为分界,使右上方的像素值为0,再使另一个文件夹里的图片以对角线为分界,使左下方的像素值为0,然后将处理后的两个文件夹的图片一一相加,给出批量处理的python代码
时间: 2024-04-29 13:25:12 浏览: 10
以下是一个处理文件夹中图片的Python代码,可以将指定文件夹中的所有图片按对角线分界,对于右上方的像素值设置为0或者对于左下方的像素值设置为0,然后将两个文件夹中处理后的图片相加。
```
import os
import cv2
import numpy as np
# 定义函数,将图片分割成左下和右上两部分,对于左下或右上的像素值设置为0
def split_image(img):
height, width, channel = img.shape
half_width = width // 2
half_height = height // 2
if channel == 1:
left_bottom = img[half_height:, :half_width]
right_top = img[:half_height, half_width:]
left_bottom[:, half_width:] = 0
right_top[:half_height, :] = 0
else:
left_bottom = img[half_height:, :half_width, :]
right_top = img[:half_height, half_width:, :]
left_bottom[:, half_width:, :] = 0
right_top[:half_height, :, :] = 0
return left_bottom, right_top
# 定义函数,将两个文件夹中的图片按照对应的顺序相加
def add_images(folder1, folder2, output_folder):
if not os.path.exists(output_folder):
os.makedirs(output_folder)
for filename in os.listdir(folder1):
if filename.endswith('.jpg') or filename.endswith('.png'):
filepath1 = os.path.join(folder1, filename)
filepath2 = os.path.join(folder2, filename)
img1 = cv2.imread(filepath1)
img2 = cv2.imread(filepath2)
left_bottom1, right_top1 = split_image(img1)
left_bottom2, right_top2 = split_image(img2)
result = np.zeros_like(img1)
result[:left_bottom1.shape[0], :left_bottom1.shape[1], :] = left_bottom1 + left_bottom2
result[result.shape[0]-right_top1.shape[0]:, result.shape[1]-right_top1.shape[1]:, :] = right_top1 + right_top2
output_file = os.path.join(output_folder, filename)
cv2.imwrite(output_file, result)
# 调用函数,将两个文件夹中的图片相加
folder1 = 'folder1'
folder2 = 'folder2'
output_folder = 'output_folder'
add_images(folder1, folder2, output_folder)
```
注意:上面的代码假设输入的图片都是RGB格式,如果是灰度图,需要将代码中的channel改为1。另外,代码中使用了OpenCV库进行图片的读取和处理,需要安装该库。
相关推荐
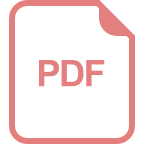
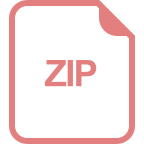














