java将txt文件导入到mysql
时间: 2023-05-31 08:19:31 浏览: 585
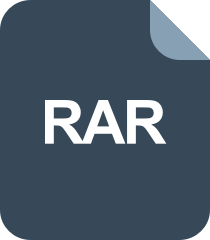
java把文本文件内容导入到数据库

### 回答1:
要将txt文件导入到MySQL中,可以使用Java编写一个程序来实现。具体步骤如下:
1. 使用Java的File类读取txt文件,将文件内容读入到程序中。
2. 使用Java的JDBC API连接到MySQL数据库。
3. 创建一个PreparedStatement对象,使用SQL语句将数据插入到MySQL中。
4. 将读取到的txt文件内容逐行插入到MySQL中。
5. 关闭PreparedStatement和数据库连接。
下面是一个简单的Java程序示例:
```java
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
public class TxtToMysql {
public static void main(String[] args) {
try {
// 读取txt文件
File file = new File("data.txt");
BufferedReader reader = new BufferedReader(new FileReader(file));
String line;
// 连接MySQL数据库
Class.forName("com.mysql.jdbc.Driver");
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/test", "root", "password");
// 插入数据
PreparedStatement ps = conn.prepareStatement("INSERT INTO data (id, name, age) VALUES (?, ?, ?)");
while ((line = reader.readLine()) != null) {
String[] data = line.split(",");
ps.setInt(1, Integer.parseInt(data[]));
ps.setString(2, data[1]);
ps.setInt(3, Integer.parseInt(data[2]));
ps.executeUpdate();
}
// 关闭连接
ps.close();
conn.close();
reader.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
在上面的示例中,我们假设txt文件中的数据格式为"id,name,age",每行一条记录。程序将数据插入到MySQL的"data"表中,表结构为"id INT PRIMARY KEY, name VARCHAR(50), age INT"。你需要根据实际情况修改程序中的数据库连接信息和SQL语句。
### 回答2:
Java将txt文件导入到MySQL需要使用Java编程语言和MySQL数据库管理系统。实现步骤如下:
1. 创建MySQL数据库和表格:首先,需要使用MySQL Workbench或者命令行创建一个数据库,然后创建一个表格,该表格包含与txt文件相同的字段。可以使用以下命令创建表格:
CREATE TABLE tbl_name (
col1 datatype,
col2 datatype,
col3 datatype,
...
);
2. 使用Java读取txt文件:需要使用Java代码读取txt文件中的数据,并将数据存储在变量中。可以使用Java的FileInputStream、InputStreamReader和BufferedReader类实现文件读取功能。
3. 将txt文件中的数据插入到MySQL数据库中:使用JDBC连接MySQL数据库,并使用INSERT INTO语句将txt文件中的数据插入到MySQL表格中,代码如下:
// 创建连接
Connection conn = DriverManager.getConnection(url, username, password);
// 创建Statement对象
Statement stmt = conn.createStatement();
// 读取txt文件中的数据,并插入到MySQL数据库中
String line = null;
while ((line = reader.readLine()) != null) {
String[] values = line.split(",");
String sql = "INSERT INTO tbl_name(col1, col2, col3, ...) VALUES ('" + values[0] + "', '" + values[1] + "', '" + values[2] + "', ...)";
stmt.executeUpdate(sql);
}
4. 关闭连接:完成数据插入后,需要关闭连接和相关对象,代码如下:
stmt.close();
conn.close();
以上就是Java将txt文件导入到MySQL的主要步骤。除了以上的实现方法,也可以使用其他框架或工具,如Spring Batch,实现相同的功能。
### 回答3:
Java将txt文件导入到MySQL可以使用JDBC(Java Database Connectivity)和SQL语句的方式实现。下面将详细介绍具体的方法:
1. 准备工作:
首先,需要确保安装了JDBC驱动程序。通常情况下,MySQL官方提供的MySQL Connector J是最常用的。如果还没有安装,请到MySQL官网下载并安装。
2. 创建表:
在MySQL中,需要先创建一张表来存储从txt文件中读取到的数据。可以使用MySQL Workbench等工具进行创建,或者使用以下SQL语句:
CREATE TABLE `table_name` (
`col1` varchar(255) NOT NULL,
`col2` varchar(255) DEFAULT NULL,
`col3` int(11) DEFAULT NULL,
PRIMARY KEY (`col1`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
该语句创建了一张名为table_name的表,包含三列col1、col2、col3。
3. 从txt文件中读取数据:
使用Java的FileReader和BufferedReader类从txt文件中逐行读取数据,并且将每一行数据分割成各个字段。可以使用split()方法进行字符串分割。
示例代码如下:
File file = new File("data.txt");
FileReader fileReader = new FileReader(file);
BufferedReader bufferedReader = new BufferedReader(fileReader);
String line;
while ((line = bufferedReader.readLine()) != null) {
String[] data = line.split(",");
String col1 = data[0];
String col2 = data[1];
int col3 = Integer.parseInt(data[2]);
// 插入数据库操作
}
bufferedReader.close();
4. 将数据插入到MySQL表中:
使用Java的JDBC连接MySQL数据库,并且使用PreparedStatement类执行SQL语句,将数据插入到MySQL表中。示例代码如下:
Class.forName("com.mysql.cj.jdbc.Driver");
Connection connection = DriverManager.getConnection(url, username, password);
String sql = "INSERT INTO table_name (col1, col2, col3) VALUES (?, ?, ?)";
PreparedStatement statement = connection.prepareStatement(sql);
statement.setString(1, col1);
statement.setString(2, col2);
statement.setInt(3, col3);
statement.executeUpdate();
statement.close();
connection.close();
5. 完整代码:
最终的Java代码如下:
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
public class ImportData {
public static void main(String[] args) {
try {
// 从txt文件中读取数据
File file = new File("data.txt");
FileReader fileReader = new FileReader(file);
BufferedReader bufferedReader = new BufferedReader(fileReader);
String line;
while ((line = bufferedReader.readLine()) != null) {
String[] data = line.split(",");
String col1 = data[0];
String col2 = data[1];
int col3 = Integer.parseInt(data[2]);
// 将数据插入到MySQL表中
Class.forName("com.mysql.cj.jdbc.Driver");
Connection connection = DriverManager.getConnection(url, username, password);
String sql = "INSERT INTO table_name (col1, col2, col3) VALUES (?, ?, ?)";
PreparedStatement statement = connection.prepareStatement(sql);
statement.setString(1, col1);
statement.setString(2, col2);
statement.setInt(3, col3);
statement.executeUpdate();
statement.close();
connection.close();
}
bufferedReader.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
其中,需要自行填写MySQL数据库的连接信息。
阅读全文
相关推荐
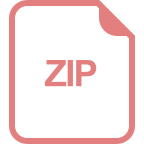
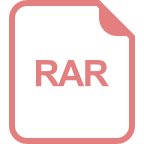
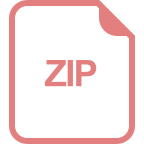
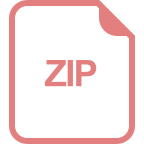
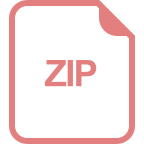
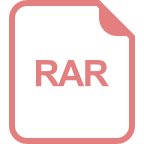
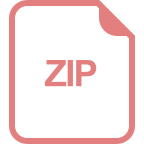
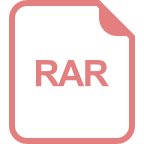
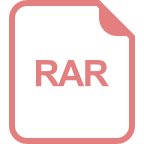
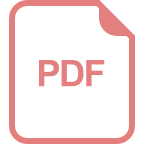
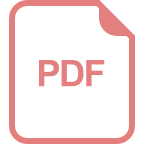
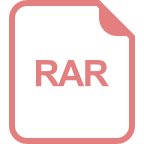
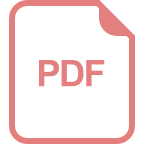
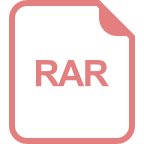
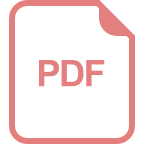