优化这个方法 this.LinksData = function (m_List,transportID) local curRouteConf = {} local tc= mainPlayer.player:FindClosestCharacter(CharacterType.TreasureCase, transportID,0,32) if tc ~= nil then local endPoint = AllPoints[table_count(AllPoints)] table.remove(AllPoints,table_count(AllPoints)) return endPoint end local tc = nil for i = 0,m_List.Count-1 do local value = Logic.TabConfig.RoutePoint_InfoMgr.instance:GetValueByIndex(i) local Links = ListToTable(value.TeleLinks) local route = nil local moveData = nil if mainPlayer.player.CurrentSite >= 200 then if value.ID % 1000 >= 300 and table_find(Links,transportID) ~= false then route = LogicWorld.DynamicMap.instance.m_RoutePoint:FindRoute(mainPlayer.player.transform.position, Vector3.zero, value.ID, 0) tc = mainPlayer.player:FindClosestCharacter(CharacterType.TreasureCase, value.Data,0,32) if route or tc ~= nil then moveData = LogicWorld.DynamicMap.instance.m_RoutePoint:CreateRouteMoveData(mainPlayer.player, route, Vector3.zero) end if not moveData then local temp = {[1] = value.ID,pos = {value.Position[0],value.Position[1],value.Position[2]}} table.insert(AllPoints,temp) local nextPoint = this.LinksData(m_List,value.Data) if nextPoint == nil then table_removeValue(AllPoints,temp) else return nextPoint end else curRouteConf = {value.ID} return curRouteConf; end end else if value.ID % 1000 < 300 and table_find(Links,transportID) ~= false then route = LogicWorld.DynamicMap.instance.m_RoutePoint:FindRoute(mainPlayer.player.transform.position, Vector3.zero, value.ID, 0) if route then moveData = LogicWorld.DynamicMap.instance.m_RoutePoint:CreateRouteMoveData(mainPlayer.player, route, Vector3.zero) end if not moveData then local temp = {[1] = value.ID,pos = {value.Position[0],value.Position[1],value.Position[2]}} table.insert(AllPoints,temp) local nextPoint = this.LinksData(m_List,value.Data) if nextPoint == nil then table_removeValue(AllPoints,temp) else return nextPoint end else curRouteConf = {value.ID} return curRouteConf; end end end end return nil end
时间: 2024-02-20 09:58:33 浏览: 102
There are a few potential optimizations that could be made to this code. Here are a few suggestions:
1. Avoid using `table_count(AllPoints)` and instead keep track of the count of `AllPoints` as a separate variable. Calling `table_count` on every iteration of the loop could be expensive if `AllPoints` is large.
2. Avoid creating a new `ListToTable` table on every iteration of the loop by moving it outside of the loop and storing it in a variable.
3. Instead of calling `table_find(Links, transportID)` on every iteration of the loop, you could use a `for` loop to iterate over `Links` and check if `transportID` is in the list. This would avoid the overhead of the `table_find` function.
4. Consider using Lua's `pairs` function instead of iterating over a `List` object using an index variable. This can make the code more readable and potentially faster.
5. Use local variables wherever possible to avoid global lookups.
Here's an updated version of the function that incorporates these optimizations:
```
function this.LinksData(m_List, transportID)
local curRouteConf = {}
local AllPoints = {}
local closestTc = mainPlayer.player:FindClosestCharacter(CharacterType.TreasureCase, transportID, 0, 32)
if closestTc ~= nil then
local endPoint = AllPoints[#AllPoints]
table.remove(AllPoints)
return endPoint
end
local routePointInfoMgr = Logic.TabConfig.RoutePoint_InfoMgr.instance
local Links = {}
for _, value in pairs(m_List) do
local route = nil
local moveData = nil
Links = Links or ListToTable(value.TeleLinks)
if mainPlayer.player.CurrentSite >= 200 then
if value.ID % 1000 >= 300 then
for _, link in pairs(Links) do
if link == transportID then
route = LogicWorld.DynamicMap.instance.m_RoutePoint:FindRoute(mainPlayer.player.transform.position, Vector3.zero, value.ID, 0)
local closestTc = mainPlayer.player:FindClosestCharacter(CharacterType.TreasureCase, value.Data, 0, 32)
if route or closestTc ~= nil then
moveData = LogicWorld.DynamicMap.instance.m_RoutePoint:CreateRouteMoveData(mainPlayer.player, route, Vector3.zero)
end
if not moveData then
local temp = {[1] = value.ID, pos = {value.Position[0], value.Position[1], value.Position[2]}}
table.insert(AllPoints, temp)
local nextPoint = this.LinksData(m_List, value.Data)
if nextPoint == nil then
table.remove(AllPoints)
else
return nextPoint
end
else
curRouteConf = {value.ID}
return curRouteConf
end
end
end
end
else
if value.ID % 1000 < 300 then
for _, link in pairs(Links) do
if link == transportID then
route = LogicWorld.DynamicMap.instance.m_RoutePoint:FindRoute(mainPlayer.player.transform.position, Vector3.zero, value.ID, 0)
if route then
moveData = LogicWorld.DynamicMap.instance.m_RoutePoint:CreateRouteMoveData(mainPlayer.player, route, Vector3.zero)
end
if not moveData then
local temp = {[1] = value.ID, pos = {value.Position[0], value.Position[1], value.Position[2]}}
table.insert(AllPoints, temp)
local nextPoint = this.LinksData(m_List, value.Data)
if nextPoint == nil then
table.remove(AllPoints)
else
return nextPoint
end
else
curRouteConf = {value.ID}
return curRouteConf
end
end
end
end
end
end
return nil
end
```
阅读全文
相关推荐
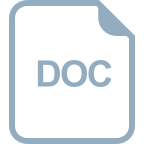
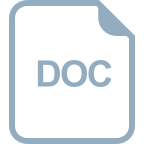
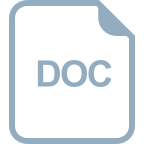
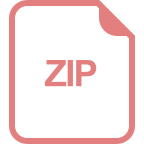
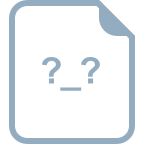
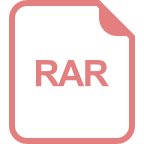
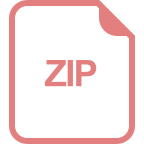
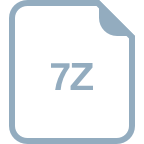
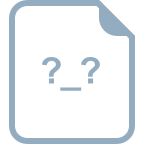
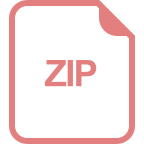
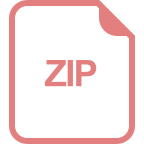
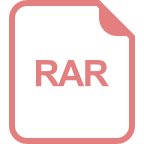
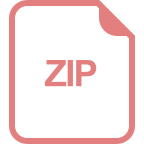
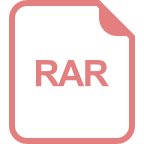
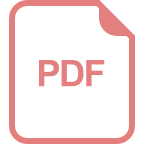
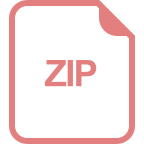
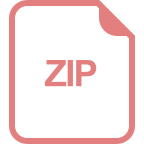
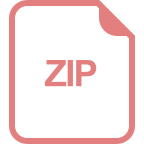
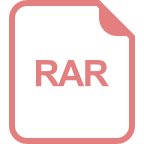