c# datagridview DisplayedCells
时间: 2023-08-10 16:08:28 浏览: 142
In C#, the `DisplayedCells` property of a `DataGridView` control returns a collection of `DataGridViewCell` objects that represents the currently displayed cells on the control. This property allows you to access and manipulate the cells that are currently visible to the user.
Here's an example of how to use the `DisplayedCells` property to iterate over all the visible cells in a `DataGridView` control:
```
foreach (DataGridViewCell cell in dataGridView1.DisplayedCells)
{
// Do something with the cell
}
```
You can use this property to perform operations on the cells that are currently visible to the user, such as formatting, validation, or data manipulation.
相关问题
代码实现将c#datagridview3控件autosizecolumnsmods属性设置未None
在C#中,DataGridView控件提供了多种属性来控制其列的显示方式,包括如何自动调整列的大小。`AutoResizeColumns` 属性可以用来自动调整列的宽度,而`AutoResizeColumnsMode` 属性则用于指定自动调整列大小的模式。`AutoResizeColumnsMode` 属性有以下几个选项:
- `AllCells`:调整所有列以适应其内容的所有单元格。
- `DisplayedCells`:仅调整显示的列,以适应其内容的所有单元格。
- `Fill`:调整列宽以填满DataGridView控件的宽度。
- `None`:不自动调整列宽。
若要将DataGridView控件的`AutoResizeColumnsMode`属性设置为`None`,你可以在代码中直接设置该属性。以下是一个简单的代码示例:
```csharp
// 假设你的DataGridView控件的名称为dataGridView1
dataGridView1.AutoResizeColumnsMode = DataGridViewAutoResizeColumnsMode.None;
```
设置为`None`之后,DataGridView将不会自动调整列宽,列宽将保持你手动设置的大小,或者按照你通过其他方式(如编程方式或用户界面手动调整)指定的大小。
c#datagridview控件根据列自适应列宽,如果列未铺满表格就自动铺满
在C#中,DataGridView控件提供了自动调整列宽的功能,可以通过设置其属性来实现。如果您想要让DataGridView中的列根据内容自适应列宽,同时如果所有列的宽度加起来没有铺满整个DataGridView,让剩余的列自动填充空白区域,可以采用以下方法:
1. 设置`AutoResizeColumns`方法来自适应所有列的宽度。这个方法会根据列中的内容自动调整每个列的宽度。
2. 如果需要让某几列优先填充宽度(例如,第一列和最后一列),可以通过设置`AutoResizeMode`属性为`Fill`来实现。这样会使得未铺满的部分由被设置的列优先填充。
3. 为了确保所有列都能够根据需要调整,可以在窗体加载时或者对数据进行更新后,调用`AutoResizeColumns`方法,并传递`DataGridViewAutoSizeColumnsMode.DisplayedCells`参数,这样会根据当前显示的单元格内容来调整列宽。
示例代码如下:
```csharp
// 调整所有列的宽度以适应其内容
dataGridView1.AutoResizeColumns(DataGridViewAutoSizeColumnsMode.DisplayedCells);
// 如果需要让未铺满的部分由某些列填充,可以设置AutoResizeMode
dataGridView1.AutoSizeColumnsMode = DataGridViewAutoSizeColumnsMode.Fill;
// 也可以根据特定情况,设置特定列的宽度优先级
// 例如,设置第一列和最后一列宽度自适应
dataGridView1.Columns[0].AutoResize(DataGridViewAutoSizeColumnMode.Fill);
dataGridView1.Columns[dataGridView1.Columns.Count - 1].AutoResize(DataGridViewAutoSizeColumnMode.Fill);
```
请根据您的具体需求选择合适的方法来调整DataGridView的列宽。
相关推荐
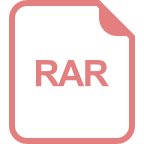
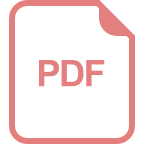
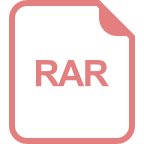
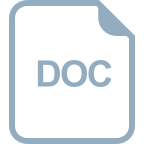
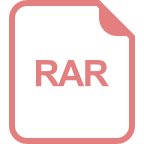
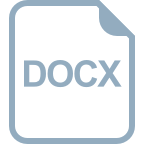
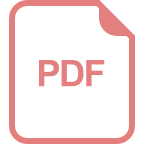
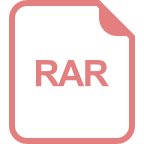
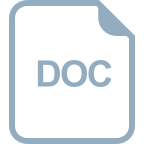


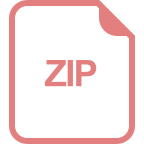
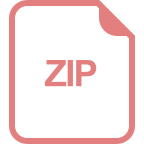