C语言使用ffmept开发将MP4文件倒序输出
时间: 2024-02-06 18:11:35 浏览: 64
可以使用FFmpeg库来实现将MP4文件倒序输出的功能。首先需要使用avformat_open_input()函数打开MP4文件,然后使用avformat_find_stream_info()函数获取流信息。接着使用av_seek_frame()函数将文件指针定位到最后一帧,然后逐帧读取并使用av_write_frame()函数将帧写入到输出文件中。
具体的代码实现可以参考以下示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdint.h>
#include <math.h>
#include <libavformat/avformat.h>
#include <libavcodec/avcodec.h>
#include <libavutil/avutil.h>
#include <libavutil/imgutils.h>
#include <libavutil/opt.h>
#include <libavutil/time.h>
int main(int argc, char *argv[])
{
AVFormatContext *ifmt_ctx = NULL, *ofmt_ctx = NULL;
AVPacket pkt;
int64_t last_dts = 0;
int64_t last_pts = 0;
int64_t duration = 0;
int ret, i;
if (argc != 3) {
printf("Usage: %s input_file output_file\n", argv[0]);
return 1;
}
av_register_all();
if ((ret = avformat_open_input(&ifmt_ctx, argv[1], NULL, NULL)) < 0) {
printf("Cannot open input file '%s': %s\n", argv[1], av_err2str(ret));
return 1;
}
if ((ret = avformat_find_stream_info(ifmt_ctx, NULL)) < 0) {
printf("Cannot find stream information: %s\n", av_err2str(ret));
return 1;
}
if ((ret = avformat_alloc_output_context2(&ofmt_ctx, NULL, NULL, argv[2])) < 0) {
printf("Cannot allocate output context: %s\n", av_err2str(ret));
return 1;
}
for (i = 0; i < ifmt_ctx->nb_streams; i++) {
AVStream *in_stream = ifmt_ctx->streams[i];
AVStream *out_stream = avformat_new_stream(ofmt_ctx, in_stream->codec->codec);
if (!out_stream) {
printf("Cannot create output stream\n");
return 1;
}
if ((ret = avcodec_copy_context(out_stream->codec, in_stream->codec)) < 0) {
printf("Cannot copy codec context: %s\n", av_err2str(ret));
return 1;
}
out_stream->codec->codec_tag = 0;
}
if (!(ofmt_ctx->oformat->flags & AVFMT_NOFILE)) {
if ((ret = avio_open(&ofmt_ctx->pb, argv[2], AVIO_FLAG_WRITE)) < 0) {
printf("Cannot open output file '%s': %s\n", argv[2], av_err2str(ret));
return 1;
}
}
if ((ret = avformat_write_header(ofmt_ctx, NULL)) < 0) {
printf("Cannot write header to output file: %s\n", av_err2str(ret));
return 1;
}
av_seek_frame(ifmt_ctx, -1, INT64_MAX, AVSEEK_FLAG_BACKWARD);
while (1) {
ret = av_read_frame(ifmt_ctx, &pkt);
if (ret < 0)
break;
if (pkt.stream_index >= ifmt_ctx->nb_streams)
continue;
pkt.stream_index = av_find_best_stream(ifmt_ctx, pkt.stream_index, 0, 0, NULL, 0);
if (pkt.stream_index < 0)
continue;
AVStream *in_stream = ifmt_ctx->streams[pkt.stream_index];
AVStream *out_stream = ofmt_ctx->streams[pkt.stream_index];
if (pkt.dts <= last_dts) {
pkt.pts = last_pts - duration;
duration += pkt.duration;
} else {
last_dts = pkt.dts;
last_pts = pkt.pts;
duration = pkt.duration;
}
pkt.dts = pkt.pts = pkt.pts + duration;
if ((ret = av_write_frame(ofmt_ctx, &pkt)) < 0) {
printf("Cannot write frame to output file: %s\n", av_err2str(ret));
return 1;
}
av_packet_unref(&pkt);
}
av_write_trailer(ofmt_ctx);
if (!(ofmt_ctx->oformat->flags & AVFMT_NOFILE))
avio_closep(&ofmt_ctx->pb);
avformat_close_input(&ifmt_ctx);
for (i = 0; i < ofmt_ctx->nb_streams; i++)
avcodec_close(ofmt_ctx->streams[i]->codec);
avformat_free_context(ofmt_ctx);
return 0;
}
```
需要注意的是,该程序仅支持倒序输出单个视频流,如果需要处理多个视频流或者音频流,则需要进行相应的修改。
阅读全文
相关推荐
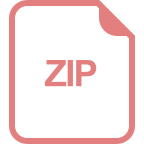
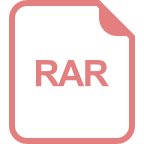
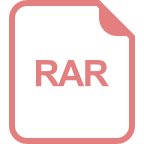
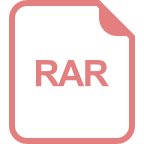
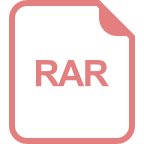
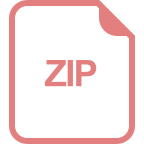
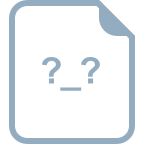
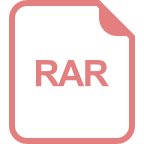