为什么$on接收的数组获取并打印出来了,但赋值给接收到的页面上的数组时在页面上显示为空
时间: 2023-10-04 19:04:51 浏览: 84
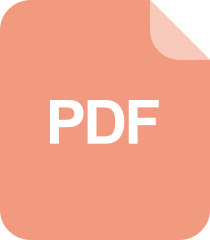
PHP 之 写时复制介绍(Copy On Write)
可能是因为Vue的响应式系统并没有检测到数组的变化。
Vue的响应式系统会在Vue实例被创建时对数据进行劫持,当数据发生变化时,响应式系统会自动通知Vue重新渲染视图。但是,如果你直接对数组进行修改(如使用索引或splice方法),响应式系统可能无法检测到这种变化。因此,如果你需要修改数组,应该使用Vue提供的修改数组的方法,例如push、pop、shift、unshift、splice和sort等。
在你的代码中,如果`this.test`是一个数组,你可以尝试使用Vue提供的修改数组的方法来更新它,例如:
```
this.test.push(res);
```
这样修改后,Vue的响应式系统就会检测到数组的变化,并通知Vue重新渲染视图,页面上的数组也会被正确地更新。
阅读全文
相关推荐
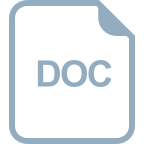
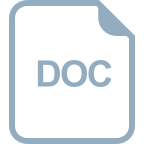
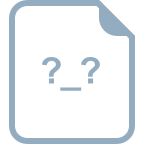
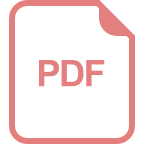
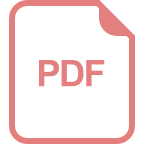
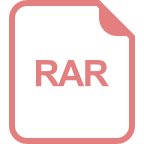
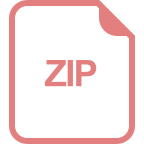
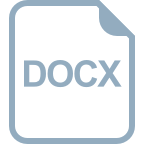
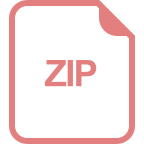
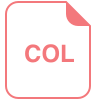
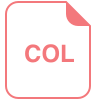
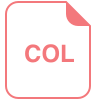
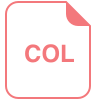




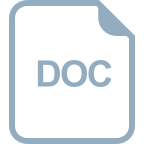
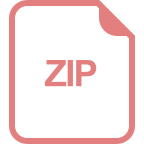