提供一段代码,使用zxing生成二维码,并在二维码上方添加文字信息
时间: 2023-03-15 10:28:50 浏览: 101
String content = "你好,这是一个二维码";
String characterSet = "utf-8";
Bitmap bitmap = ZXingUtils.createQRCode(content, 300, 300, characterSet);// 在二维码上方添加文字
Bitmap newBitmap = ZXingUtils.addTextToQRCode(bitmap, "Hello World", characterSet);
相关问题
提供一段代码,使用zxing生成二维码并添加文字
示例:import java.awt.Color;
import java.awt.Graphics2D;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import java.util.HashMap;
import javax.imageio.ImageIO;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.EncodeHintType;
import com.google.zxing.WriterException;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.QRCodeWriter;
import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel;
public class QRCodeGenerator {
// 生成二维码图片文件
public void generateQRCodeImage(String text, int width, int height, String filePath)
throws WriterException, IOException {
QRCodeWriter qrCodeWriter = new QRCodeWriter();
HashMap<EncodeHintType, Object> hints = new HashMap<EncodeHintType, Object>();
hints.put(EncodeHintType.CHARACTER_SET, "utf-8");
hints.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.M);
hints.put(EncodeHintType.MARGIN, 2);
BitMatrix bitMatrix = qrCodeWriter.encode(text, BarcodeFormat.QR_CODE, width, height, hints);
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
image.createGraphics();
Graphics2D graphics = (Graphics2D) image.getGraphics();
graphics.setColor(Color.WHITE);
graphics.fillRect(0, 0, width, height);
graphics.setColor(Color.BLACK);
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
if (bitMatrix.get(i, j)) {
graphics.fillRect(i, j, 1, 1);
}
}
}
ImageIO.write(image, "png", new File(filePath));
}
}
提供一段代码,使用zxing生成带有文字信息的二维码
### 回答1:
String qrCodeData = "这是一段文字信息";
String filePath = "C:/qrcode.png";try {
//生成二维码QRCode图片
int width = 200;
int height = 200;
String format = "png";
Hashtable hints = new Hashtable();
hints.put(EncodeHintType.CHARACTER_SET, "utf-8");
BitMatrix bitMatrix = new MultiFormatWriter().encode(qrCodeData, BarcodeFormat.QR_CODE, width, height, hints);
Path path = FileSystems.getDefault().getPath(filePath);
MatrixToImageWriter.writeToPath(bitMatrix, format, path);
} catch (Exception e) {
e.printStackTrace();
}答:使用zxing生成带有文字信息的二维码的代码如下:String qrCodeData = "这是一段文字信息";
String filePath = "C:/qrcode.png";try {
//生成二维码QRCode图片
int width = 200;
int height = 200;
String format = "png";
Hashtable hints = new Hashtable();
hints.put(EncodeHintType.CHARACTER_SET, "utf-8");
BitMatrix bitMatrix = new MultiFormatWriter().encode(qrCodeData, BarcodeFormat.QR_CODE, width, height, hints);
Path path = FileSystems.getDefault().getPath(filePath);
MatrixToImageWriter.writeToPath(bitMatrix, format, path);
} catch (Exception e) {
e.printStackTrace();
}
### 回答2:
import java.io.File;
import java.nio.charset.StandardCharsets;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.EncodeHintType;
import com.google.zxing.MultiFormatWriter;
import com.google.zxing.WriterException;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.QRCodeWriter;
import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel;
import com.google.zxing.qrcode.encoder.Encoder;
public class QRCodeGenerator {
public static void main(String[] args) {
String text = "Hello, ZXing!";
String filePath = "qrcode.png";
int width = 300;
int height = 300;
try {
// 设置QR码的纠错等级(L 7% M 15% Q 25% H 30%)
ErrorCorrectionLevel errorCorrectionLevel = ErrorCorrectionLevel.H;
// 创建一个字节矩阵即为QR码
BitMatrix bitMatrix = new QRCodeWriter().encode(text, BarcodeFormat.QR_CODE, width, height,
EncoderHints(EncodeHintType.ERROR_CORRECTION, errorCorrectionLevel));
// 生成二维码的图片格式
File outputFile = new File(filePath);
// 将二维码写入文件
MatrixToImageWriter.writeToFile(bitMatrix, "PNG", outputFile);
System.out.println("QR Code with text '" + text + "' created successfully.");
} catch (WriterException e) {
System.out.println("Error generating QR Code: " + e.getMessage());
}
}
}
以上代码使用zxing库生成带有文字信息的二维码。设置QR码的纠错等级为ErrorCorrectionLevel.H,即最高纠错等级。然后使用QRCodeWriter的encode方法生成QR码的字节矩阵。最后,将字节矩阵写入文件,并输出成功信息。
### 回答3:
使用zxing库生成带有文字信息的二维码的代码如下所示:
```java
import com.google.zxing.BarcodeFormat;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.QRCodeWriter;
import com.google.zxing.WriterException;
import javax.imageio.ImageIO;
import java.awt.*;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
public class QRCodeGenerator {
public static void main(String[] args) {
String text = "这是一个带有文字信息的二维码";
int width = 300;
int height = 300;
String format = "png";
String filePath = "qrcode.png";
try {
// 创建二维码写入器
QRCodeWriter writer = new QRCodeWriter();
BitMatrix bitMatrix = writer.encode(text, BarcodeFormat.QR_CODE, width, height);
// 创建BufferedImage对象
BufferedImage image = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
image.createGraphics();
// 将二维码像素颜色设置为黑白
Graphics2D graphics = (Graphics2D) image.getGraphics();
graphics.setColor(Color.WHITE);
graphics.fillRect(0, 0, width, height);
graphics.setColor(Color.BLACK);
// 根据bitMatrix设置二维码像素颜色
for (int x = 0; x < width; x++) {
for (int y = 0; y < height; y++) {
if (bitMatrix.get(x, y)) {
graphics.fillRect(x, y, 1, 1);
}
}
}
// 在二维码上添加文字信息
graphics.setColor(Color.BLACK);
Font font = new Font("Arial", Font.PLAIN, 12);
graphics.setFont(font);
graphics.drawString("文字信息:" + text, 5, height - 5);
// 保存二维码图片到文件
File qrCodeFile = new File(filePath);
ImageIO.write(image, format, qrCodeFile);
System.out.println("二维码生成成功!");
} catch (WriterException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这段代码使用zxing库生成二维码,将输入的文字信息转换成二维码,并在二维码上方添加文字信息。最后将生成的二维码保存在文件"qrcode.png"中。
相关推荐
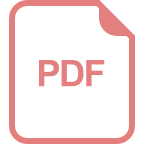
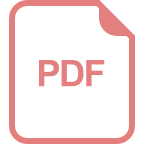
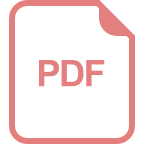












