JAVA 计算设备指定日期的使用产能 单位(分钟) 1.传入工作时段字符串,格式为(HH:mm-HH:mm;HH:mm-HH:mm;HH:mm-HH:mm), 2.传入两个工作起止时间(Date类型) 3.传入一个指定日期 3.返回设备指定日期这天使用了多少产能
时间: 2024-02-03 13:15:05 浏览: 73
可以使用 Java 中的日期时间类(如 `LocalTime`、`LocalDateTime`、`Duration` 等)来实现。以下是一个实现的示例代码:
```java
import java.time.*;
import java.time.format.DateTimeFormatter;
import java.util.List;
import java.util.ArrayList;
public class CapacityCalculator {
// 工作时间段列表,每个元素为一对起止时间
private List<LocalTime[]> workPeriods;
public CapacityCalculator(String workPeriodStr) {
// 解析工作时间段字符串,生成工作时间段列表
workPeriods = new ArrayList<>();
String[] periodStrs = workPeriodStr.split(";");
for (String periodStr : periodStrs) {
String[] times = periodStr.split("-");
LocalTime startTime = LocalTime.parse(times[0], DateTimeFormatter.ofPattern("HH:mm"));
LocalTime endTime = LocalTime.parse(times[1], DateTimeFormatter.ofPattern("HH:mm"));
workPeriods.add(new LocalTime[]{startTime, endTime});
}
}
// 计算指定日期的使用产能
public long calculateCapacity(Date date, Date startTime, Date endTime) {
// 将 Date 转换为 LocalDateTime
LocalDateTime startDateTime = LocalDateTime.ofInstant(startTime.toInstant(), ZoneId.systemDefault());
LocalDateTime endDateTime = LocalDateTime.ofInstant(endTime.toInstant(), ZoneId.systemDefault());
LocalDate targetDate = date.toInstant().atZone(ZoneId.systemDefault()).toLocalDate();
// 统计工作时间段内的总时长
Duration totalDuration = Duration.ZERO;
for (LocalTime[] period : workPeriods) {
LocalDateTime periodStartDateTime = LocalDateTime.of(targetDate, period[0]);
LocalDateTime periodEndDateTime = LocalDateTime.of(targetDate, period[1]);
// 如果时间段和计算区间没有交集,则跳过
if (periodEndDateTime.isBefore(startDateTime) || periodStartDateTime.isAfter(endDateTime)) {
continue;
}
// 限制时间段在计算区间内
if (periodStartDateTime.isBefore(startDateTime)) {
periodStartDateTime = startDateTime;
}
if (periodEndDateTime.isAfter(endDateTime)) {
periodEndDateTime = endDateTime;
}
// 累加工作时间段内的时长
Duration duration = Duration.between(periodStartDateTime, periodEndDateTime);
totalDuration = totalDuration.plus(duration);
}
// 返回使用产能(单位:分钟)
return totalDuration.toMinutes();
}
}
```
使用示例:
```java
// 创建计算器实例
CapacityCalculator calculator = new CapacityCalculator("08:00-12:00;13:00-17:00");
// 计算指定日期的使用产能
Date date = new Date(); // 指定日期
Date startTime = Date.from(LocalDateTime.of(2022, 1, 1, 9, 0).atZone(ZoneId.systemDefault()).toInstant()); // 工作起始时间
Date endTime = Date.from(LocalDateTime.of(2022, 1, 1, 16, 30).atZone(ZoneId.systemDefault()).toInstant()); // 工作结束时间
long capacity = calculator.calculateCapacity(date, startTime, endTime); // 计算产能
System.out.println("使用产能:" + capacity + " 分钟");
```
阅读全文
相关推荐
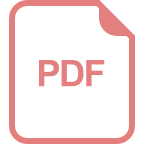
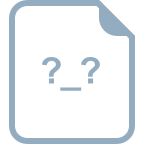










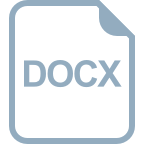
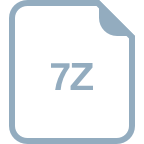