java map中相同的key去重累加
时间: 2023-05-26 09:05:03 浏览: 86
有一个叫做HashMap的类可以实现Java map中相同key去重累加的功能。
例如,我们有一个字符串数组words,要统计每个单词出现的次数:
```java
String[] words = {"apple", "orange", "banana", "apple", "orange", "pear"};
Map<String, Integer> wordCountMap = new HashMap<>();
for (String word : words) {
if (wordCountMap.containsKey(word)) {
wordCountMap.put(word, wordCountMap.get(word) + 1);
} else {
wordCountMap.put(word, 1);
}
}
```
上面的代码中,我们使用了HashMap来记录每个单词出现的次数。如果该单词已经在wordCountMap中存在,则累加该单词的出现次数;否则将该单词及其出现次数添加到map中。
最终,每个单词的出现次数就会被记录在wordCountMap对象中。例如,如果我们输入的是上面的例子,那么HashMap对象wordCountMap中的内容就会是:
```
{"apple":2, "orange":2, "banana":1, "pear":1}
```
其中,每个单词的出现次数都已经累加起来了。
相关问题
Map<String, List<BscBarcodeBo>> map 去重累加
您可以使用Java 8 Stream API中的groupingBy和mapping方法,结合Collectors.toSet和Collectors.summingInt方法来实现去重累加。具体代码如下:
```java
Map<String, List<BscBarcodeBo>> map; // 原始Map
Map<String, Integer> resultMap = map.values().stream()
.flatMap(List::stream)
.collect(Collectors.groupingBy(BscBarcodeBo::getBarcode,
Collectors.mapping(BscBarcodeBo::getNum,
Collectors.toSet())))
.entrySet().stream()
.collect(Collectors.toMap(Map.Entry::getKey,
e -> e.getValue().stream().mapToInt(Integer::intValue).sum()));
// resultMap的key是去重之后的barcode,value是对应的num累加结果。
```
步骤解释:
1. 首先将原始Map中所有BscBarcodeBo对象拆分出来,形成一个大的Stream。
2. 使用groupingBy方法按照BscBarcodeBo对象的barcode字段进行分组。
3. 针对每个分组,在mapping方法中指定BscBarcodeBo对象的num字段作为要做去重累加的字段,然后使用toSet方法进行去重(因为去重后是一个Set,所以使用toSet方法)。
4. 最后得到的结果是一个Map,key值是去重后的barcode,value是一个Set,包含了要做去重累加的所有num值。
5. 对于以上结果中的每一个entry,将entry的value转化为一个IntStream,然后调用sum方法对其进行累加操作。最终得到的结果就是每个barcode对应的num累加结果。
Java List根据string字段去重,相同的对象对int字段累加
可以通过使用Java 8提供的Stream API和Lambda表达式来根据指定的字符串字段对List进行去重操作,并对相同的对象进行整数字段的累加。具体实现步骤如下:
1. 定义一个实体类,包含需要去重和累加的字段,例如:
```
public class Person {
private String name;
private int age;
// 其他字段...
// 构造函数、getter和setter方法...
}
```
2. 初始化一个包含多个Person对象的List,例如:
```
List<Person> personList = new ArrayList<>();
personList.add(new Person("Tom", 20));
personList.add(new Person("Jerry", 18));
personList.add(new Person("Tom", 25));
personList.add(new Person("Jack", 22));
```
3. 使用Stream API进行去重和累加操作,例如:
```
Map<String, Person> resultMap = personList.stream().collect(
Collectors.toMap(
Person::getName, // 指定map的key
Function.identity(), // 指定map的value
(oldValue, newValue) -> { // 指定合并函数,用于累加相同名称的Person对象的age字段
oldValue.setAge(oldValue.getAge() + newValue.getAge());
return oldValue;
}
)
);
List<Person> resultList = new ArrayList<>(resultMap.values()); // 将map转换为List
```
以上代码将根据Person对象的name字段进行去重,并将相同名称的Person对象的age字段进行累加。最终,得到一个新的List对象,其中包含去重后的Person对象(相同名称的Person对象的age字段已经累加)。需要注意的是,此方法得到的Person对象的顺序可能与原始List的顺序不同。
相关推荐
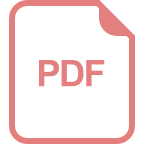
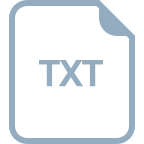







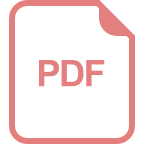
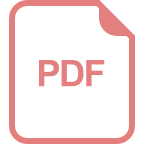
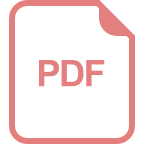