#include "stdio.h" void timecount(struct TIME *p ,int n); void TestFunc(); struct TIME {int h; int m; int s; }; void timecount(struct TIME *p ,int n) { /*********Begin**********/ /********** End **********/ } main() {struct TIME time0; int n; scanf("%d:%d:%d",&time0.h,&time0.m,&time0.s); scanf("%d",&n); timecount(&time0,n); printf("after %d seconds the time is:%d:%d:%d\n",n,time0.h,time0.m,time0.s); /* 输出改变后的时间 */ TestFunc(); } void TestFunc() /* 此函数无需改动 */ { FILE *IN,*OUT; int j,n[2]; struct TIME i[2]; IN=fopen("in.dat","r"); if(IN==NULL) {printf("Read File Error"); } OUT=fopen("out.dat","w"); if(OUT==NULL) {printf("Write File Error"); } for(j=0;j<2;j++) /* 读入两组不同的输入用于测试 */ { fscanf(IN,"%d:%d:%d",&i[j].h,&i[j].m,&i[j].s); fscanf(IN,"%d",&n[j]); } timecount(&i[0],n[0]); timecount(&i[1],n[1]); for(j=0;j<2;j++) fprintf(OUT,"%d:%d:%d\n",i[j].h, i[j].m, i[j].s); fclose(IN); fclose(OUT); }补全该程序
时间: 2024-01-17 09:40:45 浏览: 27
#include "stdio.h"
struct TIME {
int h;
int m;
int s;
};
void timecount(struct TIME *p, int n) {
int carry = 0; // 进位标志
p->s += n; // 秒数加上 n
if (p->s >= 60) { // 判断是否需要进位
carry = p->s / 60;
p->s = p->s % 60;
}
p->m += carry; // 分钟加上进位标志
carry = 0; // 重置进位标志
if (p->m >= 60) { // 判断是否需要进位
carry = p->m / 60;
p->m = p->m % 60;
}
p->h += carry; // 小时加上进位标志
p->h = p->h % 24; // 将小时数调整为合法的范围
}
void TestFunc() {
FILE *IN, *OUT;
int j, n[2];
struct TIME i[2];
IN = fopen("in.dat", "r");
if (IN == NULL) {
printf("Read File Error");
}
OUT = fopen("out.dat", "w");
if (OUT == NULL) {
printf("Write File Error");
}
for (j = 0; j < 2; j++) {
fscanf(IN, "%d:%d:%d", &i[j].h, &i[j].m, &i[j].s);
fscanf(IN, "%d", &n[j]);
}
timecount(&i[0], n[0]);
timecount(&i[1], n[1]);
for (j = 0; j < 2; j++) {
fprintf(OUT, "%d:%d:%d\n", i[j].h, i[j].m, i[j].s);
}
fclose(IN);
fclose(OUT);
}
int main() {
struct TIME time0;
int n;
scanf("%d:%d:%d", &time0.h, &time0.m, &time0.s);
scanf("%d", &n);
timecount(&time0, n);
printf("after %d seconds the time is:%d:%d:%d\n", n, time0.h, time0.m, time0.s);
TestFunc();
return 0;
}
相关推荐
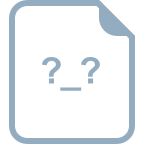
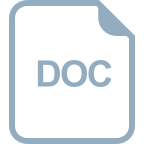
















