在 main 方法中从键盘输入两个整数,依次为:随机种子 seed 和 复数类 Complex 对象的个数 num 。然后随机生成 num 个 Complex 对象存入 cs1 数组中,再随机生成 num 个 Complex 对象存入 cs2 数组中。 然后对 cs1 和 cs2 数组中对应的复数对象依次执行如下操作:⑤ 将 cs1[i] 和 cs2[i] 相除并输出;相除时若捕获到不能相除的异常,则将捕获异常对象的消息输出。
时间: 2023-06-17 14:03:18 浏览: 161
以下是基于 Java 实现的代码:
```java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入随机种子 seed:");
int seed = scanner.nextInt();
System.out.print("请输入复数类 Complex 对象的个数 num:");
int num = scanner.nextInt();
// 根据输入的随机种子和个数生成 Complex 数组
Complex[] cs1 = new Complex[num];
Complex[] cs2 = new Complex[num];
for (int i = 0; i < num; i++) {
cs1[i] = new Complex(Math.random() * 10, Math.random() * 10);
cs2[i] = new Complex(Math.random() * 10, Math.random() * 10);
}
// 对 cs1 和 cs2 数组中对应的复数对象依次执行除法操作并输出
for (int i = 0; i < num; i++) {
try {
Complex result = cs1[i].div(cs2[i]);
System.out.printf("cs1[%d] / cs2[%d] = %s\n", i, i, result.toString());
} catch (ArithmeticException e) {
System.out.printf("cs1[%d] / cs2[%d] 无法相除,错误信息:%s\n", i, i, e.getMessage());
}
}
}
}
class Complex {
private double real; // 复数的实部
private double imag; // 复数的虚部
// 构造函数
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
// 除法操作
public Complex div(Complex other) throws ArithmeticException {
double denominator = other.real * other.real + other.imag * other.imag;
if (denominator == 0) {
throw new ArithmeticException("除数为0");
}
double real = (this.real * other.real + this.imag * other.imag) / denominator;
double imag = (this.imag * other.real - this.real * other.imag) / denominator;
return new Complex(real, imag);
}
// toString 方法,返回复数对象的字符串表示
public String toString() {
if (imag >= 0) {
return String.format("%.2f+%.2fi", real, imag);
} else {
return String.format("%.2f-%.2fi", real, -imag);
}
}
}
```
以上代码中,我们首先通过 `Scanner` 类从控制台读取随机种子和复数对象的个数。然后根据随机种子和个数生成两个 `Complex` 数组 `cs1` 和 `cs2`,其中每个 `Complex` 对象的实部和虚部都是随机生成的。接着,我们对 `cs1` 和 `cs2` 数组中对应的复数对象依次执行除法操作并输出结果。在执行除法操作时,我们使用了 `try-catch` 语句来捕获可能出现的异常,并输出异常对象的消息。如果除数为0,则会抛出 `ArithmeticException` 异常,我们在 `catch` 语句中捕获该异常并输出错误信息。
阅读全文
相关推荐
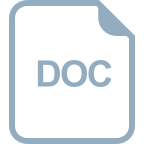
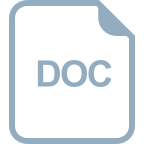
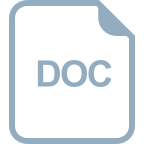
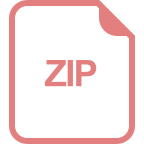
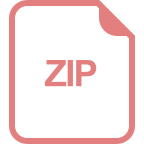
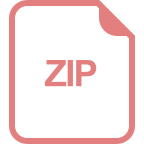
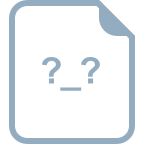
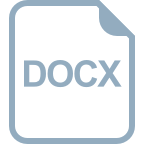
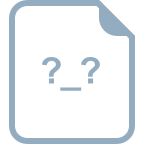
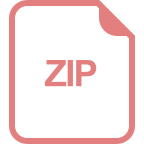
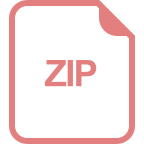
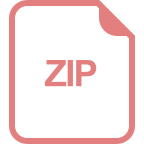
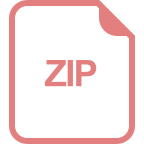
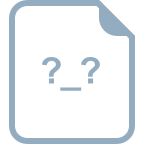
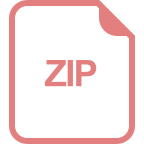
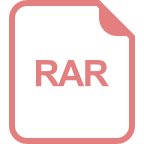
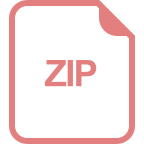
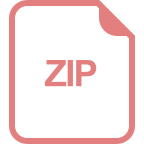
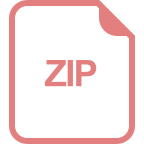