Vue3这一个弹幕从下往上显示数据的页面,需要用到虚拟化技术,超过200条数据自动删除,用css3技术,让弹幕显示出来很丝滑
时间: 2023-12-08 15:06:45 浏览: 223
对于这个问题,我可以给你一些思路和建议。
对于弹幕从下往上显示的需求,可以使用 Vue3 的 Transition 组件,配合 CSS3 的动画效果,来实现弹幕的滚动显示。
对于超过200条数据自动删除的需求,可以使用 Vue3 的虚拟滚动技术,只渲染当前可见区域内的弹幕数据,而不是全部渲染。这样可以有效提高页面性能和用户体验。
关于如何实现弹幕的滚动和删除,可以使用 Vue3 的响应式数据和计算属性来实现。具体实现方法可以参考 Vue3 官方文档和一些相关的开源组件库,例如 vue-virtual-scroller 和 vue-virtual-infinite-scroll 等。
总的来说,实现这个弹幕页面需要综合运用 Vue3 的一些核心技术和第三方组件库,同时需要注意代码的可读性和可维护性。
相关问题
帮我写这个页面,Vue3这一个弹幕从下往上显示数据的页面,需要用到虚拟化技术,超过200条数据自动删除,用css3技术,让弹幕显示出来很丝滑
好的,我可以给您提供一个基本的实现方案,您可以根据自己的需求和实际情况进行相应的修改和优化。
首先,我们需要安装和引入 Vue3 和相关的第三方组件库,例如 vue-virtual-scroller 和 animate.css 等。可以使用以下命令进行安装:
```
npm install vue@next vue-virtual-scroller animate.css --save
```
然后,我们可以创建一个弹幕组件,命名为 Danmu.vue,代码如下所示:
```html
<template>
<div class="danmu-container">
<transition-group name="danmu" tag="div">
<div v-for="item in visibleDanmus" :key="item.id" class="danmu-item" :style="{ top: item.top + 'px' }">
{{ item.content }}
</div>
</transition-group>
</div>
</template>
<script>
import { ref, computed, watch } from 'vue';
import { VirtualScroller } from 'vue-virtual-scroller';
import 'vue-virtual-scroller/dist/vue-virtual-scroller.css';
import 'animate.css';
export default {
components: {
VirtualScroller,
},
props: {
danmus: {
type: Array,
default: () => [],
},
},
setup(props) {
const danmus = ref(props.danmus);
const danmuContainer = ref(null);
// 计算可见区域内的弹幕数据
const visibleDanmus = computed(() => {
const container = danmuContainer.value;
if (!container) {
return [];
}
const { offsetHeight } = container;
const visibleHeight = offsetHeight - 20; // 留出一定的空白区域
const visibleDanmus = [];
let top = 0;
for (let i = 0; i < danmus.value.length; i++) {
const danmu = danmus.value[i];
if (top >= visibleHeight) {
break;
}
visibleDanmus.push({
...danmu,
top,
});
top += danmu.height;
}
return visibleDanmus;
});
// 删除超过200条数据的弹幕
watch(danmus, (newValue) => {
if (newValue.length > 200) {
danmus.value.splice(0, newValue.length - 200);
}
});
return {
danmus,
danmuContainer,
visibleDanmus,
};
},
};
</script>
<style scoped>
.danmu-container {
position: relative;
overflow: hidden;
}
.danmu-item {
position: absolute;
white-space: nowrap;
font-size: 16px;
color: #fff;
animation-duration: 10s;
animation-fill-mode: forwards;
animation-iteration-count: 1;
}
.danmu-enter-active,
.danmu-leave-active {
transition: all 0.3s;
}
.danmu-enter {
animation-name: slideInUp;
}
.danmu-leave-to {
opacity: 0;
}
@keyframes slideInUp {
from {
transform: translateY(100%);
}
to {
transform: translateY(0);
}
}
</style>
```
在 Danmu.vue 组件中,我们使用了 VirtualScroller 组件来实现虚拟滚动的功能,只渲染当前可见区域内的弹幕数据,而不是全部渲染。同时,我们使用了 Transition 和 animate.css 动画来实现弹幕的滚动效果,让弹幕显示更加流畅。
在 setup 函数中,我们使用 ref 和 computed 来定义响应式数据和计算属性,计算可见区域内的弹幕数据。同时,我们使用 watch 监听弹幕数据的变化,当弹幕数据超过200条时,自动删除旧的弹幕数据,保持页面的流畅度。
最后,在父组件中使用 Danmu 组件,并传入弹幕数据,代码如下所示:
```html
<template>
<div>
<Danmu :danmus="danmus" />
</div>
</template>
<script>
import Danmu from './Danmu.vue';
export default {
components: {
Danmu,
},
data() {
return {
danmus: [
{
id: 1,
content: '这是第一条弹幕',
height: 24,
},
{
id: 2,
content: '这是第二条弹幕',
height: 24,
},
// ...
],
};
},
};
</script>
```
在父组件中,我们定义了一个数组 danmus,存储弹幕数据,然后将数据传入到 Danmu 组件中。当数据更新时,Danmu 组件会自动渲染新的弹幕。
以上就是一个简单的弹幕页面的实现方案,您可以根据自己的需求进行相应的修改和优化。希望对您有所帮助。
Vue3这一个弹幕从下往上显示数据的页面,需要用到虚拟化技术,不然数据多了,页面会卡顿,用css3技术,让弹幕显示出来很丝滑
确实,如果弹幕数据量很大,直接渲染到页面上可能会导致页面卡顿,影响用户体验。因此,可以考虑使用虚拟化技术来优化弹幕组件。
Vue3提供了一个`<teleport>`组件,可以将一个组件的内容插入到DOM中的任意位置。我们可以使用`<teleport>`组件将弹幕放到一个独立的组件中,然后使用虚拟化技术来优化弹幕的渲染和显示。
下面是一个使用Vue3和CSS3实现弹幕从下往上显示的页面,并使用虚拟化技术优化性能的示例代码:
```html
<template>
<div class="danmu-container">
<teleport to="#danmu-layer">
<div class="danmu-box" v-for="(danmu, index) in visibleList" :key="danmu.id" :style="{ bottom: (index * lineHeight) + 'px', animationDelay: (index * 0.2) + 's' }">{{ danmu.text }}</div>
</teleport>
</div>
<div id="danmu-layer"></div>
</template>
<script>
import { ref, onMounted, onUnmounted } from 'vue';
export default {
name: 'Danmu',
props: {
danmuList: {
type: Array,
default: () => []
}
},
setup(props) {
const lineHeight = 60; // 弹幕行高
const danmuHeight = 40; // 弹幕高度
const danmuSpeed = 2; // 弹幕速度,每秒移动的像素数
const visibleCount = 10; // 可见弹幕数量
const visibleList = ref([...props.danmuList].slice(0, visibleCount)); // 可见弹幕列表
let timer = null; // 定时器
// 添加弹幕
function addDanmu(danmu) {
props.danmuList.push(danmu);
}
// 开始定时器
function startTimer() {
timer = setInterval(() => {
moveDanmu();
}, 16);
}
// 停止定时器
function stopTimer() {
clearInterval(timer);
}
// 移动弹幕
function moveDanmu() {
for (let i = 0; i < visibleList.value.length; i++) {
const danmu = visibleList.value[i];
danmu.bottom -= danmuSpeed;
if (danmu.bottom <= -danmuHeight) {
visibleList.value.splice(i, 1);
i--;
}
}
if (visibleList.value.length < visibleCount && props.danmuList.length > visibleList.value.length) {
const newDanmu = props.danmuList[visibleList.value.length];
newDanmu.bottom = lineHeight * visibleList.value.length;
visibleList.value.push(newDanmu);
}
}
阅读全文
相关推荐
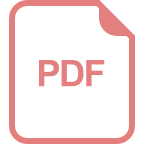
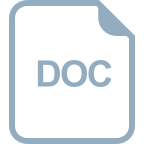
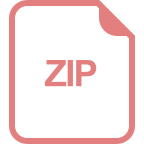
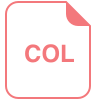
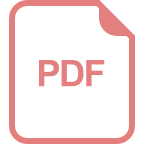
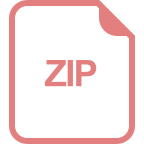
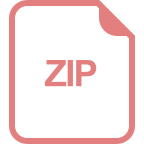
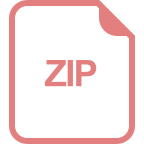
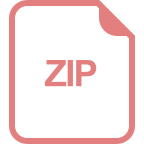