使用springboot集成spring session 和redis
时间: 2023-11-14 08:09:20 浏览: 155
很高兴回答你的问题。使用Spring Boot集成Spring Session和Redis,您可以简单地通过maven使用以下依赖关系:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.session</groupId>
<artifactId>spring-session-data-redis</artifactId>
</dependency>
```
然后,您需要在application.properties文件中进行一些配置,例如:
```
spring.redis.host=localhost
spring.redis.port=6379
spring.session.store-type=redis
```
这样,在您的代码中,您可以轻松使用Spring Session API管理用户会话。希望这可以帮助您开始使用Spring Boot集成Spring Session和Redis。
阅读全文
相关推荐
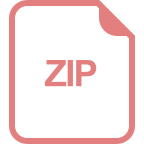
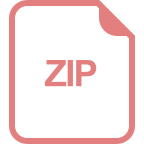
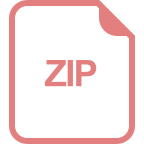
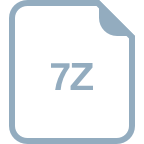
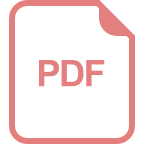
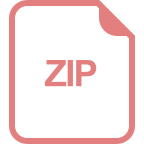
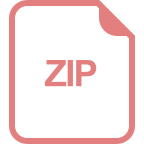
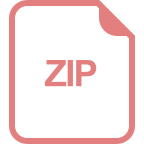
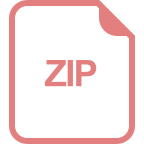
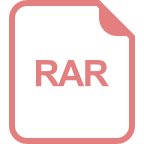
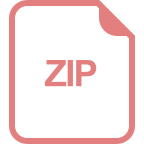
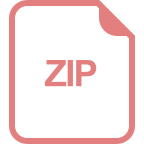
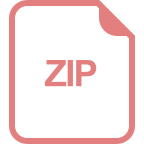
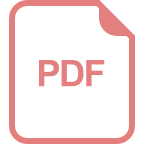
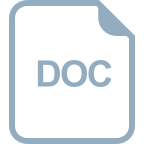
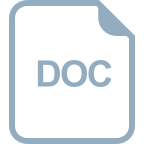