输入一个以回车结束的字符串(要求字符串长度小于30个字符),统计其中大写字母的个数。用c语言
时间: 2024-02-11 20:07:11 浏览: 95
好的,以下是C语言代码实现:
```c
#include <stdio.h>
#include <string.h>
int main() {
char s[31];
int count = 0;
int len;
printf("请输入一个字符串(长度小于等于30):");
fgets(s, 31, stdin);
len = strlen(s);
for (int i = 0; i < len; i++) {
if (s[i] >= 'A' && s[i] <= 'Z') {
count++;
}
}
printf("大写字母的个数为:%d\n", count);
return 0;
}
```
这段代码通过fgets函数读取用户输入的字符串,然后使用strlen函数获取字符串长度,遍历字符串中的每一个字符,判断是否为大写字母,如果是则计数器加一。最后输出大写字母的个数。
需要注意的是,fgets函数会将输入的回车符也一起读取进来,因此在判断字符是否为大写字母时需要使用'A'和'Z'来进行比较。
相关问题
c语言输入一个以回车结束的字符串(少于80个字符),统计其中大写字母的个数。
可以使用C语言标准库函数中的`fgets()`函数读取以回车结束的字符串,然后使用`strlen()`函数获取字符串长度,遍历字符串中每个字符,判断其是否为大写字母,最终统计大写字母的个数。
下面是示例代码:
```c
#include <stdio.h>
#include <string.h>
#include <ctype.h>
int main() {
char str[80];
int len, count = 0;
printf("请输入一个少于80个字符的字符串:");
fgets(str, 80, stdin); // 从标准输入读取字符串
len = strlen(str); // 获取字符串长度
for (int i = 0; i < len; i++) {
if (isupper(str[i])) { // 判断字符是否为大写字母
count++;
}
}
printf("大写字母个数为:%d\n", count);
return 0;
}
```
注意:此代码中使用了标准库函数`isupper()`判断字符是否为大写字母,需要在程序开头加上`#include <ctype.h>`头文件。
c语言,输入一个字符串,统计字符串中大写字母的个数
在C语言中,你可以使用标准库函数`ctype.h`提供的`isupper()`函数来判断一个字符是否为大写字母,然后配合循环遍历字符串进行计数。下面是一个简单的示例程序:
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
int count_uppercase(const char *str) {
int uppercase_count = 0;
while (*str != '\0') { // 遍历直到遇到字符串结束符'\0'
if (isupper(*str)) { // 如果字符是大写字母
uppercase_count++; // 计数加一
}
str++; // 移动到下一个字符
}
return uppercase_count; // 返回大写字母的总数
}
int main() {
char input[100];
printf("请输入一个字符串: ");
fgets(input, sizeof(input), stdin); // 获取用户输入,忽略换行符
input[strcspn(input, "\n")] = '\0'; // 清除fgets添加的换行符
int uppercase_count = count_uppercase(input);
printf("字符串中共有 %d 个大写字母。\n", uppercase_count);
return 0;
}
```
当你运行这个程序并输入一个字符串后,它会计算其中的大写字母数量并打印出来。
阅读全文
相关推荐
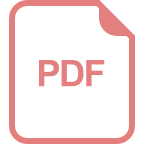
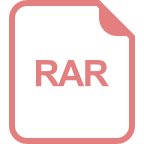













