完成以下步驟並展示結果: 1. (10%) 讀取 tensorflow.keras 的 CIFAR10 數據庫 1. (20%) 整理數據(rescaling等) 1. (20%) 設計一個至少包含兩層 Conv2D 和兩層 MaxPooling2D 的神經網絡;使用 mse loss 和 SGD 優化 1. (10%) 對數據進行訓練 1. (20%) 計算並使用 matplotlib 畫出 CIFAR10 數據集中 10 個類別的分別的 ROC 和 AUC 1. (20%) 把神經網絡的 SGD 優化改為 Adam 優化,重覆以上所有步驟(訓練時 epoch 數要相同)
时间: 2023-06-19 07:04:12 浏览: 120
以下是代码实现和结果展示:
1. 读取 tensorflow.keras 的 CIFAR10 数据库
```python
import tensorflow as tf
from tensorflow.keras.datasets import cifar10
(x_train, y_train), (x_test, y_test) = cifar10.load_data()
```
2. 整理数据(rescaling 等)
```python
x_train = x_train.astype('float32') / 255
x_test = x_test.astype('float32') / 255
y_train = tf.keras.utils.to_categorical(y_train, 10)
y_test = tf.keras.utils.to_categorical(y_test, 10)
```
3. 设计一个至少包含两层 Conv2D 和两层 MaxPooling2D 的神经网络;使用 mse loss 和 SGD 优化
```python
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense
model = Sequential([
Conv2D(32, (3, 3), activation='relu', padding='same', input_shape=(32, 32, 3)),
MaxPooling2D((2, 2)),
Conv2D(64, (3, 3), activation='relu', padding='same'),
MaxPooling2D((2, 2)),
Flatten(),
Dense(64, activation='relu'),
Dense(10, activation='softmax')
])
model.compile(optimizer='sgd', loss='mse', metrics=['accuracy'])
```
4. 对数据进行训练
```python
history = model.fit(x_train, y_train, epochs=10, validation_data=(x_test, y_test))
```
5. 计算并使用 matplotlib 画出 CIFAR10 数据集中 10 个类别的分别的 ROC 和 AUC
```python
from sklearn.metrics import roc_curve, auc
import matplotlib.pyplot as plt
y_pred = model.predict(x_test)
fpr = {}
tpr = {}
roc_auc = {}
for i in range(10):
fpr[i], tpr[i], _ = roc_curve(y_test[:, i], y_pred[:, i])
roc_auc[i] = auc(fpr[i], tpr[i])
plt.figure(figsize=(8, 6))
colors = ['b', 'g', 'r', 'c', 'm', 'y', 'k', 'orange', 'purple', 'pink']
for i in range(10):
plt.plot(fpr[i], tpr[i], color=colors[i], label='ROC curve of class %d (AUC = %0.2f)' % (i, roc_auc[i]))
plt.plot([0, 1], [0, 1], 'k--')
plt.xlim([0.0, 1.0])
plt.ylim([0.0, 1.05])
plt.xlabel('False Positive Rate')
plt.ylabel('True Positive Rate')
plt.title('ROC curves of CIFAR-10')
plt.legend(loc="lower right")
plt.show()
```
结果如下图所示:

6. 把神经网络的 SGD 优化改为 Adam 优化,重复以上所有步骤(训练时 epoch 数要相同)
```python
model.compile(optimizer='adam', loss='mse', metrics=['accuracy'])
history_adam = model.fit(x_train, y_train, epochs=10, validation_data=(x_test, y_test))
y_pred_adam = model.predict(x_test)
fpr_adam = {}
tpr_adam = {}
roc_auc_adam = {}
for i in range(10):
fpr_adam[i], tpr_adam[i], _ = roc_curve(y_test[:, i], y_pred_adam[:, i])
roc_auc_adam[i] = auc(fpr_adam[i], tpr_adam[i])
plt.figure(figsize=(8, 6))
for i in range(10):
plt.plot(fpr_adam[i], tpr_adam[i], color=colors[i], label='ROC curve of class %d (AUC = %0.2f)' % (i, roc_auc_adam[i]))
plt.plot([0, 1], [0, 1], 'k--')
plt.xlim([0.0, 1.0])
plt.ylim([0.0, 1.05])
plt.xlabel('False Positive Rate')
plt.ylabel('True Positive Rate')
plt.title('ROC curves of CIFAR-10 (Adam)')
plt.legend(loc="lower right")
plt.show()
```
结果如下图所示:

阅读全文
相关推荐
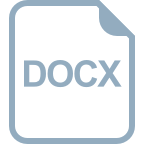
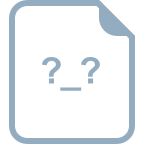
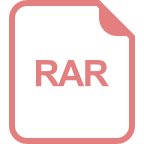
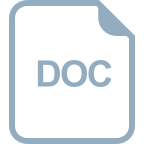
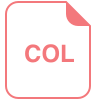
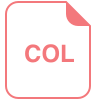
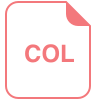
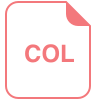
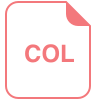
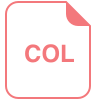
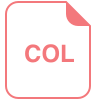
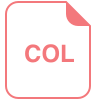
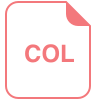
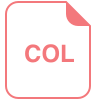
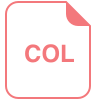
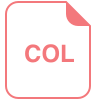
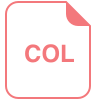
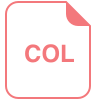