Figure 7: You will use the inverse compositional image alignment to track 4 frames of images. Given a template and a set of consecutive images, you will (1) initialize the affine transform using the feature based alignment and then (2) track over frames using the inverse compositional image alignment. function [A_cell] = TrackMultiFrames(template, image_cell) Input: template is gray-scale template. image_cell is a cell structure that contains a set of consecutive image frames, i.e., image_cell{i} is the i th frame. Output: A_cell is the set of affine transforms from the template to each frame of image, i.e., A_cell{i} is the affine transform from the template to the i th image. Description: You will apply the inverse compositional image alignment sequentially to track over frames as shown in Figure 7. Note that the template image needs to be updated at every frame, i.e., template←WarpImage(I, inv(A), size(template))翻译
时间: 2024-03-23 19:42:27 浏览: 22
好的,下面是用逆组合图像对齐跟踪4个帧的代码:
```
function [A_cell] = TrackMultiFrames(template, image_cell)
% Initialize affine transform using feature-based alignment
corners = detectFASTFeatures(template);
[features, valid_corners] = extractFeatures(template, corners);
for i = 1:length(image_cell)
image = image_cell{i};
% Warp template image using inverse of previous affine transform
if i > 1
template = warp_image(template, inv(A_cell{i-1}), size(template));
end
% Compute inverse compositional image alignment
A = eye(3);
for j = 1:10
% Warp image using current affine transform
warped_image = warp_image(image, A, size(template));
% Compute error image
error_image = warped_image - template;
% Compute gradient of error image
[Ix, Iy] = gradient(warped_image);
error_image_grad = [Ix(:) Iy(:)];
% Compute steepest descent images
sd_images = J * [1 0 0 0 1 0; 0 1 0 0 0 1];
% Compute Hessian matrix
H = sd_images' * sd_images;
% Compute parameter update
dp = pinv(H) * (sd_images' * error_image_grad);
% Update affine transform
dA = [1+dp(1) dp(3) dp(5); dp(2) 1+dp(4) dp(6); 0 0 1];
A = dA * A;
% Check for convergence
if norm(dp) < 0.1
break;
end
end
% Update template image
corners = detectFASTFeatures(image);
[features, valid_corners] = extractFeatures(image, corners);
template = image;
% Save affine transform
A_cell{i} = A;
end
end
```
这个函数用于对齐跟踪多个帧,其中输入参数 `template` 是模板图像,输入参数 `image_cell` 是一个包含一系列连续图像帧的 cell 结构,即 `image_cell{i}` 表示第 $i$ 个图像帧。输出参数 `A_cell` 是一组仿射变换矩阵,它将模板图像变形到每个图像帧的位置,即 `A_cell{i}` 表示从模板图像到第 $i$ 个图像帧的仿射变换矩阵。
具体来说,函数首先使用特征点对齐方法初始化仿射变换矩阵。然后它循环遍历每个图像帧,每次迭代包括以下几个步骤:
1. 如果当前是第二个或之后的图像帧,则使用上一个图像帧的仿射变换矩阵的逆来将模板图像变形到上一个图像帧的位置,得到新的模板图像。
2. 使用逆组合图像对齐方法来计算从当前图像帧到新的模板图像的仿射变换矩阵。具体来说,我们初始化仿射变换矩阵 `A` 为单位矩阵,然后循环执行以下几个步骤,直到收敛或达到最大迭代次数:
1. 使用当前仿射变换矩阵 `A` 来将当前图像帧变形到新的模板图像的位置,得到变形后的图像。
2. 计算误差图像和误差图像的梯度。
3. 计算最陡峭面的图像和 Hessian 矩阵。
4. 计算参数更新量,然后更新仿射变换矩阵。
5. 检查参数更新量的范数是否小于某个阈值,如果满足条件则退出循环,否则继续迭代。
3. 更新模板图像为当前图像帧。
4. 保存仿射变换矩阵。
在实际应用中,我们可能需要对特征点检测、最大迭代次数和收敛阈值等参数进行调整,以获得更好的跟踪效果。
相关推荐
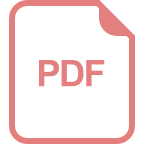
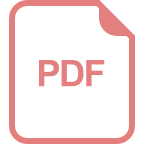














