HashSet<String>迭代输出算法
时间: 2023-05-19 09:05:44 浏览: 71
可以使用Java中的迭代器来遍历HashSet<String>中的元素,具体代码如下:
HashSet<String> set = new HashSet<String>();
// 添加元素
set.add("apple");
set.add("banana");
set.add("orange");
// 迭代输出
Iterator<String> iterator = set.iterator();
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
输出结果为:
apple
banana
orange
相关问题
c# 遍历hashset
在C#中,遍历HashSet可以使用foreach循环或者迭代器。具体实现方法如下:
1.使用foreach循环遍历HashSet:
```
HashSet<string> hashSet = new HashSet<string>();
//向HashSet中添加元素
hashSet.Add("a");
hashSet.Add("b");
hashSet.Add("c");
//使用foreach循环遍历HashSet
foreach (string str in hashSet)
{
Console.WriteLine(str);
}
```
2.使用迭代器遍历HashSet:
```
HashSet<string> hashSet = new HashSet<string>();
//向HashSet中添加元素
hashSet.Add("a");
hashSet.Add("b");
hashSet.Add("c");
//使用迭代器遍历HashSet
IEnumerator<string> enumerator = hashSet.GetEnumerator();
while (enumerator.MoveNext())
{
Console.WriteLine(enumerator.Current);
}
```
需要注意的是,在遍历HashSet时,元素的顺序是不确定的,因为HashSet是按照Hash算法来存储元素的。
用Java实现重用搜索结果的迭代的Anytime repair A*算法
Anytime Repair A* (ARA*)算法是一种启发式搜索算法,旨在快速找到最优解,同时允许在搜索过程中随时停止并返回当前最好的解决方案。 ARA*基于A*算法,但使用了两个重要的技术:任意时间截止和重用搜索结果。重用搜索结果是指,在搜索过程中,如果找到一个状态,它已经被搜索过了,那么可以使用之前的搜索结果来加速搜索过程,从而减少搜索时间。
下面是用Java实现重用搜索结果的迭代的Anytime repair A*算法的示例代码:
```
import java.util.*;
public class ARAStar {
private static final int MAX_COST = Integer.MAX_VALUE;
private Map<String, Integer> gScore;
private Map<String, Integer> hScore;
private Map<String, Integer> fScore;
private PriorityQueue<String> openSet;
private Set<String> closedSet;
private Map<String, String> cameFrom;
private int goalX, goalY;
private int[][] obstacles;
public ARAStar(int[][] obstacles, int goalX, int goalY) {
this.gScore = new HashMap<>();
this.hScore = new HashMap<>();
this.fScore = new HashMap<>();
this.openSet = new PriorityQueue<>(Comparator.comparingInt(fScore::get));
this.closedSet = new HashSet<>();
this.cameFrom = new HashMap<>();
this.obstacles = obstacles;
this.goalX = goalX;
this.goalY = goalY;
}
public List<String> search(String start) {
gScore.put(start, 0);
hScore.put(start, heuristic(start));
fScore.put(start, hScore.get(start));
openSet.add(start);
while (!openSet.isEmpty()) {
String current = openSet.poll();
if (isGoal(current)) {
return reconstructPath(current);
}
if (fScore.get(current) > getMaxFScore()) {
continue;
}
closedSet.add(current);
for (String neighbor : getNeighbors(current)) {
if (closedSet.contains(neighbor)) {
continue;
}
int tentativeGScore = gScore.get(current) + getCost(current, neighbor);
if (!gScore.containsKey(neighbor) || tentativeGScore < gScore.get(neighbor)) {
cameFrom.put(neighbor, current);
gScore.put(neighbor, tentativeGScore);
hScore.put(neighbor, heuristic(neighbor));
fScore.put(neighbor, tentativeGScore + hScore.get(neighbor));
if (!openSet.contains(neighbor)) {
openSet.add(neighbor);
}
}
}
}
return null;
}
private int getCost(String a, String b) {
int ax = Integer.parseInt(a.split(",")[0]);
int ay = Integer.parseInt(a.split(",")[1]);
int bx = Integer.parseInt(b.split(",")[0]);
int by = Integer.parseInt(b.split(",")[1]);
if (obstacles[ax][ay] == MAX_COST || obstacles[bx][by] == MAX_COST) {
return MAX_COST;
}
return Math.abs(ax - bx) + Math.abs(ay - by);
}
private List<String> getNeighbors(String node) {
List<String> neighbors = new ArrayList<>();
int x = Integer.parseInt(node.split(",")[0]);
int y = Integer.parseInt(node.split(",")[1]);
if (x > 0) {
neighbors.add((x - 1) + "," + y);
}
if (y > 0) {
neighbors.add(x + "," + (y - 1));
}
if (x < obstacles.length - 1) {
neighbors.add((x + 1) + "," + y);
}
if (y < obstacles[0].length - 1) {
neighbors.add(x + "," + (y + 1));
}
return neighbors;
}
private boolean isGoal(String node) {
int x = Integer.parseInt(node.split(",")[0]);
int y = Integer.parseInt(node.split(",")[1]);
return x == goalX && y == goalY;
}
private int heuristic(String node) {
int x = Integer.parseInt(node.split(",")[0]);
int y = Integer.parseInt(node.split(",")[1]);
return Math.abs(x - goalX) + Math.abs(y - goalY);
}
private List<String> reconstructPath(String node) {
List<String> path = new ArrayList<>();
path.add(node);
while (cameFrom.containsKey(node)) {
node = cameFrom.get(node);
path.add(0, node);
}
return path;
}
public void setMaxFScore(int maxFScore) {
for (String node : openSet) {
if (fScore.get(node) > maxFScore) {
gScore.put(node, MAX_COST);
fScore.put(node, MAX_COST);
}
}
}
private int getMaxFScore() {
return fScore.get(openSet.peek());
}
}
```
在这个示例中,我们使用了一个二维数组来表示地图和障碍物。 `search`方法接受一个起点,并返回一条路径到达目标点。`setMaxFScore`方法用于更新当前的最大f值,以便搜索更接近目标的路径,并且可以重复使用以前的搜索结果。
阅读全文
相关推荐
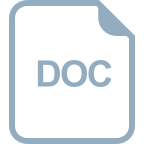
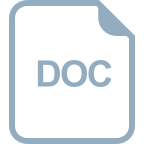
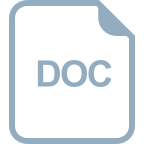
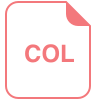
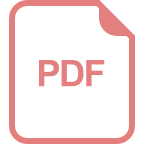
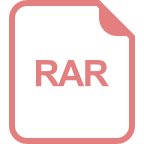
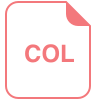
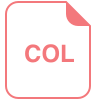
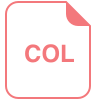
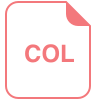
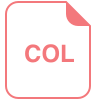
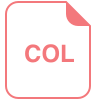
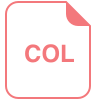
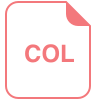
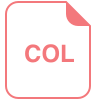
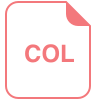
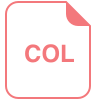