基于51单片机与mlx90614将温度显示到lcd1602显示器上的程序
时间: 2023-12-14 22:02:21 浏览: 69
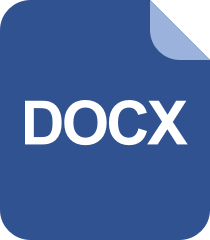
基于51单片机的1602液晶显示温度和时间的C程序.docx
以下是基于51单片机与mlx90614将温度显示到lcd1602显示器上的程序代码:
```c
#include <reg51.h>
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#include "lcd1602.h"
#define FOSC 11059200L //晶振频率
#define BAUD 9600 //串口波特率
#define PRESCALER 12 //定时器1分频系数
#define T1MS (65536-FOSC/1000/PRESCALER) //定时器1的重载值
#define DELAY_MS(x) {unsigned int i; for(i=0;i<x;i++) Delay1ms();} //延时函数
sbit SDA=P1^1; //IIC数据线
sbit SCL=P1^0; //IIC时钟线
sbit ADDR0=P1^2; //IIC地址位
sbit ADDR1=P1^3; //IIC地址位
unsigned char code Init_Temp[]={0x02,0x00,0x00,0x00,0x01,0x60,0x00,0x01}; //初始化命令
unsigned char code Read_Temp[]={0x02,0x00,0x00,0x00,0x00,0x60,0x02,0x00}; //读取温度命令
unsigned char IIC_Start(void); //IIC总线起始信号
void IIC_Stop(void); //IIC总线停止信号
void IIC_SendByte(unsigned char byte); //IIC发送字节
unsigned char IIC_RecvByte(void); //IIC接收字节
unsigned char MLX90614_Init(void); //初始化mlx90614温度传感器
float MLX90614_ReadTemp(void); //从mlx90614传感器读取温度数据
void Delay1ms(void); //1ms延时函数
void InitUART(void); //初始化UART
void SendChar(unsigned char ch); //发送字符
void SendString(unsigned char *s); //发送字符串
void SendFloat(float num, unsigned char point); //发送浮点数
void main(void)
{
float temperature;
unsigned char str[20];
LCD1602_Init(); //初始化LCD1602显示器
InitUART(); //初始化UART
MLX90614_Init(); //初始化mlx90614温度传感器
while(1)
{
temperature = MLX90614_ReadTemp(); //从mlx90614传感器读取温度数据
sprintf(str, "Temp: %.1f C", temperature); //将温度数据格式化为字符串
LCD1602_WriteString(0, 0, str); //在LCD1602显示器上显示温度数据
SendString(str); //通过串口发送温度数据
DELAY_MS(1000); //延时1s
}
}
unsigned char IIC_Start(void)
{
SDA=1;
SCL=1;
DELAY_MS(1);
if(!SDA) return 0;
SDA=0;
DELAY_MS(1);
if(SDA) return 0;
SCL=0;
DELAY_MS(1);
return 1;
}
void IIC_Stop(void)
{
SDA=0;
SCL=1;
DELAY_MS(1);
SDA=1;
DELAY_MS(1);
}
void IIC_SendByte(unsigned char byte)
{
unsigned char i;
for(i=0; i<8; i++)
{
SDA = byte>>7-i & 0x01;
SCL=1;
DELAY_MS(1);
SCL=0;
DELAY_MS(1);
}
SDA=1;
SCL=1;
DELAY_MS(1);
SCL=0;
DELAY_MS(1);
}
unsigned char IIC_RecvByte(void)
{
unsigned char i, byte=0;
SDA=1;
for(i=0; i<8; i++)
{
byte <<= 1;
SCL=1;
DELAY_MS(1);
byte |= SDA;
SCL=0;
DELAY_MS(1);
}
return byte;
}
unsigned char MLX90614_Init(void)
{
unsigned char i;
IIC_Start();
IIC_SendByte(0xB4);
if(IIC_RecvByte() != 0x00)
{
IIC_Stop();
return 0;
}
for(i=0; i<sizeof(Init_Temp); i++)
{
IIC_SendByte(Init_Temp[i]);
if(IIC_RecvByte() != Init_Temp[i])
{
IIC_Stop();
return 0;
}
}
IIC_Stop();
return 1;
}
float MLX90614_ReadTemp(void)
{
unsigned char i;
unsigned char buf[3];
float temp;
IIC_Start();
IIC_SendByte(0xB4);
if(IIC_RecvByte() != 0x00)
{
IIC_Stop();
return 0;
}
for(i=0; i<sizeof(Read_Temp); i++)
{
IIC_SendByte(Read_Temp[i]);
if(IIC_RecvByte() != Read_Temp[i])
{
IIC_Stop();
return 0;
}
}
IIC_Stop();
DELAY_MS(5);
IIC_Start();
IIC_SendByte(0xB5);
if(IIC_RecvByte() != 0x00)
{
IIC_Stop();
return 0;
}
for(i=0; i<3; i++)
{
buf[i] = IIC_RecvByte();
if(i == 1) buf[i] &= 0x1F; //取温度数据的低13位
}
IIC_Stop();
temp = (float)((buf[1]<<8) + buf[0]) * 0.02 - 273.15; //将温度数据转换为摄氏度
return temp;
}
void Delay1ms(void)
{
unsigned char i, j;
i = T1MS / 256;
j = T1MS % 256;
do
{
while(--j);
} while(--i);
}
void InitUART(void)
{
TMOD |= 0x20; //定时器1工作在模式2,8位自动重载
TH1 = 256 - FOSC/12/16/BAUD; //计算波特率重载值
TL1 = TH1;
TR1 = 1; //启动定时器1
SCON = 0x50; //串口工作在模式1,允许接收
ES = 1; //允许串口中断
EA = 1; //开启总中断
}
void SendChar(unsigned char ch)
{
SBUF = ch;
while(!TI);
TI = 0;
}
void SendString(unsigned char *s)
{
while(*s)
{
SendChar(*s++);
}
}
void SendFloat(float num, unsigned char point)
{
unsigned char str[20];
sprintf(str, "%.*f", point, num);
SendString(str);
}
void UART_ISR() interrupt 4
{
if(RI)
{
RI = 0;
}
if(TI)
{
TI = 0;
}
}
```
该程序使用了LCD1602显示器和UART串口,通过IIC总线与mlx90614温度传感器通信,实现了从mlx90614传感器读取温度数据并显示到LCD1602显示器上,并通过串口发送温度数据。
阅读全文
相关推荐
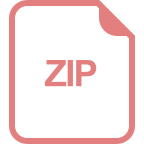

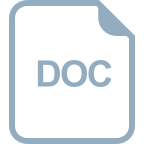
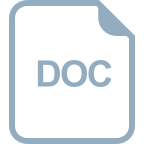
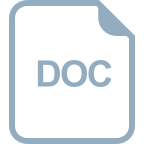
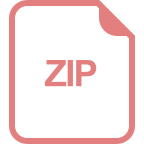
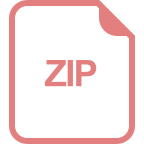
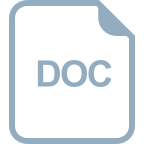
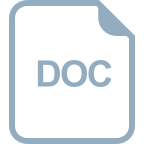

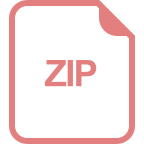
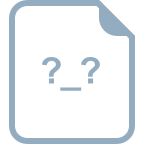
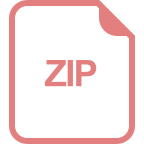