Python的selenium可以写多线程自动化吗,可以的话又是怎么分配工作的呢
时间: 2023-06-26 21:04:51 浏览: 118
是的,Python的selenium可以用多线程实现自动化。多线程可以加速自动化脚本的执行,提高效率。
多线程的实现方式有很多种,其中一种比较简单的方式是使用Python的`threading`模块。具体实现步骤如下:
1. 创建一个线程池,即多个线程
2. 每个线程负责执行一个任务
3. 使用队列将任务分配给每个线程
下面是一个示例代码:
```python
import threading
import queue
from selenium import webdriver
# 创建线程数
THREAD_NUM = 5
# 任务队列
task_queue = queue.Queue()
# 线程执行的任务函数
def run_task():
while not task_queue.empty():
task = task_queue.get()
driver = webdriver.Chrome()
driver.get(task)
# 执行任务...
driver.quit()
# 向任务队列中添加任务
task_queue.put('https://www.example.com/1')
task_queue.put('https://www.example.com/2')
task_queue.put('https://www.example.com/3')
task_queue.put('https://www.example.com/4')
task_queue.put('https://www.example.com/5')
# 创建线程池
threads = []
for i in range(THREAD_NUM):
thread = threading.Thread(target=run_task)
threads.append(thread)
# 启动所有线程
for thread in threads:
thread.start()
# 等待所有线程执行完毕
for thread in threads:
thread.join()
```
在上面的示例代码中,我们创建了一个包含5个线程的线程池,将5个任务添加到任务队列中。每个线程从任务队列中获取一个任务,然后使用selenium模拟浏览器打开网页并执行任务。当任务队列为空时,线程退出。
需要注意的是,多线程并不是越多越好,线程数过多会增加系统的负担,影响执行效率。在实际应用中,需要根据具体情况合理设置线程数。
阅读全文
相关推荐
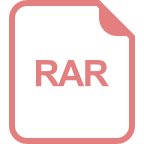
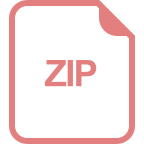
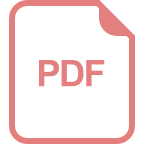
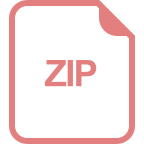
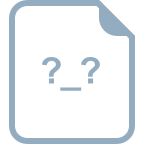
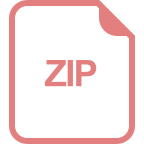
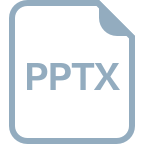
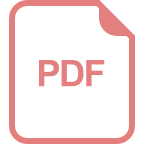
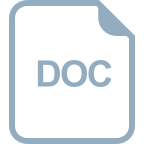
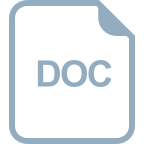