QT使用smtp发送文件到邮箱
时间: 2023-08-16 21:03:25 浏览: 312
在Qt中,可以使用Qt Network模块来发送电子邮件。以下是一个简单的示例代码,用于将文件发送到电子邮件地址。
```cpp
#include <QCoreApplication>
#include <QFile>
#include <QDebug>
#include <QTextStream>
#include <QDateTime>
#include <QFileInfo>
#include <QDir>
#include <QtNetwork/QSslSocket>
#include <QtNetwork/QSmtp>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
// 配置SMTP
QString smtpServer = "smtp.gmail.com";
int smtpPort = 465;
QString smtpUser = "your-email@gmail.com"; // 发件人邮箱
QString smtpPassword = "your-password"; // 发件人邮箱密码
bool useSsl = true;
// 配置邮件信息
QString from = smtpUser;
QString to = "recipient-email@example.com"; // 收件人邮箱
QString subject = "Test Email";
QString body = "This is a test email.";
QString attachmentFilePath = "C:/path/to/file.txt";
// 创建邮件对象
QFileInfo fileInfo(attachmentFilePath);
QFile attachmentFile(attachmentFilePath);
if (!attachmentFile.open(QIODevice::ReadOnly))
{
qDebug() << "Failed to open attachment file.";
return -1;
}
QByteArray attachmentData = attachmentFile.readAll();
QByteArray attachmentEncodedData = attachmentData.toBase64();
QString attachmentFileName = fileInfo.fileName();
attachmentFile.close();
// 发送邮件
QSmtp smtp;
if (useSsl)
{
smtp.connectToHostEncrypted(smtpServer, smtpPort);
}
else
{
smtp.connectToHost(smtpServer, smtpPort);
}
if (!smtp.login(smtpUser, smtpPassword))
{
qDebug() << "Failed to login.";
return -1;
}
smtp.sendMail(from, to, subject, body, &attachmentEncodedData, &attachmentFileName);
smtp.quit();
return a.exec();
}
```
在以上代码中,我们首先配置了SMTP服务器的相关信息,然后创建了一个包含附件的电子邮件对象,并使用SMTP协议将其发送到指定的收件人邮箱。
阅读全文
相关推荐








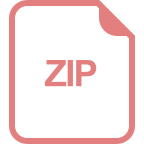



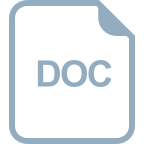


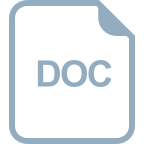
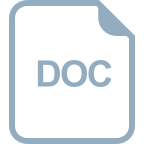

