promise怎么返回一个自定义函数
时间: 2023-04-09 15:01:02 浏览: 102
可以使用箭头函数来返回一个自定义函数,例如:
```
const promise = new Promise((resolve, reject) => {
const customFunction = () => {
console.log('This is a custom function');
};
resolve(customFunction);
});
promise.then((func) => {
func();
});
```
在 Promise 的回调函数中,定义一个自定义函数,并将其作为参数传递给 resolve 函数。在 then 方法中,可以获取到这个自定义函数并执行它。
相关问题
如何在微信小程序JS中调用自定义函数,获取返回值
要在微信小程序JS中调用自定义函数并获取返回值,可以使用回调函数或者Promise对象来实现。
1. 使用回调函数
在调用自定义函数时,传入一个回调函数,在自定义函数执行完毕后,将结果作为参数传入回调函数中。例如:
```
function myFunction(param1, param2, callback) {
// 执行一些操作
// ...
// 返回结果
const result = "some result";
// 调用回调函数,将结果作为参数传入
callback(result);
}
```
在调用该函数时,传入一个回调函数:
```
myFunction(param1, param2, function(result) {
console.log(result);
});
```
当myFunction函数执行完毕后,回调函数会被调用,并将结果作为参数传入,可以在回调函数中处理结果。
2. 使用Promise对象
在自定义函数中,创建一个Promise对象,并在Promise中执行异步操作,当异步操作完成时,调用resolve方法将结果返回。例如:
```
function myFunction(param1, param2) {
return new Promise(function(resolve, reject) {
// 异步操作
setTimeout(function() {
// 返回结果
const result = "some result";
// 调用resolve方法,将结果返回
resolve(result);
}, 1000);
});
}
```
在调用该函数时,可以使用then方法获取返回值:
```
myFunction(param1, param2).then(function(result) {
console.log(result);
});
```
当myFunction函数执行完毕后,Promise对象会调用then方法,并将结果作为参数传入,可以在then方法中处理结果。
自定义Promise.catch()
在 JavaScript 中,Promise.catch() 方法用于捕获 Promise 链中的错误。它是 Promise.prototype.catch() 方法的简写形式。
你可以通过如下方式自定义 Promise.catch() 方法:
```javascript
Promise.prototype.customCatch = function(onRejected) {
return this.then(undefined, onRejected);
};
```
上述代码中,我们将自定义的 catch 方法命名为 `customCatch`。它实际上是在 Promise.prototype 上添加了一个新的方法,该方法会调用原始的 `then` 方法,并传入 undefined 作为成功回调函数参数,以及自定义的 `onRejected` 函数作为错误回调函数参数。
使用自定义的 `customCatch` 方法时,可以像使用原生的 `catch` 方法一样使用它:
```javascript
myPromise
.then(response => {
// 处理成功情况
})
.customCatch(error => {
// 处理错误情况
});
```
这样,无论是调用原生的 `catch` 方法还是自定义的 `customCatch` 方法,都能捕获到 Promise 链中的错误。
阅读全文
相关推荐
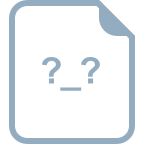
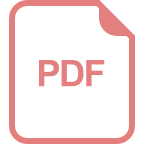
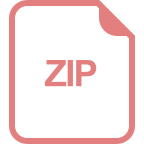
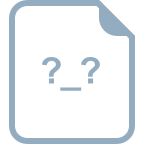
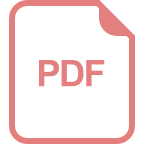
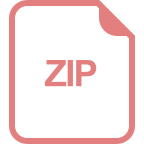
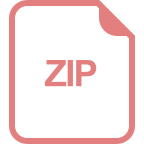
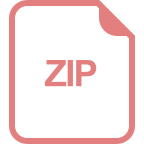
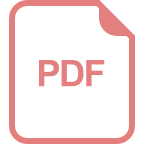
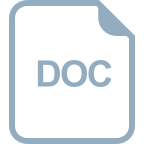
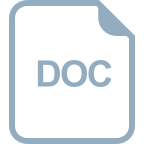
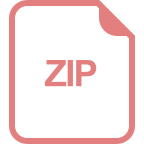
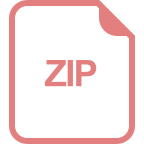
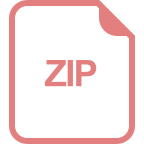
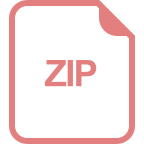