编写Java代码合并分布式系统HDFS上特定后缀名的文件,并通过浏览器查看文件合并前后的界面
时间: 2024-03-17 15:40:10 浏览: 17
以下是一个简单的Java代码示例,用于合并HDFS上指定后缀名的文件。代码使用了Hadoop的API,并且需要在Hadoop集群上运行。
```java
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.URI;
import java.util.ArrayList;
import java.util.List;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.FileUtil;
import org.apache.hadoop.fs.Path;
public class MergeFiles {
public static void main(String[] args) throws IOException {
// 获取 Hadoop 配置
Configuration conf = new Configuration();
// 获取 HDFS 文件系统
FileSystem fs = FileSystem.get(URI.create("hdfs://namenode:9000"), conf);
// 指定要合并的文件夹
Path inputDir = new Path("/input/");
// 指定合并后的文件名
String mergedFileName = "merged.txt";
// 指定要合并的文件后缀名
String fileSuffix = ".txt";
// 获取文件夹中所有指定后缀名的文件
List<Path> inputPaths = new ArrayList<Path>();
for (Path path : FileUtil.stat2Paths(fs.listStatus(inputDir))) {
if (path.getName().endsWith(fileSuffix)) {
inputPaths.add(path);
}
}
// 合并文件
Path mergedFile = new Path(inputDir, mergedFileName);
OutputStream out = fs.create(mergedFile);
for (Path path : inputPaths) {
InputStream in = fs.open(path);
try {
byte[] buffer = new byte[4096];
int bytesRead = -1;
while ((bytesRead = in.read(buffer)) > 0) {
out.write(buffer, 0, bytesRead);
}
} finally {
in.close();
}
}
out.close();
System.out.println("Files merged successfully.");
}
}
```
在合并完文件后,可以使用Java内置的HTTP服务器在浏览器中查看合并前后的文件。以下是一个简单的示例:
```java
import java.io.IOException;
import java.net.InetSocketAddress;
import com.sun.net.httpserver.HttpExchange;
import com.sun.net.httpserver.HttpHandler;
import com.sun.net.httpserver.HttpServer;
public class ViewMergedFile {
public static void main(String[] args) throws IOException {
// 指定 HTTP 服务器端口号
int port = 8000;
// 指定要显示的文件名
String fileName = "merged.txt";
// 获取 HTTP 服务器实例
HttpServer server = HttpServer.create(new InetSocketAddress(port), 0);
// 注册 HTTP 请求处理器
server.createContext("/", new HttpHandler() {
@Override
public void handle(HttpExchange exchange) throws IOException {
byte[] response = fileName.getBytes();
exchange.sendResponseHeaders(200, response.length);
exchange.getResponseBody().write(response);
exchange.close();
}
});
// 启动 HTTP 服务器
server.start();
System.out.println("Server started successfully.");
}
}
```
启动以上两个Java程序后,可以在浏览器中访问 http://localhost:8000/merged.txt 来查看合并后的文件。
相关推荐
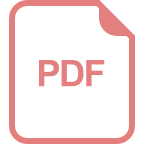
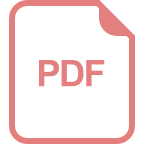
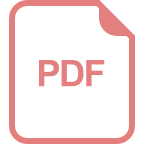













