生成一套使用FastDDS框架,能够进行文件传输的代码
时间: 2023-07-15 20:15:46 浏览: 247
以下是使用FastDDS框架进行文件传输的基本代码:
1. 首先,在你的项目中导入FastDDS库和相关头文件。
```cpp
#include <fastdds/dds/domain/DomainParticipantFactory.hpp>
#include <fastdds/dds/domain/DomainParticipant.hpp>
#include <fastdds/dds/publisher/DataWriter.hpp>
#include <fastdds/dds/subscriber/DataReader.hpp>
#include <fastdds/dds/subscriber/SampleInfo.hpp>
#include <fastrtps/utils/eClock.h>
#include <fastrtps/Domain.h>
#include <fastrtps/qos/QosPolicies.h>
#include <fastrtps/attributes/ParticipantAttributes.h>
#include <fastrtps/attributes/PublisherAttributes.h>
#include <fastrtps/attributes/SubscriberAttributes.h>
#include <fastrtps/attributes/TopicAttributes.h>
#include <fastrtps/attributes/WriterAttributes.h>
#include <fastrtps/attributes/ReaderAttributes.h>
```
2. 定义文件传输所需的数据结构。
```cpp
struct FileMsg {
std::string name;
std::vector<uint8_t> data;
};
```
3. 定义发布者和订阅者的QoS。
```cpp
eprosima::fastdds::dds::DataWriterQos writer_qos;
eprosima::fastdds::dds::DataReaderQos reader_qos;
// Set policies to enable large data messages
writer_qos.history().kind = eprosima::fastrtps::KEEP_ALL_HISTORY_QOS;
writer_qos.resource_limits().max_samples = INT32_MAX;
writer_qos.resource_limits().allocated_samples = INT32_MAX;
writer_qos.reliability().kind = eprosima::fastrtps::RELIABLE_RELIABILITY_QOS;
reader_qos.history().kind = eprosima::fastrtps::KEEP_ALL_HISTORY_QOS;
reader_qos.resource_limits().max_samples = INT32_MAX;
reader_qos.resource_limits().allocated_samples = INT32_MAX;
reader_qos.reliability().kind = eprosima::fastrtps::RELIABLE_RELIABILITY_QOS;
```
4. 创建发布者和订阅者所需的配置。
```cpp
eprosima::fastdds::dds::DomainParticipantQos participant_qos;
eprosima::fastdds::dds::PublisherQos publisher_qos;
eprosima::fastdds::dds::SubscriberQos subscriber_qos;
eprosima::fastdds::dds::TopicQos topic_qos;
eprosima::fastdds::dds::DataWriterQos writer_qos;
eprosima::fastdds::dds::DataReaderQos reader_qos;
// Set the participant QoS
participant_qos.name("Participant_sub");
// Set the publisher QoS
publisher_qos.qos.m_publishMode.kind = eprosima::fastrtps::PublishModeQosPolicyKind::ASYNCHRONOUS_PUBLISH_MODE;
publisher_qos.qos.m_disablePositiveACKs.enabled = true;
// Set the subscriber QoS
subscriber_qos.qos.m_subscriptionMatching.ignore_name = true;
subscriber_qos.qos.m_subscriptionMatching.ignore_user_data = true;
```
5. 创建参与者、主题、发布者和订阅者。
```cpp
// Create the DomainParticipant
eprosima::fastdds::dds::DomainParticipant* participant_ = eprosima::fastdds::dds::DomainParticipantFactory::get_instance()->create_participant(0, participant_qos);
// Create the Topic
eprosima::fastdds::dds::Topic* topic_ = participant_->create_topic("file_topic", "FileMsg", topic_qos);
// Create the Publisher
eprosima::fastdds::dds::Publisher* publisher_ = participant_->create_publisher(publisher_qos);
// Create the Subscriber
eprosima::fastdds::dds::Subscriber* subscriber_ = participant_->create_subscriber(subscriber_qos);
```
6. 创建发布者和订阅者所需的Writer和Reader。
```cpp
// Create the DataWriter
eprosima::fastdds::dds::DataWriter* writer_ = publisher_->create_datawriter(topic_, writer_qos);
// Create the DataReader
eprosima::fastdds::dds::DataReader* reader_ = subscriber_->create_datareader(topic_, reader_qos);
```
7. 发布者发送文件数据。
```cpp
void send_file(const std::string& file_path) {
std::ifstream file(file_path, std::ios::binary | std::ios::ate);
if (!file.is_open()) {
std::cout << "Unable to open file: " << file_path << std::endl;
return;
}
// Get the file size
std::streamsize file_size = file.tellg();
file.seekg(0, std::ios::beg);
// Read the file into a buffer
std::vector<uint8_t> buffer(file_size);
if (!file.read((char*)buffer.data(), file_size)) {
std::cout << "Error reading file: " << file_path << std::endl;
return;
}
// Create the FileMsg object
FileMsg msg;
msg.name = file_path.substr(file_path.find_last_of("/\\") + 1);
msg.data = buffer;
// Publish the message
writer_->write(&msg);
}
```
8. 订阅者接收文件数据。
```cpp
void receive_file() {
FileMsg file_msg;
eprosima::fastdds::dds::SampleInfo info;
if (reader_->take_next_sample(&file_msg, &info) == eprosima::fastrtps::types::ReturnCode_t::RETCODE_OK) {
if (info.valid_data) {
std::ofstream file(file_msg.name, std::ios::binary);
if (!file.is_open()) {
std::cout << "Unable to create file: " << file_msg.name << std::endl;
return;
}
// Write the data to the file
file.write((char*)file_msg.data.data(), file_msg.data.size());
std::cout << "Received file: " << file_msg.name << std::endl;
}
}
}
```
这些是基本的FastDDS文件传输代码。你需要根据你的具体需求进行修改和扩展。
阅读全文
相关推荐
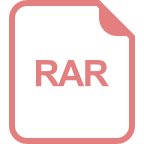






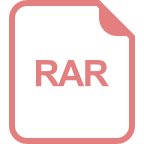

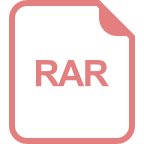





