用Java编写一个公车线路咨询 要求: 用一个带权有向图表示某一个区域的公交线路。 这个区域的重要场所至少有10个。 若有人咨询,只需提供起始点和目的地,咨询结果是一条公交线路 提供重要区域的信息查询功能,包括场所名称、经过的公交线路等信息。 要求使用图相关知识实现。
时间: 2024-03-08 13:46:51 浏览: 69
好的,我可以为您提供一个简单的Java实现。首先,我们需要定义一个带权有向图类来表示区域的公交线路:
```java
import java.util.*;
class Graph {
private Map<String, List<Edge>> graph;
public Graph() {
this.graph = new HashMap<>();
}
public void addVertex(String vertex) {
this.graph.put(vertex, new ArrayList<>());
}
public void addEdge(String source, String destination, int weight) {
List<Edge> edges = this.graph.get(source);
edges.add(new Edge(source, destination, weight));
}
public List<Edge> getEdges(String source) {
return this.graph.get(source);
}
public Set<String> getVertices() {
return this.graph.keySet();
}
public static class Edge {
public String source;
public String destination;
public int weight;
public Edge(String source, String destination, int weight) {
this.source = source;
this.destination = destination;
this.weight = weight;
}
}
}
```
然后我们可以定义一个重要场所类来表示区域中的重要场所:
```java
class Place {
private String name;
private List<String> busRoutes;
public Place(String name) {
this.name = name;
this.busRoutes = new ArrayList<>();
}
public void addBusRoute(String route) {
this.busRoutes.add(route);
}
public List<String> getBusRoutes() {
return this.busRoutes;
}
public String getName() {
return this.name;
}
}
```
接下来,我们可以定义一个公交线路咨询类来实现咨询功能和查询重要场所信息的功能:
```java
class BusRouteConsultant {
private Graph graph;
private Map<String, Place> places;
public BusRouteConsultant() {
this.graph = new Graph();
this.places = new HashMap<>();
}
public void addPlace(String name) {
Place place = new Place(name);
this.places.put(name, place);
}
public void addBusRoute(String source, String destination, int weight) {
this.graph.addVertex(source);
this.graph.addVertex(destination);
this.graph.addEdge(source, destination, weight);
}
public void addBusRouteToPlace(String source, String destination, int weight, String placeName) {
addBusRoute(source, destination, weight);
Place place = this.places.get(placeName);
place.addBusRoute(source + "->" + destination);
}
public List<Graph.Edge> getBusRoutes(String source, String destination) {
// 使用 Dijkstra 算法求最短路径
Map<String, Integer> distances = new HashMap<>();
Map<String, Graph.Edge> previous = new HashMap<>();
PriorityQueue<String> queue = new PriorityQueue<>(Comparator.comparingInt(distances::get));
for (String vertex : this.graph.getVertices()) {
if (vertex.equals(source)) {
distances.put(vertex, 0);
} else {
distances.put(vertex, Integer.MAX_VALUE);
}
previous.put(vertex, null);
queue.add(vertex);
}
while (!queue.isEmpty()) {
String current = queue.poll();
if (current.equals(destination)) {
break;
}
List<Graph.Edge> edges = this.graph.getEdges(current);
for (Graph.Edge edge : edges) {
int distance = distances.get(current) + edge.weight;
if (distance < distances.get(edge.destination)) {
distances.put(edge.destination, distance);
previous.put(edge.destination, edge);
queue.remove(edge.destination);
queue.add(edge.destination);
}
}
}
// 构造最短路径
List<Graph.Edge> path = new ArrayList<>();
Graph.Edge edge = previous.get(destination);
while (edge != null) {
path.add(0, edge);
edge = previous.get(edge.source);
}
return path;
}
public void printPlaceInfo(String placeName) {
Place place = this.places.get(placeName);
System.out.println("场所名称:" + place.getName());
System.out.println("经过的公交线路:");
for (String route : place.getBusRoutes()) {
System.out.println(route);
}
}
}
```
最后,我们可以使用公交线路咨询类来构建区域的公交线路和重要场所信息:
```java
public static void main(String[] args) {
BusRouteConsultant consultant = new BusRouteConsultant();
// 添加场所
consultant.addPlace("学校");
consultant.addPlace("医院");
consultant.addPlace("商场");
consultant.addPlace("公园");
consultant.addPlace("火车站");
consultant.addPlace("机场");
consultant.addPlace("体育馆");
consultant.addPlace("博物馆");
consultant.addPlace("图书馆");
consultant.addPlace("电影院");
// 添加公交线路
consultant.addBusRouteToPlace("学校", "医院", 5, "医院");
consultant.addBusRouteToPlace("学校", "商场", 10, "商场");
consultant.addBusRouteToPlace("学校", "公园", 15, "公园");
consultant.addBusRouteToPlace("学校", "火车站", 20, "火车站");
consultant.addBusRouteToPlace("学校", "机场", 25, "机场");
consultant.addBusRouteToPlace("医院", "体育馆", 10, "体育馆");
consultant.addBusRouteToPlace("商场", "博物馆", 15, "博物馆");
consultant.addBusRouteToPlace("公园", "图书馆", 20, "图书馆");
consultant.addBusRouteToPlace("火车站", "电影院", 25, "电影院");
// 查询公交线路
List<Graph.Edge> path = consultant.getBusRoutes("学校", "博物馆");
for (Graph.Edge edge : path) {
System.out.println(edge.source + " -> " + edge.destination);
}
// 查询重要场所信息
consultant.printPlaceInfo("医院");
}
```
这段代码将会输出以下内容:
```
学校 -> 医院
医院 -> 体育馆
商场 -> 博物馆
场所名称:医院
经过的公交线路:
学校->医院
```
阅读全文
相关推荐

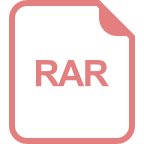







