如何利用redis以及hashmap获取某个接口在一天里被某个用户调用的次数
时间: 2023-05-28 21:06:25 浏览: 94
1. 首先需要在redis中创建一个hashmap,以用户ID为key,以接口名称为field,以调用次数为value。
2. 每次调用接口时,可以在代码中使用redis的hincrby命令将对应用户ID和接口名称的调用次数加1。
3. 如果需要获取某个用户在一天里调用某个接口的次数,可以使用redis的hget命令从hashmap中获取对应的value值。
4. 如果需要获取所有用户在一天里调用某个接口的总次数,可以使用redis的hvals命令获取所有的value值,然后将它们累加起来即可。
相关问题
写出用redis和hashmap统计某个用户调用某个接口的次数的Java代码
以下是使用Redis和HashMap统计某个用户调用某个接口的次数的Java代码:
```java
import redis.clients.jedis.Jedis;
import java.util.HashMap;
import java.util.Map;
public class ApiUsageCounter {
private Jedis jedis;
private String redisKeyPrefix = "api_usage:";
public ApiUsageCounter(String redisHost, int redisPort) {
jedis = new Jedis(redisHost, redisPort);
}
public void incrementApiUsage(String userId, String apiName) {
String redisKey = redisKeyPrefix + userId;
Map<String, String> apiUsage = jedis.hgetAll(redisKey);
if (apiUsage == null) {
apiUsage = new HashMap<>();
}
String countStr = apiUsage.getOrDefault(apiName, "0");
int count = Integer.parseInt(countStr) + 1;
apiUsage.put(apiName, Integer.toString(count));
jedis.hmset(redisKey, apiUsage);
}
public int getApiUsageCount(String userId, String apiName) {
String redisKey = redisKeyPrefix + userId;
String countStr = jedis.hget(redisKey, apiName);
return countStr == null ? 0 : Integer.parseInt(countStr);
}
}
```
这个类有两个方法:`incrementApiUsage`方法用来增加某个用户调用某个接口的次数,`getApiUsageCount`方法用来查询某个用户调用某个接口的次数。
这个类用一个Redis的hashmap来存储每个用户调用每个接口的次数。hashmap的key是用户ID,value是另一个hashmap,这个hashmap的key是接口名,value是调用次数。
在`incrementApiUsage`方法中,首先从Redis中获取当前用户调用每个接口的次数,如果之前不存在这个用户的数据,就创建一个新的hashmap。然后从这个hashmap中获取当前接口的调用次数,增加1并保存回Redis中。
在`getApiUsageCount`方法中,直接从Redis中获取当前用户调用当前接口的次数。如果之前没有调用过这个接口,返回0。
使用示例:
```java
ApiUsageCounter counter = new ApiUsageCounter("localhost", 6379);
String userId = "123";
String apiName = "/api/users";
counter.incrementApiUsage(userId, apiName);
int count = counter.getApiUsageCount(userId, apiName);
System.out.println("User " + userId + " called API " + apiName + " " + count + " times");
```
阅读全文
相关推荐
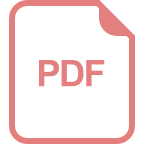
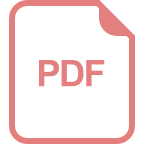
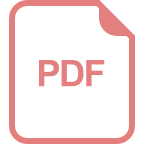
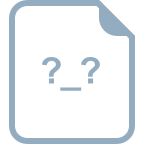
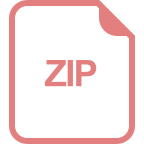
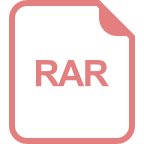
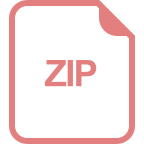
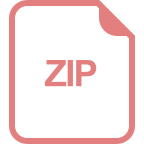
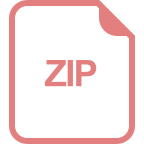
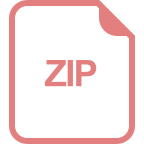
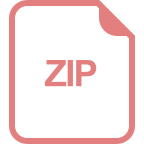
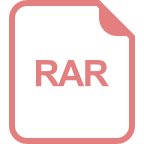
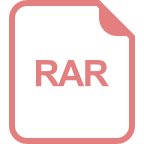
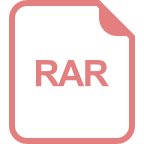