能帮我写一个基于token认证的drf的认证代码吗
时间: 2023-09-11 20:05:12 浏览: 134
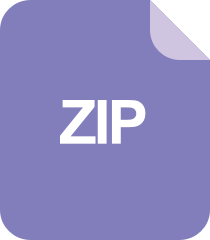
Token验证的代码

### 回答1:
当然可以! 下面是一个使用 `TokenAuthentication` 的例子:
```
from rest_framework.authentication import TokenAuthentication
class ExampleView(APIView):
authentication_classes = [TokenAuthentication]
permission_classes = [IsAuthenticated]
def get(self, request, format=None):
content = {
'status': 'request was permitted'
}
return Response(content)
```
这段代码中,我们使用 `TokenAuthentication` 作为认证类,并使用 `IsAuthenticated` 权限类。这样,只有在认证成功时,才会允许请求通过。
希望这能帮到你!
### 回答2:
当然可以帮你写一个基于token认证的DRF(Django Rest Framework)的认证代码。首先,确保你已经在Django的MIDDLEWARE设置中添加了以下中间件:
```python
MIDDLEWARE = [
...
'django.contrib.auth.middleware.AuthenticationMiddleware',
...
]
```
然后,在你的项目的设置文件(settings.py)中,添加REST_FRAMEWORK的配置项:
```python
REST_FRAMEWORK = {
'DEFAULT_AUTHENTICATION_CLASSES': [
'rest_framework.authentication.TokenAuthentication',
],
}
```
接下来,创建一个自定义的认证类,用于处理token认证逻辑。在你的应用的认证文件(auth.py)里面,添加如下代码:
```python
from rest_framework.authentication import TokenAuthentication
from rest_framework import exceptions
class CustomTokenAuthentication(TokenAuthentication):
def authenticate_credentials(self, key):
model = self.get_model()
try:
token = model.objects.get(key=key)
except model.DoesNotExist:
raise exceptions.AuthenticationFailed('Invalid token')
if not token.user.is_active:
raise exceptions.AuthenticationFailed('User inactive or deleted')
return (token.user, token)
```
然后,在你的视图文件(views.py)中,使用这个自定义的认证类进行认证:
```python
from rest_framework.views import APIView
from rest_framework.authentication import SessionAuthentication
from .auth import CustomTokenAuthentication
class ExampleView(APIView):
authentication_classes = [CustomTokenAuthentication, SessionAuthentication]
def get(self, request, format=None):
# 处理GET请求的逻辑
...
```
这样,你的DRF视图就使用了基于token认证的自定义认证类了。
注意,你还需要在数据库中创建Token模型,并在每次用户登录或注册时生成一个唯一的token,并将其保存在数据库中。另外,需要为每个用户关联一个token。
### 回答3:
当然可以帮您编写一个基于Token认证的Django REST framework(DRF)的认证代码。
首先,您需要在DRF的设置文件中启用Token认证。在settings.py中添加以下内容:
```python
REST_FRAMEWORK = {
'DEFAULT_AUTHENTICATION_CLASSES': [
'rest_framework.authentication.TokenAuthentication',
],
}
```
接下来,您需要创建一个用于Token认证的自定义视图。在您的views.py文件中添加以下代码:
```python
from rest_framework.authtoken.views import ObtainAuthToken
from rest_framework.authtoken.models import Token
from rest_framework.response import Response
class CustomAuthToken(ObtainAuthToken):
def post(self, request, *args, **kwargs):
serializer = self.serializer_class(data=request.data, context={'request': request})
serializer.is_valid(raise_exception=True)
user = serializer.validated_data['user']
token, created = Token.objects.get_or_create(user=user)
return Response({'token': token.key})
```
这个自定义视图继承自DRF自带的ObtainAuthToken视图,并覆写了post方法。在post方法中,我们验证用户的凭证,然后获取或创建对应的Token,并返回给用户。
最后,您需要将自定义视图添加到URL路由中。在您的urls.py文件中添加以下代码:
```python
from .views import CustomAuthToken
urlpatterns = [
# ...其他URL配置...
path('api/token-auth/', CustomAuthToken.as_view(), name='token_auth'),
]
```
配置完以上代码后,您可以向`/api/token-auth/` URL发送POST请求,包含正确的用户名和密码,即可获得Token作为响应。
以上就是一个基于Token认证的DRF认证代码的示例。您可以根据自己的项目需求进行调整和扩展。希望能够帮到您!
阅读全文
相关推荐
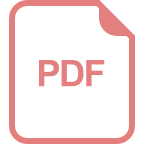
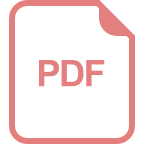
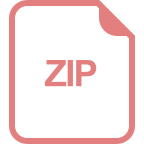
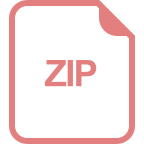
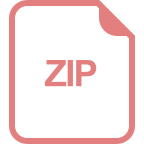
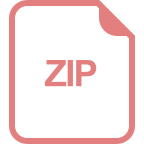
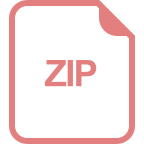
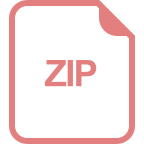
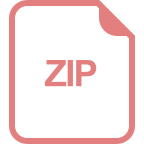
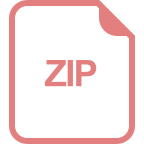
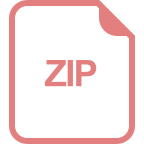
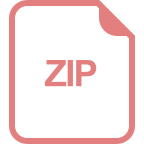
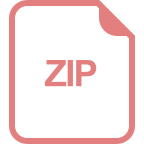
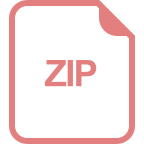
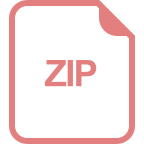
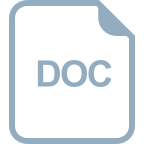
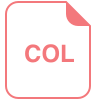
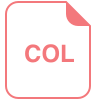