设计一个简单的Linux二级文件系统并给出代码
时间: 2023-07-16 11:14:18 浏览: 141
我可以提供一个简单的Linux二级文件系统的设计思路和代码示例。
设计思路:
这是一个基于内存的简单文件系统,它的主要组成部分包括:
1. SuperBlock:超级块,存储了整个文件系统的信息,如块大小、块数量、inode数量等。
2. Inode:索引节点,存储了文件或目录的元数据,如文件类型、权限、大小、创建时间、修改时间等。
3. Block:数据块,存储了文件的实际数据。
4. Directory Entry:目录项,存储了目录下的文件或目录的名称和对应的inode号。
代码示例:
以下是一个简单的Linux二级文件系统的代码示例,包括了SuperBlock、Inode、Block和Directory Entry的定义和相关操作函数的实现。
```C
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define BLOCK_SIZE 512
#define INODE_SIZE 64
#define DIR_ENTRY_SIZE 32
/* SuperBlock结构体 */
struct SuperBlock {
int block_size; // 块大小
int block_count; // 块数量
int inode_count; // inode数量
int free_block_count; // 空闲块数量
int free_inode_count; // 空闲inode数量
};
/* Inode结构体 */
struct Inode {
int type; // 文件类型,0表示文件,1表示目录
int permission; // 权限
int size; // 大小
int block_count; // 数据块数量
int blocks[10]; // 数据块指针
time_t ctime; // 创建时间
time_t mtime; // 修改时间
};
/* Block结构体 */
struct Block {
char data[BLOCK_SIZE];
};
/* Directory Entry结构体 */
struct DirEntry {
char name[28]; // 文件或目录名
int inode; // inode号
};
/* 初始化超级块 */
void init_super_block(struct SuperBlock *sb, int block_count, int inode_count) {
sb->block_size = BLOCK_SIZE;
sb->block_count = block_count;
sb->inode_count = inode_count;
sb->free_block_count = block_count;
sb->free_inode_count = inode_count;
}
/* 初始化inode */
void init_inode(struct Inode *inode, int type, int permission) {
inode->type = type;
inode->permission = permission;
inode->size = 0;
inode->block_count = 0;
memset(inode->blocks, 0, sizeof(inode->blocks));
inode->ctime = time(NULL);
inode->mtime = time(NULL);
}
/* 分配一个空闲inode */
int alloc_inode(struct SuperBlock *sb) {
if (sb->free_inode_count == 0) {
printf("Error: no free inode\n");
return -1;
}
int inode = sb->inode_count - sb->free_inode_count;
sb->free_inode_count--;
return inode;
}
/* 分配一个空闲数据块 */
int alloc_block(struct SuperBlock *sb) {
if (sb->free_block_count == 0) {
printf("Error: no free block\n");
return -1;
}
int block = sb->block_count - sb->free_block_count;
sb->free_block_count--;
return block;
}
/* 释放一个inode */
void free_inode(struct SuperBlock *sb, int inode) {
sb->free_inode_count++;
}
/* 释放一个数据块 */
void free_block(struct SuperBlock *sb, int block) {
sb->free_block_count++;
}
/* 获取inode对应的数据块 */
int get_block(struct Inode *inode, int index) {
if (index < 10) {
return inode->blocks[index];
}
printf("Error: file too large\n");
return -1;
}
/* 设置inode对应的数据块 */
void set_block(struct Inode *inode, int index, int block) {
if (index < 10) {
inode->blocks[index] = block;
inode->block_count++;
} else {
printf("Error: file too large\n");
}
}
/* 读取数据块 */
void read_block(struct Block *block, int fd, int offset) {
fseek(fd, offset * BLOCK_SIZE, SEEK_SET);
fread(block->data, BLOCK_SIZE, 1, fd);
}
/* 写入数据块 */
void write_block(struct Block *block, int fd, int offset) {
fseek(fd, offset * BLOCK_SIZE, SEEK_SET);
fwrite(block->data, BLOCK_SIZE, 1, fd);
}
/* 根据inode号读取inode */
void read_inode(struct Inode *inode, int fd, int inode_num) {
fseek(fd, sizeof(struct SuperBlock) + inode_num * INODE_SIZE, SEEK_SET);
fread(inode, INODE_SIZE, 1, fd);
}
/* 根据inode号写入inode */
void write_inode(struct Inode *inode, int fd, int inode_num) {
fseek(fd, sizeof(struct SuperBlock) + inode_num * INODE_SIZE, SEEK_SET);
fwrite(inode, INODE_SIZE, 1, fd);
}
/* 根据目录项名字查找inode号 */
int find_dir_entry(struct Block *block, char *name) {
int i;
struct DirEntry *de;
for (i = 0; i < BLOCK_SIZE / DIR_ENTRY_SIZE; i++) {
de = (struct DirEntry *)(block->data + i * DIR_ENTRY_SIZE);
if (strcmp(de->name, name) == 0) {
return de->inode;
}
}
return -1;
}
/* 在目录下创建一个文件 */
void create_file(struct SuperBlock *sb, struct Inode *dir_inode, char *name, int permission) {
if (dir_inode->type != 1) {
printf("Error: not a directory\n");
return;
}
int inode_num = alloc_inode(sb);
if (inode_num == -1) {
return;
}
int block_num = alloc_block(sb);
if (block_num == -1) {
free_inode(sb, inode_num);
return;
}
struct Inode inode;
init_inode(&inode, 0, permission);
set_block(&inode, 0, block_num);
write_inode(&inode, fd, inode_num);
struct Block block;
memset(&block, 0, sizeof(block));
write_block(&block, fd, block_num);
struct DirEntry de;
strncpy(de.name, name, 28);
de.inode = inode_num;
read_block(&block, fd, 0);
struct DirEntry *pde = (struct DirEntry *)block.data;
int i;
for (i = 0; i < BLOCK_SIZE / DIR_ENTRY_SIZE; i++) {
if (pde->inode == 0) {
memcpy(pde, &de, DIR_ENTRY_SIZE);
write_block(&block, fd, 0);
return;
}
pde++;
}
printf("Error: directory is full\n");
free_inode(sb, inode_num);
free_block(sb, block_num);
}
/* 在目录下创建一个目录 */
void create_dir(struct SuperBlock *sb, struct Inode *dir_inode, char *name, int permission) {
if (dir_inode->type != 1) {
printf("Error: not a directory\n");
return;
}
int inode_num = alloc_inode(sb);
if (inode_num == -1) {
return;
}
int block_num = alloc_block(sb);
if (block_num == -1) {
free_inode(sb, inode_num);
return;
}
struct Inode inode;
init_inode(&inode, 1, permission);
set_block(&inode, 0, block_num);
write_inode(&inode, fd, inode_num);
struct Block block;
memset(&block, 0, sizeof(block));
write_block(&block, fd, block_num);
struct DirEntry de;
strncpy(de.name, name, 28);
de.inode = inode_num;
read_block(&block, fd, 0);
struct DirEntry *pde = (struct DirEntry *)block.data;
int i;
for (i = 0; i < BLOCK_SIZE / DIR_ENTRY_SIZE; i++) {
if (pde->inode == 0) {
memcpy(pde, &de, DIR_ENTRY_SIZE);
write_block(&block, fd, 0);
return;
}
pde++;
}
printf("Error: directory is full\n");
free_inode(sb, inode_num);
free_block(sb, block_num);
}
/* 初始化文件系统 */
void init_filesystem(char *filename, int block_count, int inode_count) {
FILE *fd = fopen(filename, "w+");
if (fd == NULL) {
printf("Error: cannot create file system\n");
return;
}
struct SuperBlock sb;
init_super_block(&sb, block_count, inode_count);
fwrite(&sb, sizeof(sb), 1, fd);
struct Inode root_inode;
init_inode(&root_inode, 1, 0777);
write_inode(&root_inode, fd, 0);
struct Block root_block;
memset(&root_block, 0, sizeof(root_block));
struct DirEntry de;
strncpy(de.name, ".", 28);
de.inode = 0;
memcpy(root_block.data, &de, DIR_ENTRY_SIZE);
strncpy(de.name, "..", 28);
de.inode = 0;
memcpy(root_block.data + DIR_ENTRY_SIZE, &de, DIR_ENTRY_SIZE);
write_block(&root_block, fd, 0);
fclose(fd);
}
/* 打开文件系统 */
void open_filesystem(char *filename) {
fd = fopen(filename, "r+");
if (fd == NULL) {
printf("Error: cannot open file system\n");
return;
}
fread(&sb, sizeof(sb), 1, fd);
}
/* 关闭文件系统 */
void close_filesystem() {
fclose(fd);
}
/* 创建文件 */
void create_file(char *path, int permission) {
int inode_num = 0;
char *p = strtok(path, "/");
while (p != NULL) {
struct Inode inode;
read_inode(&inode, fd, inode_num);
int block_num = get_block(&inode, 0);
struct Block block;
read_block(&block, fd, block_num);
inode_num = find_dir_entry(&block, p);
if (inode_num == -1) {
create_file(&sb, &inode, p, permission);
return;
}
p = strtok(NULL, "/");
}
printf("Error: file already exists\n");
}
/* 创建目录 */
void create_dir(char *path, int permission) {
int inode_num = 0;
char *p = strtok(path, "/");
while (p != NULL) {
struct Inode inode;
read_inode(&inode, fd, inode_num);
int block_num = get_block(&inode, 0);
struct Block block;
read_block(&block, fd, block_num);
inode_num = find_dir_entry(&block, p);
if (inode_num == -1) {
create_dir(&sb, &inode, p, permission);
return;
}
p = strtok(NULL, "/");
}
printf("Error: directory already exists\n");
}
/* 删除文件 */
void delete_file(char *path) {
int inode_num = 0;
char *p = strtok(path, "/");
while (p != NULL) {
struct Inode inode;
read_inode(&inode, fd, inode_num);
int block_num = get_block(&inode, 0);
struct Block block;
read_block(&block, fd, block_num);
inode_num = find_dir_entry(&block, p);
if (inode_num == -1) {
printf("Error: file not found\n");
return;
}
p = strtok(NULL, "/");
}
struct Inode inode;
read_inode(&inode, fd, inode_num);
if (inode.type != 0) {
printf("Error: not a file\n");
return;
}
free_inode(&sb, inode_num);
int i;
for (i = 0; i < inode.block_count; i++) {
free_block(&sb, inode.blocks[i]);
}
}
/* 删除目录 */
void delete_dir(char *path) {
int inode_num = 0;
char *p = strtok(path, "/");
while (p != NULL) {
struct Inode inode;
read_inode(&inode, fd, inode_num);
int block_num = get_block(&inode, 0);
struct Block block;
read_block(&block, fd, block_num);
inode_num = find_dir_entry(&block, p);
if (inode_num == -1) {
printf("Error: directory not found\n");
return;
}
p = strtok(NULL, "/");
}
struct Inode inode;
read_inode(&inode, fd, inode_num);
if (inode.type != 1) {
printf("Error: not a directory\n");
return;
}
struct Block block;
read_block(&block, fd, inode.blocks[0]);
struct DirEntry *de = (struct DirEntry *)block.data;
int i;
for (i = 0; i < BLOCK_SIZE / DIR_ENTRY_SIZE; i++) {
if (de->inode != 0) {
struct Inode child_inode;
read_inode(&child_inode, fd, de->inode);
if (child_inode.type == 0) {
free_inode(&sb, de->inode);
int j;
for (j = 0; j < child_inode.block_count; j++) {
free_block(&sb, child_inode.blocks[j]);
}
} else {
delete_dir(child_inode.blocks[0]);
free_inode(&sb, de->inode);
free_block(&sb, child_inode.blocks[0]);
}
}
de++;
}
free_inode(&sb, inode_num);
free_block(&sb, inode.blocks[0]);
}
/* 列出目录下的文件和目录 */
void list_dir(char *path) {
int inode_num = 0;
char *p = strtok(path, "/");
while (p != NULL) {
struct Inode inode;
read_inode(&inode, fd, inode_num);
int block_num = get_block(&inode, 0);
struct Block block;
read_block(&block, fd, block_num);
inode_num = find_dir_entry(&block, p);
if (inode_num == -1) {
printf("Error: directory not found\n");
return;
}
p = strtok(NULL, "/");
}
struct Inode inode;
read_inode(&inode, fd, inode_num);
if (inode.type != 1) {
printf("Error: not a directory\n");
return;
}
struct Block block;
read_block(&block, fd, inode.blocks[0]);
struct DirEntry *de = (struct DirEntry *)block.data;
int i;
for (i = 0; i < BLOCK_SIZE / DIR_ENTRY_SIZE; i++) {
if (de->inode != 0) {
struct Inode child_inode;
read_inode(&child_inode, fd, de->inode);
if (child_inode.type == 0) {
printf("%s\n", de->name);
} else {
printf("%s/\n", de->name);
}
}
de++;
}
}
int main() {
init_filesystem("test.fs", 1024, 128);
open_filesystem("test.fs");
create_dir("/", 0777);
create_file("/test.txt", 0666);
list_dir("/");
delete_file("/test.txt");
delete_dir("/");
close_filesystem();
return 0;
}
```
以上代码仅供参考,可能存在不完善的地方,仅供学习和交流。
阅读全文
相关推荐
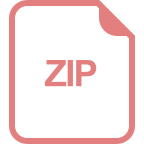
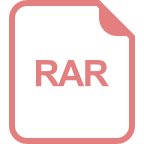
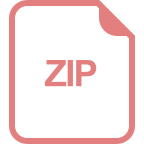
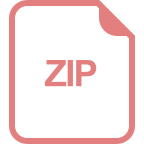
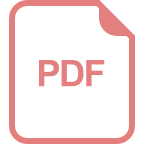
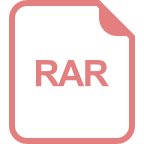
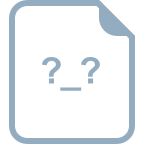
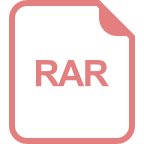
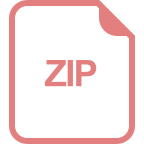
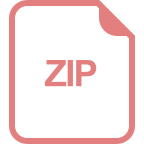
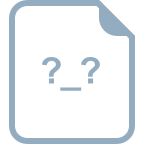
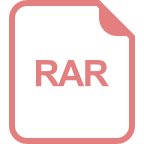
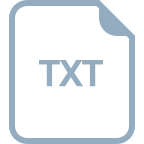
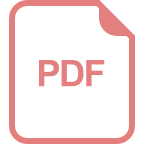
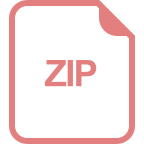
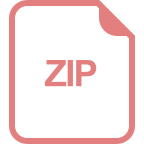
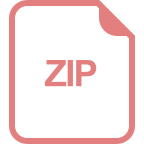
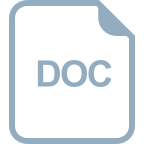
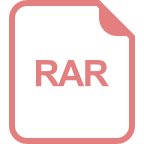