c++中unordered_set用法
时间: 2023-05-31 22:20:09 浏览: 316
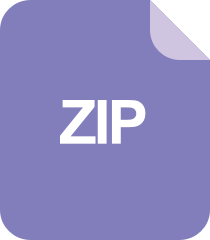
unordered_flat_set:C ++连续内存哈希容器。-开源
### 回答1:
unordered_set是C++ STL中的一个容器,用于存储一组不重复的元素,其内部实现是基于哈希表的。unordered_set中的元素是无序的,但是可以通过哈希函数快速查找元素。
unordered_set的用法和其他STL容器类似,可以使用insert()函数向其中插入元素,使用erase()函数删除元素,使用find()函数查找元素等。另外,unordered_set还提供了一些其他的成员函数,如size()、empty()、clear()等。
unordered_set的定义方式如下:
```c++
#include <unordered_set>
using namespace std;
unordered_set<int> mySet; //定义一个存储int类型元素的unordered_set
```
在使用unordered_set时,需要注意以下几点:
1. unordered_set中的元素必须是可哈希的,即需要定义哈希函数和相等比较函数。
2. unordered_set中的元素是无序的,不能通过下标访问元素。
3. unordered_set中的元素不允许重复,如果插入重复元素会被忽略。
下面是一个使用unordered_set的例子:
```c++
#include <iostream>
#include <unordered_set>
using namespace std;
int main()
{
unordered_set<int> mySet;
mySet.insert(1);
mySet.insert(2);
mySet.insert(3);
mySet.insert(2); //插入重复元素,会被忽略
cout << "mySet size: " << mySet.size() << endl; //输出元素个数
if (mySet.find(2) != mySet.end()) //查找元素2
cout << "2 is in mySet" << endl;
else
cout << "2 is not in mySet" << endl;
mySet.erase(3); //删除元素3
for (auto it = mySet.begin(); it != mySet.end(); ++it) //遍历元素
cout << *it << " ";
cout << endl;
mySet.clear(); //清空元素
cout << "mySet size: " << mySet.size() << endl; //输出元素个数
return ;
}
```
### 回答2:
unordered_set是STL提供的一种高效的哈希表容器,它能够快速地将数据插入到哈希表中,同时也能够快速地进行查找和删除操作。
使用unordered_set需要包含头文件<unordered_set>。和其他STL容器一样,定义一个unordered_set对象需要指定元素类型和哈希函数,例如:
unordered_set<int> my_set; //其中元素类型是int
unordered_set<string> my_set2; //其中元素类型是string
如果需要自定义哈希函数,可以使用unordered_set提供的hash模板函数,例如:
struct MyHashFunc {
size_t operator()(const my_struct& s) const {
return hash<string>()(s.key) ^ hash<int>()(s.value);
}
};
unordered_set<my_struct, MyHashFunc> my_set; //其中元素类型是my_struct,哈希函数是MyHashFunc
下面是unordered_set的常用操作:
1.插入元素
unordered_set提供了insert()函数,可以将元素插入到unordered_set中,例如:
unordered_set<int> my_set;
my_set.insert(1);
my_set.insert(2);
my_set.insert(3);
2.查找元素
unordered_set提供了find()函数,可以在unordered_set中查找元素,它返回一个迭代器,指向第一个等于查找值的元素,如果没有找到,则返回unordered_set::end()。例如:
auto it = my_set.find(2);
if (it == my_set.end()) {
cout << "2 not found in my_set" << endl;
}
3.删除元素
unordered_set提供了erase()函数,可以在unordered_set中删除元素,它可以接受元素值或迭代器作为参数。例如:
my_set.erase(2);
4.遍历元素
unordered_set可以使用迭代器来遍历所有的元素,例如:
for (auto it = my_set.begin(); it != my_set.end(); ++it) {
cout << *it << endl;
}
因为unordered_set是一个哈希表,所以元素的顺序是无序的。此外,unordered_set还提供了size()函数可以返回unordered_set中元素的个数,empty()函数可以判断unordered_set是否为空。
### 回答3:
unordered_set是C++ STL中的一种容器,它是一个哈希表,可以被用来存储唯一的元素。unordered_set内部元素的排列顺序并不是按照插入顺序,而是按照哈希值决定的。
unordered_set 的定义方式如下:
```c++
unordered_set<int> s; // 存储int类型的unordered_set
```
与其他的STL容器一样,unordered_set也提供了许多操作:插入元素、删除元素、查找元素等等。下面简要介绍unordered_set的基本用法:
1. 插入元素
插入元素可以用insert()函数实现:
```c++
unordered_set<int> s;
s.insert(1);
s.insert(2);
s.insert(3);
```
上述代码中,将元素1、2、3插入unordered_set中。
2. 删除元素
删除元素可以用erase()函数实现:
```c++
unordered_set<int> s{1, 2, 3};
s.erase(2);
```
上述代码中,从unordered_set中删除元素2。
3. 查找元素
查找元素可以用find()函数实现:
```c++
unordered_set<int> s{1, 2, 3};
if (s.find(2) != s.end()) {
cout << "found" << endl;
} else {
cout << "not found" << endl;
}
```
上述代码中,查找unordered_set中是否存在元素2,如果存在,输出“found”,否则输出“not found”。
unordered_set还有其他许多函数,比如size()函数获取unordered_set的元素数量,empty()函数判断unordered_set是否为空等等。此外,我们也可以自定义哈希函数和判断相等的函数,以便unordered_set能够正确处理自定义类型的元素。
阅读全文
相关推荐
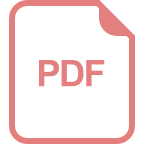
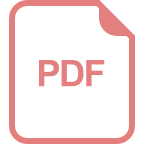













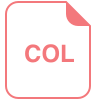
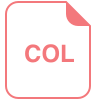
