用SpringBoot写服务器还需要考虑多线程问题吗
时间: 2024-06-10 19:10:09 浏览: 71
是的,使用SpringBoot编写服务器时,仍需要考虑多线程问题。尽管SpringBoot可以自动管理线程池,但在编写代码时,需要考虑到多线程环境下的并发问题,如线程安全和数据一致性等。同时,也需要注意避免线程阻塞和死锁等问题,以确保服务器的性能和稳定性。因此,在编写SpringBoot服务器时,需要特别关注多线程编程技巧和相关的并发编程模型。
相关问题
springboot 多线程下载
以下是一个使用Spring Boot实现多线程下载文件的示例代码:
```java
@RestController
public class DownloadController {
private static final int BUFFER_SIZE = 4096;
@GetMapping("/download")
public ResponseEntity<Resource> downloadFile(@RequestParam String fileUrl) throws IOException {
URL url = new URL(fileUrl);
HttpURLConnection httpConn = (HttpURLConnection) url.openConnection();
int responseCode = httpConn.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
String fileName = "";
String disposition = httpConn.getHeaderField("Content-Disposition");
String contentType = httpConn.getContentType();
int contentLength = httpConn.getContentLength();
if (disposition != null) {
int index = disposition.indexOf("filename=");
if (index > 0) {
fileName = disposition.substring(index + 10, disposition.length() - 1);
}
} else {
fileName = fileUrl.substring(fileUrl.lastIndexOf("/") + 1, fileUrl.length());
}
InputStream inputStream = httpConn.getInputStream();
ByteArrayOutputStream outputStream = new ByteArrayOutputStream();
byte[] buffer = new byte[BUFFER_SIZE];
int bytesRead = -1;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
byte[] bytes = outputStream.toByteArray();
HttpHeaders headers = new HttpHeaders();
headers.add(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=" + fileName);
headers.add(HttpHeaders.CONTENT_TYPE, contentType);
headers.add(HttpHeaders.CONTENT_LENGTH, String.valueOf(contentLength));
Resource resource = new ByteArrayResource(bytes);
return ResponseEntity.ok()
.headers(headers)
.contentLength(bytes.length)
.contentType(MediaType.parseMediaType(contentType))
.body(resource);
} else {
throw new RuntimeException("Failed to download file: " + fileUrl);
}
}
}
```
该示例代码使用了Spring Boot的@RestController注解,将下载文件的方法映射到了“/download”路径上。该方法接收一个名为“fileUrl”的请求参数,该参数指定了要下载的文件的URL地址。
在方法中,我们首先使用Java标准库中的HttpURLConnection类打开了一个HTTP连接,并获取了响应码。如果响应码为HTTP_OK(即200),则说明连接成功,我们可以开始下载文件。
接下来,我们从HTTP连接中获取了文件的一些元数据,例如文件名、文件类型和文件大小等。如果响应头中包含了Content-Disposition字段,则说明服务器希望我们将文件保存为指定的文件名;否则,我们将使用URL中的文件名作为文件名。
然后,我们从HTTP连接中获取了文件的输入流,并使用Java标准库中的ByteArrayOutputStream类将文件内容读入内存中。在读取文件内容时,我们使用了一个大小为4096字节的缓冲区,以提高读取效率。
最后,我们将文件内容封装为一个Spring框架中的Resource对象,并将该对象作为响应体返回给客户端。在响应头中,我们设置了Content-Disposition、Content-Type和Content-Length等字段,以便客户端正确地处理响应。
多线程下载一个ftp文件 Java springboot框架实现
在Java Spring Boot框架中,可以通过使用Apache Commons Net库来实现多线程下载FTP文件。以下是一个简单的示例代码:
```
import org.apache.commons.net.ftp.FTPClient;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.scheduling.annotation.Async;
import org.springframework.stereotype.Service;
import java.io.*;
@Service
public class FTPService {
@Value("${ftp.server}")
private String server;
@Value("${ftp.port}")
private int port;
@Value("${ftp.username}")
private String username;
@Value("${ftp.password}")
private String password;
@Async
public void downloadFile(String remoteFilePath, String localFilePath) throws IOException {
FTPClient ftpClient = new FTPClient();
try {
ftpClient.connect(server, port);
ftpClient.login(username, password);
ftpClient.enterLocalPassiveMode();
ftpClient.setFileType(FTPClient.BINARY_FILE_TYPE);
OutputStream outputStream = new BufferedOutputStream(new FileOutputStream(localFilePath));
InputStream inputStream = ftpClient.retrieveFileStream(remoteFilePath);
byte[] bytesArray = new byte[4096];
int bytesRead = -1;
while ((bytesRead = inputStream.read(bytesArray)) != -1) {
outputStream.write(bytesArray, 0, bytesRead);
}
boolean success = ftpClient.completePendingCommand();
if (success) {
System.out.println("File has been downloaded successfully.");
}
} catch (IOException ex) {
throw ex;
} finally {
if (ftpClient.isConnected()) {
ftpClient.logout();
ftpClient.disconnect();
}
}
}
}
```
在上面的代码中,我们使用了@Async注解将下载操作异步执行。这样可以避免在下载时阻塞主线程。
另外,我们还使用了Apache Commons Net库提供的FTPClient类来连接FTP服务器并下载文件。在下载时,我们使用了BufferedOutputStream和BufferedInputStream来提高读写效率。
需要注意的是,以上代码中只实现了单线程下载文件,如果要实现多线程下载,可以考虑使用Java线程池来创建多个下载任务。每个任务可以下载文件的一部分,最后将所有部分合并成完整的文件。
阅读全文
相关推荐
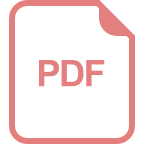
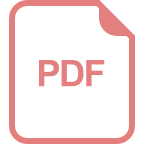
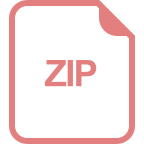
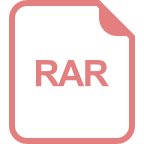
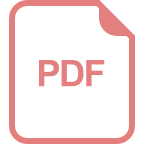
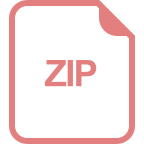

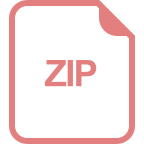
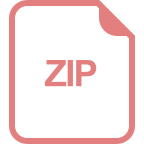
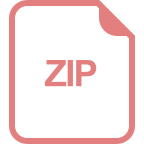
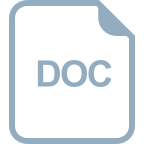
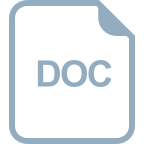
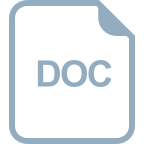
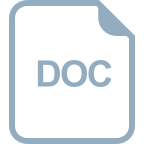
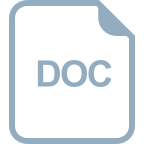
