编写一个用Visual Attention Network提取图像特征,然后作为Transformer in Transformer的输入的复合网络
时间: 2023-11-15 16:04:30 浏览: 47
Visual Attention Network(VAN)是一种利用注意力机制从图像中提取特征的方法。而Transformer in Transformer(TNT)是一种使用Transformer模型在特征层次上进行自我注意力的方法。本文将介绍如何将这两种方法结合起来,构建一个用VAN提取图像特征,然后作为TNT的输入的复合网络。
首先,我们需要定义VAN的结构。VAN的核心是注意力机制,它可以帮助网络在图像中关注重要的区域。具体来说,VAN包含以下层次:
1. 卷积层:用于提取图像的低级特征。
2. 自注意力层:用于将低级特征转换为高级特征,并强调图像中重要的区域。
3. 池化层:用于将高级特征压缩为固定大小的向量。
下面是一个简单的VAN实现:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class VAN(nn.Module):
def __init__(self, input_channels, hidden_channels, output_channels):
super(VAN, self).__init__()
self.conv = nn.Conv2d(input_channels, hidden_channels, kernel_size=3, padding=1)
self.self_attn = nn.MultiheadAttention(hidden_channels, num_heads=8)
self.pool = nn.AdaptiveAvgPool2d(output_size=(1, 1))
self.fc = nn.Linear(hidden_channels, output_channels)
def forward(self, x):
x = self.conv(x)
x = F.relu(x)
x = x.flatten(start_dim=2)
x = x.permute(2, 0, 1)
x, _ = self.self_attn(x, x, x)
x = x.permute(1, 2, 0)
x = self.pool(x)
x = x.squeeze()
x = self.fc(x)
return x
```
接下来,我们需要定义TNT的结构。TNT使用Transformer模型在特征层次上进行自我注意力。具体来说,TNT包含以下层次:
1. 嵌入层:用于将输入特征向量嵌入到Transformer的维度空间中。
2. Transformer in Transformer层:用于对嵌入向量进行自我注意力。
3. 池化层:用于将高级特征压缩为固定大小的向量。
下面是一个简单的TNT实现:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class TNT(nn.Module):
def __init__(self, input_channels, hidden_channels, output_channels, num_layers=2, num_heads=8):
super(TNT, self).__init__()
self.embed = nn.Linear(input_channels, hidden_channels)
self.transformer_in_transformer = nn.TransformerEncoderLayer(d_model=hidden_channels, nhead=num_heads)
self.pool = nn.AdaptiveAvgPool1d(output_size=1)
self.fc = nn.Linear(hidden_channels, output_channels)
def forward(self, x):
x = self.embed(x)
x = x.permute(1, 0, 2)
x = self.transformer_in_transformer(x)
x = x.permute(1, 2, 0)
x = self.pool(x)
x = x.squeeze()
x = self.fc(x)
return x
```
最后,我们将VAN和TNT组合在一起,形成一个复合网络。具体来说,我们将VAN用于从图像中提取特征,然后将这些特征作为TNT的输入。这样,TNT可以在特征层次上进行自我注意力,以进一步提取图像的高级特征。
```python
import torch
import torch.nn as nn
class VAN_TNT(nn.Module):
def __init__(self, van_input_channels, van_hidden_channels, van_output_channels, tnt_input_channels, tnt_hidden_channels, tnt_output_channels, num_layers=2, num_heads=8):
super(VAN_TNT, self).__init__()
self.van = VAN(van_input_channels, van_hidden_channels, van_output_channels)
self.tnt = TNT(tnt_input_channels, tnt_hidden_channels, tnt_output_channels, num_layers, num_heads)
def forward(self, x):
x = self.van(x)
x = self.tnt(x)
return x
```
这个复合网络可以用于从图像中提取特征,并将这些特征用于分类、检测或其他任务。
相关推荐
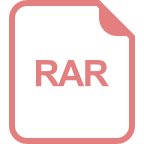
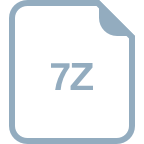










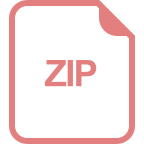
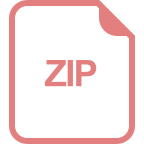
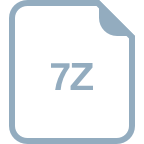
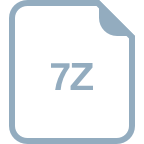
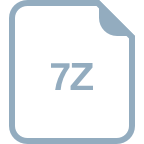