代码优化 private int calculateScore() { int singleChoiceScore = 0; int multiChoiceScore = 0; // 计算单选题得分 RadioGroup singleChoiceGroup = findViewById(R.id.rg_1); int selectedSingleChoiceId = singleChoiceGroup.getCheckedRadioButtonId(); if (selectedSingleChoiceId == R.id.rb_1) { singleChoiceScore = 50; } // 计算多选题得分 CheckBox ck1 = findViewById(R.id.ck_1); CheckBox ck2 = findViewById(R.id.ck_2); CheckBox ck3 = findViewById(R.id.ck_3); CheckBox ck4 = findViewById(R.id.ck_4); CheckBox ck5 = findViewById(R.id.ck_5); int checkedCount = 0; // 统计选中的选项数量 if (ck1.isChecked()) { checkedCount++; } if (ck2.isChecked()) { checkedCount++; } if (ck3.isChecked()) { checkedCount++; } if (ck4.isChecked()) { checkedCount++; } if (ck5.isChecked()) { checkedCount++; } if (checkedCount == 0) { multiChoiceScore = 0; } else if (checkedCount == 2) { multiChoiceScore = 20; } else if (checkedCount == 3) { multiChoiceScore = 30; } else if (checkedCount == 4) { multiChoiceScore = 50; } return singleChoiceScore + multiChoiceScore; } private String calculateGrade(int score) { if (score < 60) { return "不及格"; } else if (score < 70) { return "合格"; } else if (score < 90) { return "良好"; } else { return "优秀"; } }
时间: 2024-01-10 19:03:16 浏览: 27
可以对代码进行如下优化:
1. 将计算多选题得分的部分抽取出来,封装成一个方法,提高代码的可复用性和可读性。
2. 通过使用数组和循环来简化计算多选题得分的代码。
3. 将计算成绩等级的代码改为使用 switch/case 语句,提高代码的可读性。
优化后的代码如下:
```
private int calculateScore() {
int singleChoiceScore = 0;
int multiChoiceScore = calculateMultiChoiceScore();
// 计算单选题得分
RadioGroup singleChoiceGroup = findViewById(R.id.rg_1);
int selectedSingleChoiceId = singleChoiceGroup.getCheckedRadioButtonId();
if (selectedSingleChoiceId == R.id.rb_1) {
singleChoiceScore = 50;
}
return singleChoiceScore + multiChoiceScore;
}
private int calculateMultiChoiceScore() {
CheckBox[] checkBoxes = new CheckBox[] {
findViewById(R.id.ck_1),
findViewById(R.id.ck_2),
findViewById(R.id.ck_3),
findViewById(R.id.ck_4),
findViewById(R.id.ck_5)
};
int checkedCount = 0;
for (int i = 0; i < checkBoxes.length; i++) {
if (checkBoxes[i].isChecked()) {
checkedCount++;
}
}
switch (checkedCount) {
case 0:
return 0;
case 2:
return 20;
case 3:
return 30;
case 4:
return 50;
default:
return 0;
}
}
private String calculateGrade(int score) {
switch (score / 10) {
case 6:
return "合格";
case 7:
return "良好";
case 8:
case 9:
case 10:
return "优秀";
default:
return "不及格";
}
}
```
相关推荐
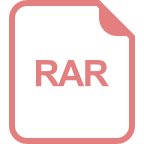














