题目:办公室日常管理信息系统 系统功能基本要求: 文件管理信息:包括文件编号、文件种类、文件名称、存放位置等; 考勤管理:包括姓名、年龄、职务、日期、出勤情况等;查询员工的出勤情况。会议记录:包括会议时间、参会人、记录员、会议内容等; 办公室日常事务管理,包括时间、事务、记录人。按条件查询,统计(用C++语言vs2022编译器)程序代码
时间: 2023-07-10 08:35:23 浏览: 128
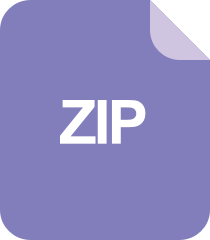
办公室日常管理信息系统

以下是一个简单的C++程序代码示例,实现了所述功能:
```cpp
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class File {
public:
int id;
string type;
string name;
string location;
};
class Employee {
public:
string name;
int age;
string position;
};
class Attendance {
public:
string name;
string date;
string status;
};
class MeetingRecord {
public:
string time;
vector<string> attendees;
string recorder;
string content;
};
class DailyAffair {
public:
string time;
string affair;
string recorder;
};
vector<File> files;
vector<Employee> employees;
vector<Attendance> attendances;
vector<MeetingRecord> meetingRecords;
vector<DailyAffair> dailyAffairs;
void addFile() {
File file;
cout << "Enter file id: ";
cin >> file.id;
cout << "Enter file type: ";
cin >> file.type;
cout << "Enter file name: ";
cin >> file.name;
cout << "Enter file location: ";
cin >> file.location;
files.push_back(file);
}
void addEmployee() {
Employee employee;
cout << "Enter employee name: ";
cin >> employee.name;
cout << "Enter employee age: ";
cin >> employee.age;
cout << "Enter employee position: ";
cin >> employee.position;
employees.push_back(employee);
}
void addAttendance() {
Attendance attendance;
cout << "Enter employee name: ";
cin >> attendance.name;
cout << "Enter date (YYYY-MM-DD): ";
cin >> attendance.date;
cout << "Enter attendance status (present/absent): ";
cin >> attendance.status;
attendances.push_back(attendance);
}
void addMeetingRecord() {
MeetingRecord meetingRecord;
cout << "Enter meeting time: ";
cin >> meetingRecord.time;
cout << "Enter attendees (separated by space): ";
string attendee;
getline(cin, attendee);
while (attendee != "") {
meetingRecord.attendees.push_back(attendee);
getline(cin, attendee);
}
cout << "Enter recorder: ";
cin >> meetingRecord.recorder;
cout << "Enter meeting content: ";
getline(cin, meetingRecord.content);
meetingRecords.push_back(meetingRecord);
}
void addDailyAffair() {
DailyAffair dailyAffair;
cout << "Enter time: ";
cin >> dailyAffair.time;
cout << "Enter affair: ";
getline(cin, dailyAffair.affair);
cout << "Enter recorder: ";
cin >> dailyAffair.recorder;
dailyAffairs.push_back(dailyAffair);
}
void queryAttendance() {
string name;
cout << "Enter employee name: ";
cin >> name;
for (const auto& attendance : attendances) {
if (attendance.name == name) {
cout << attendance.date << " " << attendance.status << endl;
}
}
}
int main() {
while (true) {
cout << "1. Add file" << endl;
cout << "2. Add employee" << endl;
cout << "3. Add attendance" << endl;
cout << "4. Add meeting record" << endl;
cout << "5. Add daily affair" << endl;
cout << "6. Query attendance" << endl;
cout << "7. Exit" << endl;
cout << "Enter your choice: ";
int choice;
cin >> choice;
switch (choice) {
case 1:
addFile();
break;
case 2:
addEmployee();
break;
case 3:
addAttendance();
break;
case 4:
addMeetingRecord();
break;
case 5:
addDailyAffair();
break;
case 6:
queryAttendance();
break;
case 7:
return 0;
default:
cout << "Invalid choice!" << endl;
}
}
}
```
该程序通过菜单提供了添加文件、添加员工、添加考勤记录、添加会议记录、添加日常事务等功能,同时还提供了查询某个员工的出勤情况的功能。具体实现过程中,采用了面向对象的编程思想,并使用了向量(vector)等STL容器进行数据存储和操作。
阅读全文
相关推荐


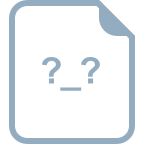
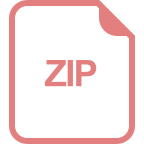
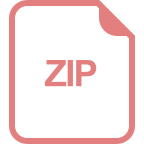
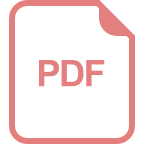
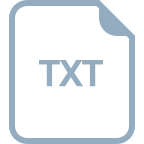