java使用自定义注解实现校验实体类中的参数是否为空
时间: 2024-05-10 18:13:53 浏览: 8
好的,这是一个技术问题,我可以为您提供答案。
在 Java 中实现参数校验可以使用自定义注解来解决。您需要先定义一个注解类,例如通过 @NotNull 注解来表示参数不能为空。
然后在实体类中对需要进行校验的参数添加该注解,在业务逻辑中处理时就可以通过反射获取注解并进行校验。
以下是一个简单的示例代码:
```
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.FIELD)
public @interface NotNull {
String message() default "参数不能为空";
}
public class User {
@NotNull
private String username;
@NotNull
private String password;
// 省略其他属性及方法
}
public class UserValidator {
public static boolean validate(User user) {
Field[] fields = user.getClass().getDeclaredFields();
for (Field field : fields) {
if (field.isAnnotationPresent(NotNull.class)) {
field.setAccessible(true);
try {
Object value = field.get(user);
if (value == null || "".equals(value)) {
System.out.println("校验未通过:" + field.getAnnotation(NotNull.class).message());
return false;
}
} catch (Exception e) {
e.printStackTrace();
return false;
}
}
}
System.out.println("校验通过");
return true;
}
}
// 测试
public static void main(String[] args) {
User user = new User();
user.setUsername(null);
user.setPassword("password");
UserValidator.validate(user);
}
```
输出结果为:
```
校验未通过:参数不能为空
```
这样可以方便地对实体类中的参数进行校验。
相关推荐
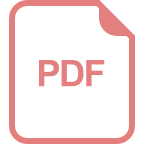
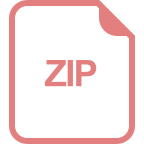
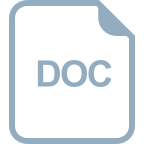














