在Androidstudio中实现蓝牙扫描
时间: 2024-05-07 13:17:55 浏览: 165
好的,下面是在Android Studio中实现蓝牙扫描的步骤:
1. 在AndroidManifest.xml文件中添加蓝牙权限:
```
<uses-permission android:name="android.permission.BLUETOOTH"/>
<uses-permission android:name="android.permission.BLUETOOTH_ADMIN"/>
```
2. 在布局文件中添加一个按钮用于扫描:
```
<Button
android:id="@+id/scanButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Scan"/>
```
3. 在Activity的onCreate方法中获取蓝牙适配器,并设置扫描按钮的点击事件:
```
BluetoothAdapter bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
Button scanButton = findViewById(R.id.scanButton);
scanButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (bluetoothAdapter.isEnabled()) {
bluetoothAdapter.startDiscovery();
} else {
Toast.makeText(MainActivity.this, "Bluetooth is not enabled", Toast.LENGTH_SHORT).show();
}
}
});
```
4. 在Activity中注册广播接收器,用于接收蓝牙设备的扫描结果:
```
private final BroadcastReceiver broadcastReceiver = new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
if (BluetoothDevice.ACTION_FOUND.equals(action)) {
BluetoothDevice device = intent.getParcelableExtra(BluetoothDevice.EXTRA_DEVICE);
Log.d("Bluetooth", "Found device: " + device.getName() + " - " + device.getAddress());
}
}
};
@Override
protected void onResume() {
super.onResume();
IntentFilter filter = new IntentFilter(BluetoothDevice.ACTION_FOUND);
registerReceiver(broadcastReceiver, filter);
}
@Override
protected void onPause() {
super.onPause();
unregisterReceiver(broadcastReceiver);
}
```
以上就是在Android Studio中实现蓝牙扫描的基本步骤,你可以根据自己的需求对代码进行进一步的完善。
阅读全文
相关推荐
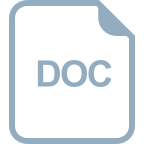
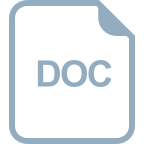
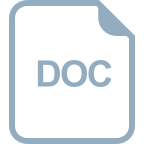
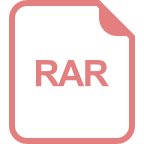
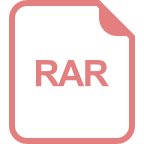
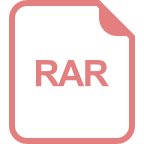
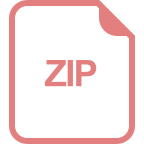
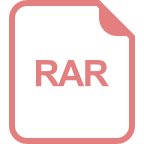
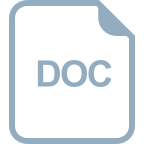
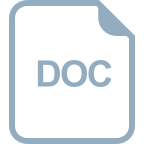








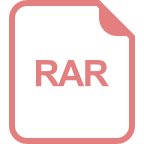