进程的管道通信 编制一段程序,实现进程的管道通信。 使用系统调用pipe()建立一条管道线;两个子进程P1和P2分别向管道中写一句话: Child 1 is sending a message! Child 2 is sending a message! 而父进程则从管道中读出来自于两个子进程的信息,显示在屏幕上。 要求父进程先接收子进程P1发来的消息,然后再接收子进程P2发来的消息。
时间: 2023-07-14 18:13:25 浏览: 75
下面是实现进程的管道通信的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#define MSG_SIZE 100
int main() {
int fd[2];
pid_t pid1, pid2;
char msg1[MSG_SIZE] = "Child 1 is sending a message!";
char msg2[MSG_SIZE] = "Child 2 is sending a message!";
char buf[MSG_SIZE];
if (pipe(fd) < 0) {
perror("pipe error");
exit(EXIT_FAILURE);
}
pid1 = fork();
if (pid1 < 0) {
perror("fork error");
exit(EXIT_FAILURE);
} else if (pid1 == 0) { // 子进程1
close(fd[0]); // 关闭读端
if (write(fd[1], msg1, strlen(msg1)) < 0) {
perror("write error");
exit(EXIT_FAILURE);
}
close(fd[1]); // 关闭写端
exit(EXIT_SUCCESS);
} else { // 父进程
waitpid(pid1, NULL, 0); // 等待子进程1结束
pid2 = fork();
if (pid2 < 0) {
perror("fork error");
exit(EXIT_FAILURE);
} else if (pid2 == 0) { // 子进程2
close(fd[0]); // 关闭读端
if (write(fd[1], msg2, strlen(msg2)) < 0) {
perror("write error");
exit(EXIT_FAILURE);
}
close(fd[1]); // 关闭写端
exit(EXIT_SUCCESS);
} else { // 父进程
close(fd[1]); // 关闭写端
// 从子进程1读取消息
if (read(fd[0], buf, MSG_SIZE) < 0) {
perror("read error");
exit(EXIT_FAILURE);
}
printf("%s\n", buf);
// 从子进程2读取消息
if (read(fd[0], buf, MSG_SIZE) < 0) {
perror("read error");
exit(EXIT_FAILURE);
}
printf("%s\n", buf);
close(fd[0]); // 关闭读端
waitpid(pid2, NULL, 0); // 等待子进程2结束
}
}
return 0;
}
```
程序首先使用系统调用`pipe()`建立一个管道,然后创建两个子进程P1和P2。P1向管道中写入一句话,并结束。父进程接收到P1的结束信号后,再创建P2,P2向管道中写入一句话,并结束。最后父进程从管道中分别读取来自于P1和P2的消息,并输出到屏幕上。注意父进程需要先接收来自于P1的消息,然后再接收来自于P2的消息。
相关推荐
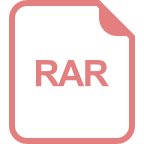
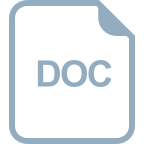
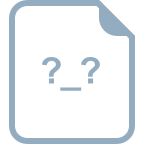














