在InvertColor.cpp补充代码 对图像进行反色处理
时间: 2024-10-08 13:05:06 浏览: 77
在C++中,对图像进行反色处理通常涉及读取图像数据、遍历像素并反转其颜色值,然后将结果保存回新的图像文件。下面是一个简单的`InvertColor.cpp`代码片段示例,这里假设我们使用了OpenCV库来处理图像:
```cpp
#include <opencv2/opencv.hpp>
// 函数定义,接受图片路径作为输入,返回反色后的图片
cv::Mat invertColor(const std::string& input_image_path) {
// 1. 读取原始图像
cv::Mat original_image = cv::imread(input_image_path, cv::IMREAD_COLOR);
if (original_image.empty()) {
std::cerr << "Error: Could not read image." << std::endl;
return original_image; // 返回空矩阵表示错误
}
// 2. 获取原图的深度和通道数
int depth = original_image.depth();
int channels = original_image.channels();
// 3. 创建一个同大小的新矩阵用于存储反色后的图像
cv::Mat inverted_image(original_image.size(), CV_MAKETYPE(depth, channels));
// 4. 遍历像素,逐像素反转颜色
for (int y = 0; y < original_image.rows; ++y) {
for (int x = 0; x < original_image.cols; ++x) {
cv::Vec3b pixel = original_image.at<cv::Vec3b>(y, x); // 读取像素值
pixel[0] = 255 - pixel[0]; // 反转B通道(蓝色)
pixel[1] = 255 - pixel[1]; // 反转G通道(绿色)
pixel[2] = 255 - pixel[2]; // 反转R通道(红色)
inverted_image.at<cv::Vec3b>(y, x) = pixel; // 写回新像素值
}
}
// 5. 返回反色后的图像
return inverted_image;
}
// 主函数可以这样调用上述函数
int main() {
std::string input_path = "path_to_your_input_image.jpg";
cv::Mat result = invertColor(input_path);
// 保存反色后的图像
imwrite("inverted_" + input_path, result);
return 0;
}
阅读全文
相关推荐



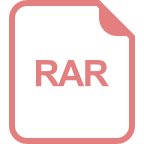
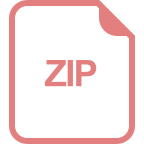
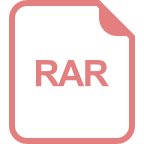




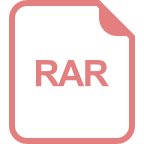





