使用C语言写了一个删除C:\test目录十分钟之前的jpg图片的代码
时间: 2024-02-11 09:07:28 浏览: 163
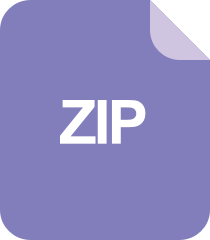
一个删除整个目录的C程序

以下是使用C语言实现删除C:\test目录十分钟之前的jpg图片的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdbool.h>
#include <time.h>
#include <dirent.h>
#include <sys/stat.h>
#include <unistd.h>
#define DIR_PATH "C:/test" // 需要删除文件的目录路径
#define DEL_TIME 600 // 删除时间(秒)
bool is_jpg(char *filename) {
char *ext = strrchr(filename, '.');
if (ext != NULL && strcmp(ext, ".jpg") == 0) {
return true;
}
return false;
}
bool is_within_time(time_t mtime, int seconds) {
time_t now = time(NULL);
time_t diff = now - mtime;
if (diff > seconds) {
return false;
}
return true;
}
void delete_file(char *filepath) {
remove(filepath);
printf("Deleted %s\n", filepath);
}
void delete_old_jpg(char *dirpath, int seconds) {
DIR *dir = opendir(dirpath);
if (dir == NULL) {
fprintf(stderr, "Error opening directory %s\n", dirpath);
return;
}
struct dirent *entry;
while ((entry = readdir(dir)) != NULL) {
char filepath[512];
sprintf(filepath, "%s/%s", dirpath, entry->d_name);
struct stat sbuf;
if (stat(filepath, &sbuf) != -1) {
if (S_ISREG(sbuf.st_mode) && is_jpg(entry->d_name) && is_within_time(sbuf.st_mtime, seconds)) {
delete_file(filepath);
}
}
}
closedir(dir);
}
int main() {
delete_old_jpg(DIR_PATH, DEL_TIME);
return 0;
}
```
该代码通过 opendir、readdir、stat 和 remove 函数实现了遍历目录、获取文件信息以及删除文件的功能。主要步骤如下:
1. 定义了两个辅助函数 is_jpg 和 is_within_time,用于判断文件是否为 jpg 格式和是否在指定时间之内。
2. 定义了 delete_file 函数,用于删除指定文件。
3. 定义了 delete_old_jpg 函数,用于遍历目录,获取文件信息,判断是否需要删除,并调用 delete_file 函数删除文件。
4. 在 main 函数中调用 delete_old_jpg 函数,删除指定目录中十分钟之前的 jpg 图片。
值得注意的是,该代码仅供参考,实际使用中需要根据具体情况进行修改和测试。特别是删除文件操作有一定风险,需要谨慎处理。
阅读全文
相关推荐
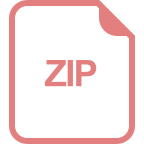
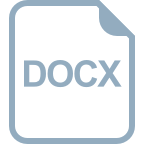
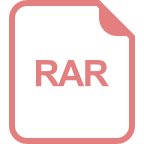
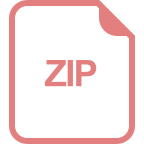
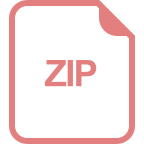
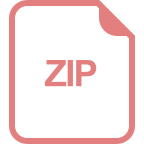
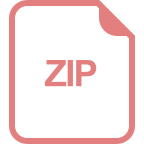
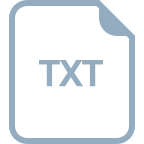
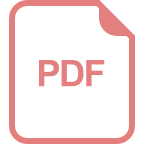
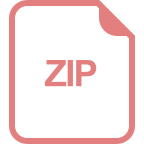
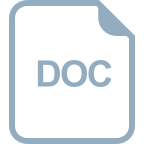
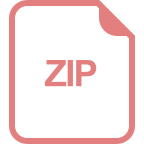
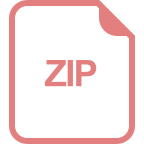