帮我实现一个transformer的结构
时间: 2023-05-22 15:00:40 浏览: 89
好的,以下是一个简单的Transformer结构的实现代码:
```
import torch
import torch.nn as nn
import torch.nn.functional as F
class Transformer(nn.Module):
def __init__(self, input_dim, hidden_dim, num_layers, num_heads):
super(Transformer, self).__init__()
self.input_dim = input_dim
self.hidden_dim = hidden_dim
self.num_layers = num_layers
self.num_heads = num_heads
self.embedding = nn.Embedding(input_dim, hidden_dim)
self.pos_encoding = PositionalEncoding(hidden_dim)
self.layers = nn.ModuleList([TransformerLayer(hidden_dim, num_heads) for _ in range(num_layers)])
self.fc = nn.Linear(hidden_dim, input_dim)
def forward(self, x):
x = self.embedding(x)
x = self.pos_encoding(x)
for layer in self.layers:
x = layer(x)
x = self.fc(x)
return x
class TransformerLayer(nn.Module):
def __init__(self, hidden_dim, num_heads):
super(TransformerLayer, self).__init__()
self.hidden_dim = hidden_dim
self.num_heads = num_heads
self.self_attn = MultiHeadAttention(hidden_dim, num_heads)
self.norm1 = nn.LayerNorm(hidden_dim)
self.feed_forward = FeedForward(hidden_dim)
self.norm2 = nn.LayerNorm(hidden_dim)
def forward(self, x):
residual = x
x = self.self_attn(x)
x = self.norm1(x + residual)
residual = x
x = self.feed_forward(x)
x = self.norm2(x + residual)
return x
class MultiHeadAttention(nn.Module):
def __init__(self, hidden_dim, num_heads):
super(MultiHeadAttention, self).__init__()
self.hidden_dim = hidden_dim
self.num_heads = num_heads
self.q_linear = nn.Linear(hidden_dim, hidden_dim)
self.k_linear = nn.Linear(hidden_dim, hidden_dim)
self.v_linear = nn.Linear(hidden_dim, hidden_dim)
self.fc = nn.Linear(hidden_dim, hidden_dim)
def forward(self, x):
batch_size, seq_len, hidden_dim = x.size()
q = self.q_linear(x).view(batch_size, seq_len, self.num_heads, hidden_dim // self.num_heads).transpose(1, 2)
k = self.k_linear(x).view(batch_size, seq_len, self.num_heads, hidden_dim // self.num_heads).transpose(1, 2)
v = self.v_linear(x).view(batch_size, seq_len, self.num_heads, hidden_dim // self.num_heads).transpose(1, 2)
attn_scores = torch.matmul(q, k.transpose(-2, -1)) / (hidden_dim // self.num_heads) ** 0.5
attn_probs = F.softmax(attn_scores, dim=-1)
attn_output = torch.matmul(attn_probs, v)
attn_output = attn_output.transpose(1, 2).contiguous().view(batch_size, seq_len, hidden_dim)
attn_output = self.fc(attn_output)
return attn_output
class FeedForward(nn.Module):
def __init__(self, hidden_dim):
super(FeedForward, self).__init__()
self.hidden_dim = hidden_dim
self.fc1 = nn.Linear(hidden_dim, hidden_dim * 4)
self.fc2 = nn.Linear(hidden_dim * 4, hidden_dim)
def forward(self, x):
x = F.relu(self.fc1(x))
x = self.fc2(x)
return x
class PositionalEncoding(nn.Module):
def __init__(self, hidden_dim, max_len=5000):
super(PositionalEncoding, self).__init__()
self.hidden_dim = hidden_dim
pe = torch.zeros(max_len, hidden_dim)
position = torch.arange(0, max_len, dtype=torch.float).unsqueeze(1)
div_term = torch.exp(torch.arange(0, hidden_dim, 2).float() * (-math.log(10000.0) / hidden_dim))
pe[:, 0::2] = torch.sin(position * div_term)
pe[:, 1::2] = torch.cos(position * div_term)
pe = pe.unsqueeze(0).transpose(0, 1)
self.register_buffer('pe', pe)
def forward(self, x):
x = x + self.pe[:x.size(0), :]
return x
```
这个Transformer结构包括了多头自注意力机制、前馈神经网络和位置编码等模块,可以用于序列到序列的任务,比如机器翻译、文本生成等。
阅读全文
相关推荐
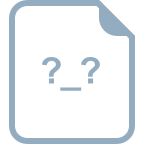
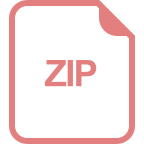
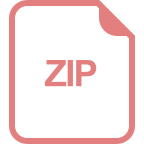
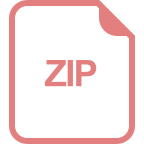
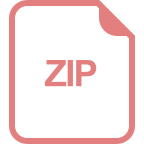
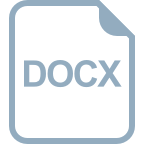
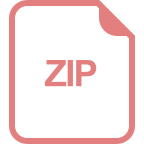
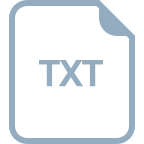
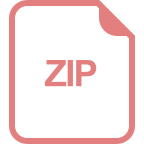
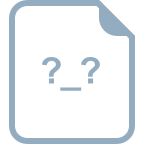
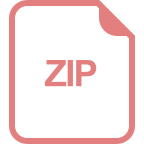
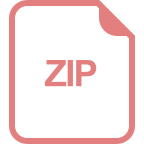
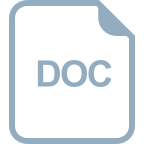





