用php+mysql写一个留言板,要求提交留言的页面有留言编号,留言标题,留言内容,留言人,留言人email,留言人ip,留言时间,留言人性别。留言板还能进行更新和删除操作。
时间: 2024-05-10 20:17:56 浏览: 165
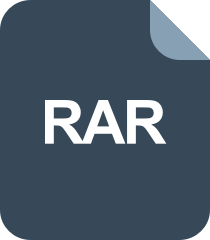
php+mysql.rar_PHP + mysql_php 留言板_php留言板_site:www.pudn.com_留
好的,以下是一个简单的留言板的实现代码,使用了php和mysql:
1.创建数据库表
首先需要在mysql中创建一个名为“message_board”的数据库,并在该数据库中创建一个名为“messages”的表,表结构如下:
```
CREATE TABLE `messages` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`title` varchar(255) NOT NULL,
`content` text NOT NULL,
`name` varchar(50) NOT NULL,
`email` varchar(100) NOT NULL,
`ip` varchar(20) NOT NULL,
`gender` varchar(10) NOT NULL,
`create_time` datetime NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
```
2.提交留言页面
在提交留言的页面(例如message.php)中,需要包含以下代码:
```
<!DOCTYPE html>
<html>
<head>
<title>留言板</title>
</head>
<body>
<h1>留言板</h1>
<form method="POST" action="submit.php">
<label for="title">标题:</label>
<input type="text" name="title" id="title" required><br><br>
<label for="content">内容:</label><br>
<textarea name="content" id="content" rows="10" cols="50" required></textarea><br><br>
<label for="name">姓名:</label>
<input type="text" name="name" id="name" required><br><br>
<label for="email">邮箱:</label>
<input type="email" name="email" id="email" required><br><br>
<label for="gender">性别:</label>
<select name="gender" id="gender" required>
<option value="male">男</option>
<option value="female">女</option>
</select><br><br>
<input type="submit" value="提交">
</form>
</body>
</html>
```
该页面包含了一个表单,用户可以在表单中填写留言的相关信息并提交。
3.提交留言处理
在提交留言的处理页面(例如submit.php)中,需要包含以下代码:
```
<?php
//连接数据库
$conn = mysqli_connect("localhost", "username", "password", "message_board");
if (!$conn) {
die("连接失败: " . mysqli_connect_error());
}
//获取表单数据
$title = mysqli_real_escape_string($conn, $_POST['title']);
$content = mysqli_real_escape_string($conn, $_POST['content']);
$name = mysqli_real_escape_string($conn, $_POST['name']);
$email = mysqli_real_escape_string($conn, $_POST['email']);
$ip = $_SERVER['REMOTE_ADDR'];
$gender = mysqli_real_escape_string($conn, $_POST['gender']);
$create_time = date('Y-m-d H:i:s');
//插入留言数据
$sql = "INSERT INTO messages (title, content, name, email, ip, gender, create_time) VALUES ('$title', '$content', '$name', '$email', '$ip', '$gender', '$create_time')";
if (mysqli_query($conn, $sql)) {
echo "留言已提交";
} else {
echo "提交留言时发生错误: " . mysqli_error($conn);
}
//关闭数据库连接
mysqli_close($conn);
?>
```
该页面首先连接数据库,然后获取表单中提交的留言信息,使用mysqli_real_escape_string()函数来转义用户输入的数据,以避免SQL注入攻击。接着将留言信息插入到mysql数据库中。
4.留言列表显示
在留言板首页(例如index.php)中,需要包含以下代码:
```
<!DOCTYPE html>
<html>
<head>
<title>留言板</title>
</head>
<body>
<h1>留言板</h1>
<a href="message.php">提交留言</a><br><br>
<table border="1">
<tr>
<th>编号</th>
<th>标题</th>
<th>内容</th>
<th>姓名</th>
<th>邮箱</th>
<th>IP地址</th>
<th>性别</th>
<th>留言时间</th>
<th>操作</th>
</tr>
<?php
//连接数据库
$conn = mysqli_connect("localhost", "username", "password", "message_board");
if (!$conn) {
die("连接失败: " . mysqli_connect_error());
}
//查询留言数据
$sql = "SELECT * FROM messages ORDER BY create_time DESC";
$result = mysqli_query($conn, $sql);
//输出留言列表
if (mysqli_num_rows($result) > 0) {
while ($row = mysqli_fetch_assoc($result)) {
echo "<tr>";
echo "<td>" . $row['id'] . "</td>";
echo "<td>" . $row['title'] . "</td>";
echo "<td>" . $row['content'] . "</td>";
echo "<td>" . $row['name'] . "</td>";
echo "<td>" . $row['email'] . "</td>";
echo "<td>" . $row['ip'] . "</td>";
echo "<td>" . $row['gender'] . "</td>";
echo "<td>" . $row['create_time'] . "</td>";
echo "<td><a href='edit.php?id=" . $row['id'] . "'>编辑</a> | <a href='delete.php?id=" . $row['id'] . "'>删除</a></td>";
echo "</tr>";
}
} else {
echo "<tr><td colspan='9'>暂无留言</td></tr>";
}
//关闭数据库连接
mysqli_close($conn);
?>
</table>
</body>
</html>
```
该页面首先包含一个提交留言的链接,点击该链接可以跳转到message.php页面。然后查询数据库中的留言数据,并将其输出到一个HTML表格中,同时为每条留言提供编辑和删除操作的链接。
5.编辑留言
在编辑留言的页面(例如edit.php)中,需要包含以下代码:
```
<!DOCTYPE html>
<html>
<head>
<title>编辑留言</title>
</head>
<body>
<h1>编辑留言</h1>
<form method="POST" action="update.php">
<input type="hidden" name="id" value="<?php echo $_GET['id']; ?>">
<label for="title">标题:</label>
<input type="text" name="title" id="title" value="<?php echo $row['title']; ?>" required><br><br>
<label for="content">内容:</label><br>
<textarea name="content" id="content" rows="10" cols="50" required><?php echo $row['content']; ?></textarea><br><br>
<label for="name">姓名:</label>
<input type="text" name="name" id="name" value="<?php echo $row['name']; ?>" required><br><br>
<label for="email">邮箱:</label>
<input type="email" name="email" id="email" value="<?php echo $row['email']; ?>" required><br><br>
<label for="gender">性别:</label>
<select name="gender" id="gender" required>
<option value="male" <?php if ($row['gender'] == 'male') { echo "selected"; } ?>>男</option>
<option value="female" <?php if ($row['gender'] == 'female') { echo "selected"; } ?>>女</option>
</select><br><br>
<input type="submit" value="更新">
</form>
</body>
</html>
```
该页面包含一个表单,用户可以在表单中修改留言的相关信息并提交。同时,需要根据传递的留言编号(例如edit.php?id=1)从数据库中获取该留言的原始数据,并将其显示在表单中。
6.更新留言处理
在更新留言的处理页面(例如update.php)中,需要包含以下代码:
```
<?php
//连接数据库
$conn = mysqli_connect("localhost", "username", "password", "message_board");
if (!$conn) {
die("连接失败: " . mysqli_connect_error());
}
//获取表单数据
$id = mysqli_real_escape_string($conn, $_POST['id']);
$title = mysqli_real_escape_string($conn, $_POST['title']);
$content = mysqli_real_escape_string($conn, $_POST['content']);
$name = mysqli_real_escape_string($conn, $_POST['name']);
$email = mysqli_real_escape_string($conn, $_POST['email']);
$gender = mysqli_real_escape_string($conn, $_POST['gender']);
//更新留言数据
$sql = "UPDATE messages SET title='$title', content='$content', name='$name', email='$email', gender='$gender' WHERE id='$id'";
if (mysqli_query($conn, $sql)) {
echo "留言已更新";
} else {
echo "更新留言时发生错误: " . mysqli_error($conn);
}
//关闭数据库连接
mysqli_close($conn);
?>
```
该页面首先连接数据库,然后获取表单中提交的留言信息,使用mysqli_real_escape_string()函数来转义用户输入的数据,以避免SQL注入攻击。接着将留言信息更新到mysql数据库中。
7.删除留言处理
在删除留言的处理页面(例如delete.php)中,需要包含以下代码:
```
<?php
//连接数据库
$conn = mysqli_connect("localhost", "username", "password", "message_board");
if (!$conn) {
die("连接失败: " . mysqli_connect_error());
}
//获取留言编号
$id = mysqli_real_escape_string($conn, $_GET['id']);
//删除留言数据
$sql = "DELETE FROM messages WHERE id='$id'";
if (mysqli_query($conn, $sql)) {
echo "留言已删除";
} else {
echo "删除留言时发生错误: " . mysqli_error($conn);
}
//关闭数据库连接
mysqli_close($conn);
?>
```
该页面首先连接数据库,然后获取传递的留言编号(例如delete.php?id=1),将该留言从mysql数据库中删除。
以上就是一个简单的留言板的实现代码,你可以根据自己的需求进行修改和优化。
阅读全文
相关推荐
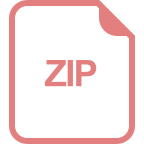
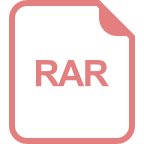
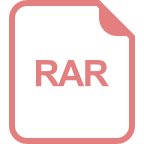
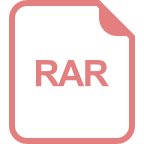
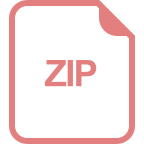
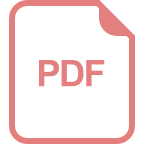
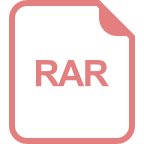
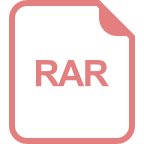
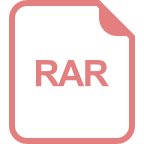
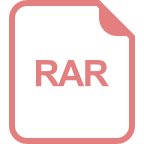
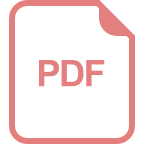