SSm架构 查找mysql数据库的代码
时间: 2024-05-05 09:22:15 浏览: 113
以下是在SSM架构下查找MySQL数据库的Java代码示例:
1. 首先需要在Spring配置文件中配置数据源和MyBatis的SqlSessionFactory:
```xml
<!-- 配置数据源 -->
<bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource">
<property name="driverClassName" value="${jdbc.driverClassName}"/>
<property name="url" value="${jdbc.url}"/>
<property name="username" value="${jdbc.username}"/>
<property name="password" value="${jdbc.password}"/>
</bean>
<!-- 配置MyBatis的SqlSessionFactory -->
<bean id="sqlSessionFactory" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource"/>
<property name="configLocation" value="classpath:mybatis-config.xml"/>
</bean>
```
2. 在MyBatis配置文件中配置Mapper映射文件:
```xml
<!-- 配置Mapper映射文件 -->
<mappers>
<mapper resource="com/example/mapper/UserMapper.xml"/>
</mappers>
```
3. 编写Mapper接口和Mapper映射文件:
UserMapper.java
```java
public interface UserMapper {
User selectUserById(int id);
}
```
UserMapper.xml
```xml
<!-- 配置Mapper映射文件 -->
<mapper namespace="com.example.mapper.UserMapper">
<select id="selectUserById" parameterType="int" resultType="com.example.pojo.User">
SELECT * FROM user WHERE id=#{id}
</select>
</mapper>
```
4. 在Service层中调用Mapper接口中的方法:
```java
@Service
public class UserServiceImpl implements UserService {
@Autowired
private UserMapper userMapper;
@Override
public User selectUserById(int id) {
return userMapper.selectUserById(id);
}
}
```
5. 在Controller层中调用Service层中的方法:
```java
@RestController
@RequestMapping("/user")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/{id}")
public User getUserById(@PathVariable("id") int id) {
return userService.selectUserById(id);
}
}
```
以上代码示例中,使用了Spring、MyBatis和MySQL数据库。其中,数据源的配置使用了Apache Commons DBCP连接池,Mapper映射文件使用了XML配置。通过以上步骤,我们可以在SSM架构下轻松地实现MySQL数据库的查询操作。
阅读全文
相关推荐
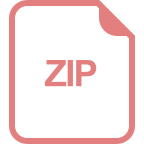
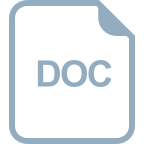
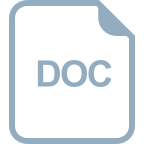
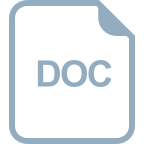
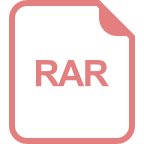
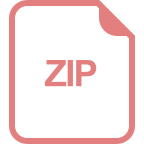
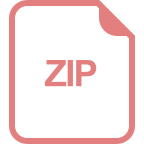
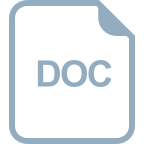
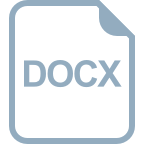
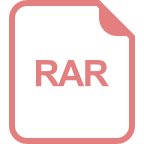
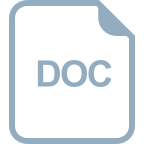
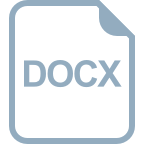
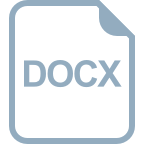
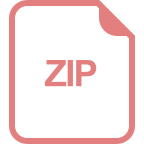
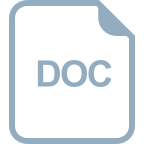
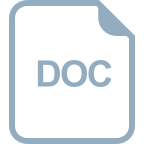